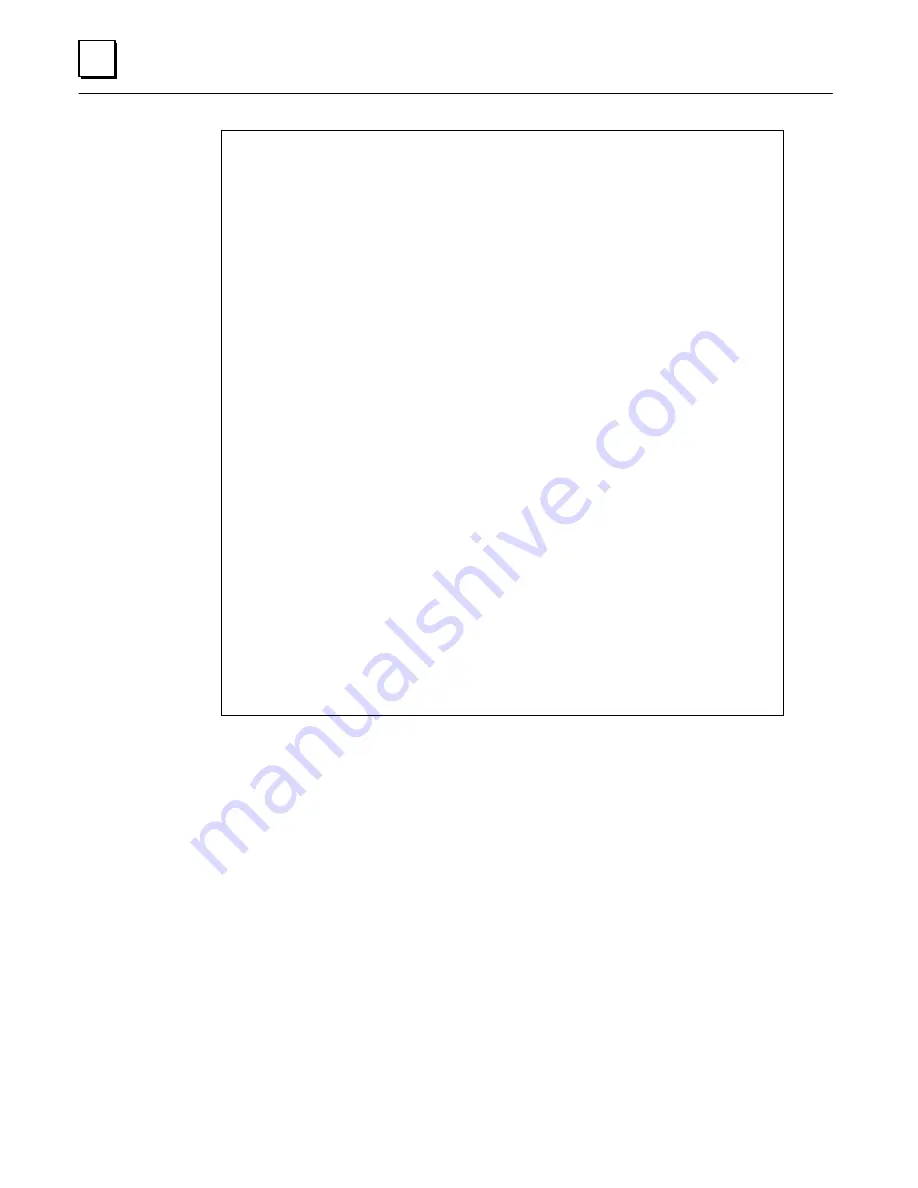
A
A-4
VME DLAN/DLAN+ Interface Module User’s Manual - August 1995
GFK-1044A
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
ÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁÁ
/*
* The implementation shown below assumes a GE
Series 90
–
70 PCM.
*/
int vme_write( unsigned short vme_hi,
unsigned short vme_lo,
void far* src,
int len,
unsigned char am )
{
int i;
unsigned short far* p;
unsigned short far* q = src;
FP_SEG( p ) = VME_WIN_SEG;
FP_OFF( p ) = vme_lo;
Set_vme_ctl( vme_hi, am );
for (i = len/2; i > 0;
––
i) { /* copy the data word by word */
*(p++) = *(q++);
}
if (len % 2) { /* len is odd; copy the last byte */
*((unsigned char far*)p) = *((unsigned char far*)q);
}
return( NO_ERROR );
}
/*
* The implementation shown below assumes a GE
Series 90
–
70 PCM.
*/
int vme_read( void far* dest,
unsigned short vme_hi,
unsigned short vme_lo,
int len,
unsigned char am )
{
int i;
unsigned short far* p;
unsigned short far* q = dest;
FP_SEG( p ) = VME_WIN_SEG;
FP_OFF( p ) = vme_lo;
Set_vme_ctl( vme_hi, am );
for (i = len/2; i > 0;
––
i) { /* copy the data word by word */
*(q++) = *(p++);
}
if (len % 2) { /* len is odd; copy the last byte */
*((unsigned char far*)q) = *((unsigned char far*)p);
}
return( NO_ERROR );
}
The implementation of
vme_setup
is intended to be as portable as possible. First, the
rack
and
slot
parameter are checked for out-of-bounds values. The minimum and maximum
acceptable values are defined in DLANINIT.H.
Next,
vme_setup
calculates the standard access mode starting address and short
non-privileged address modifier code assigned to the rack/slot location of the target module,
based on the Series 90-70 conventions. These conventions are detailed in the Series 90-70
Programmable Controller User’s Guide to Integration of 3rd Party VME Modules, GFK-0488.
The rules for calculating start address and address modifier values appear immediately ahead of
the code that initializes the
setup_data
array. Note that the least significant 16 bits of every
module’s standard mode starting address must be zero. Only the byte containing bits 16
through 23 is calculated.
After
setup_data
is initialized, the last byte of the setup data area in the module’s VME
dual-ported memory is read using short non-privileged mode. The value in this byte must be
zero before the module may be initialized.