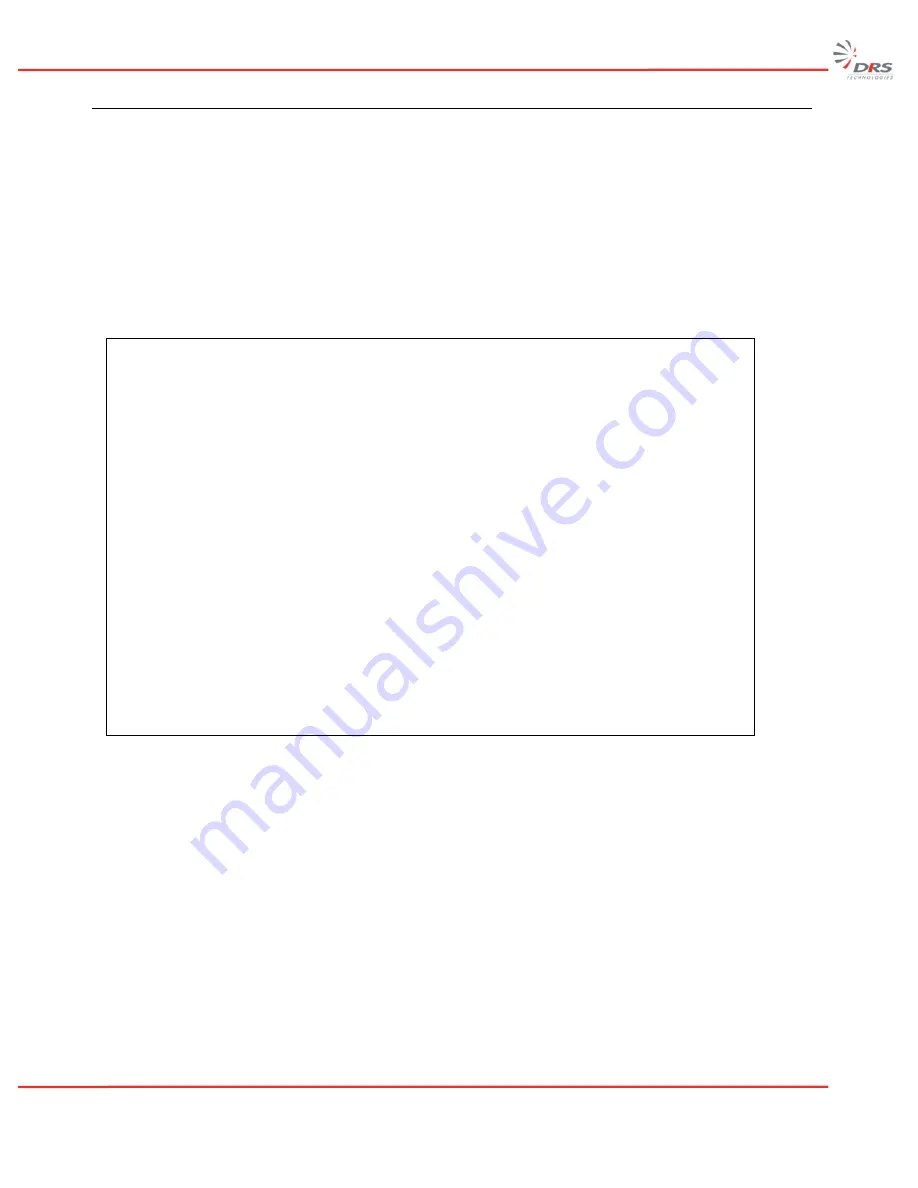
Tamarisk
®
320
Software ICD
7
2.1 CHECKSUM COMPUTATION
Every message has an appended 8-bit checksum. The checksum is computed using all bytes in the
message from the start character to the last data byte.
𝐶ℎ𝑒𝑐𝑘𝑠𝑢𝑚
=
�� −𝑏𝑦𝑡𝑒
(
𝑖
)
𝑁
𝑖=1
� 𝑚𝑜𝑑𝑢𝑙𝑜
256
Note that summing the negative byte values must be performed using 2’s-complement math, which
is common on most processors. Below is example code that computes the message checksum:
Uint8
ComputeChecksum (Uint8 uy_message_id,
Uint8 * pauy_parameters,
Uint32 ul_parameters_size_in_bytes)
{
Uint8 uy_message_checksum = 0 ;
uy_message_checksum -= 0x01 ; // start character
uy_message_checksum -= uy_message_id ;
uy_message_checksum -= ul_parameters_size_in_bytes ;
while
(ul_parameters_size_in_bytes --)
{
uy_message_checksum -= * pauy_para+ ;
}
uy_message_checksum &= 0xFF ;
return
uy_message_checksum ;
}
// ComputeChecksum
Alternatively, the checksum may be computed using the equivalent formula that follows:
Checksum = (~(two’s complement sum of all message byte) + 1)& 0xFF
For example, let’s say that the checksum for 0x01 0x2A 0x02 0x00 0x01 needs to be computed.
The two’s complement sum of all the message bytes would be
0x01 + 0x2A + 0x02 + 0x00 + 0x01 = 0x2E
Next, the formula indicates that the 0x2E must be inverted.
~0x2E = 0xFFF…FFFD1
Then, 1 must be added.0xFFF…FFFD1 + 1 = 0xFFF…FFFD2
Finally, a bit-wise AND with 0xFF needs to be performed.
0xFFF…FFFD2 & 0xFF = 0xD2.