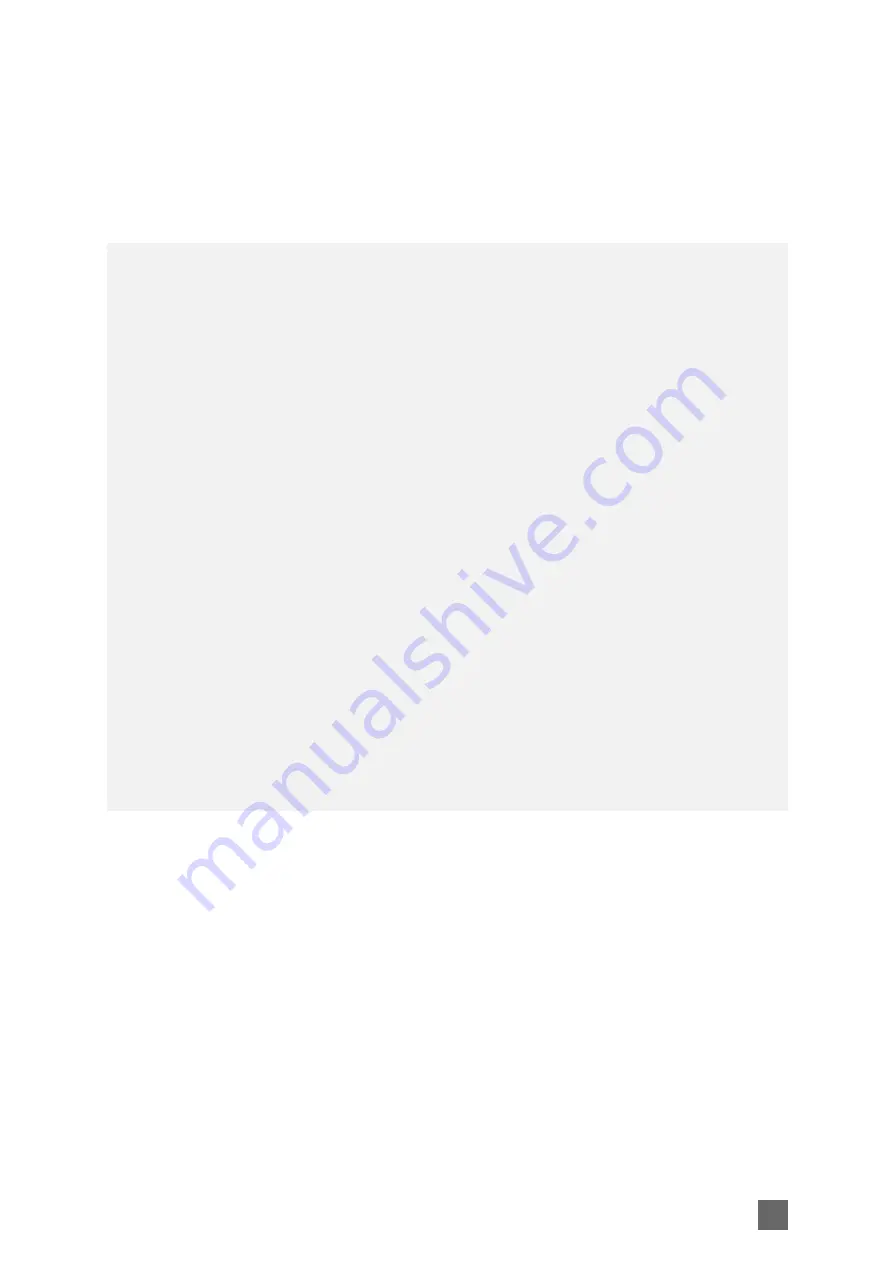
15
5.1. A first example
This example creates a grabber and displays basic information about the interface, device, and
remote device modules it contains:
#include <iostream>
#include <EGrabber.h>
// 1
static const uint32_t CARD_IX = 0;
static const uint32_t DEVICE_IX = 0;
void showInfo() {
Euresys::EGenTL gentl;
// 2
Euresys::EGrabber<> grabber(gentl, CARD_IX, DEVICE_IX);
// 3
std::string card = grabber.getString<Euresys::InterfaceModule>("InterfaceID");
// 4
std::string dev = grabber.getString<Euresys::DeviceModule>("DeviceID");
// 5
int64_t width = grabber.getInteger<Euresys::RemoteModule>("Width");
// 6
int64_t height = grabber.getInteger<Euresys::RemoteModule>("Height");
// 6
std::cout << "Interface:
" << card << std::endl;
std::cout << "Device:
" << dev
<< std::endl;
std::cout << "Resolution:
" << width << "x" << height << std::endl;
}
int main() {
try {
// 7
showInfo();
} catch (const std::exception &e) {
// 7
std::cout << "error: " << e.what() << std::endl;
}
}
1. Include
EGrabber.h
, which defines the
Euresys::EGrabber
class, and includes the other
header files we need (such as
EGenTL.h
and the standard
).
2. Create a
Euresys::EGenTL
object. This involves the following operations:
n
locate and dynamically load the Coaxlink GenTL producer (
coaxlink.cti
);
n
retrieve pointers to the functions exported by
coaxlink.cti
, and make them available
via
Euresys::EGenTL
methods;
n
initialize
coaxlink.cti
(this is done by calling the GenTL initialization function
GCInitLib
).
3. Create a
Euresys::EGrabber
object. The constructor needs the
gentl
object created in
step 2. It also takes as optional arguments the indices of the interface and device to use.
The purpose of the angle brackets (
<>
) that come after
EGrabber
will become clear later. For
now, they can be safely ignored.
5. Euresys::EGrabber
Coaxlink
Programmer Guide