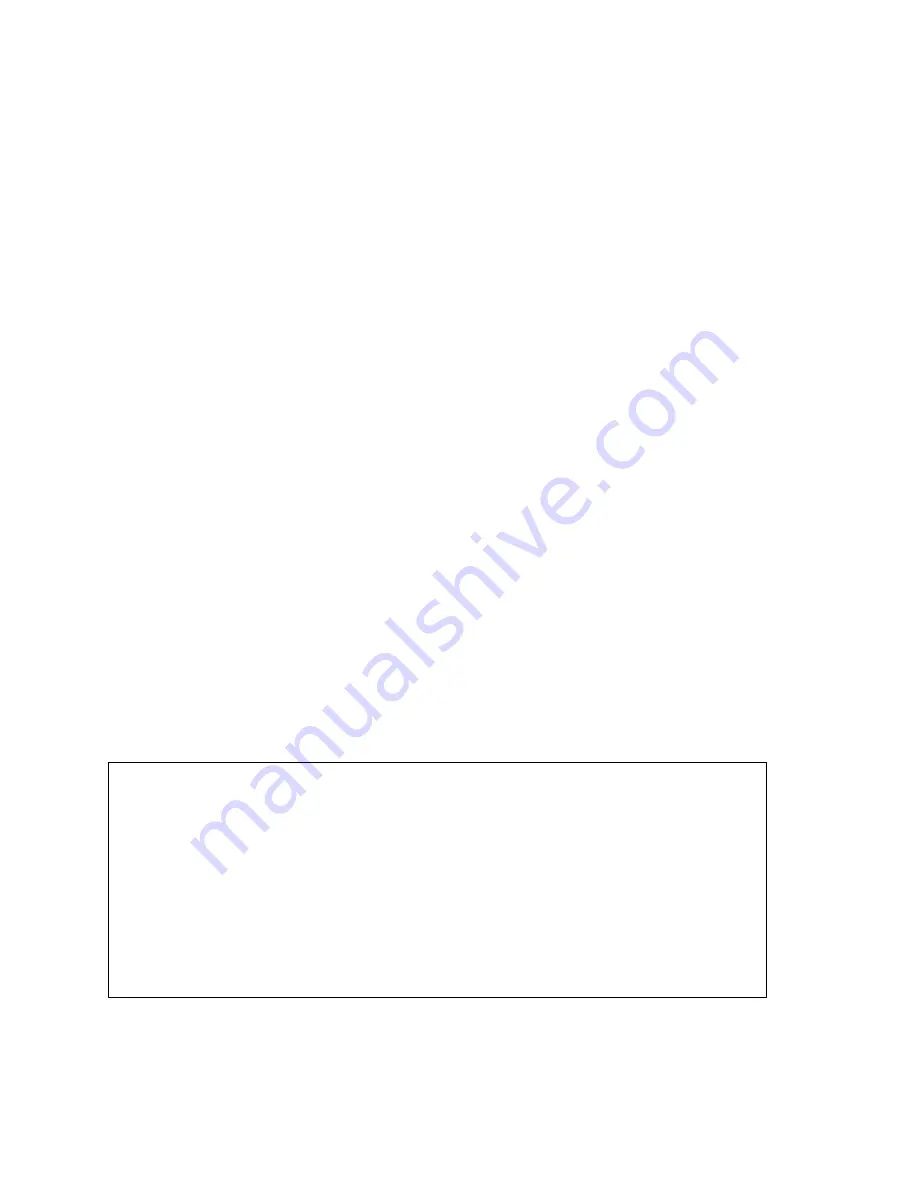
21
Note: If a script is not running to accept that data, the VISA function viWrite will return an I/O
error.
To retrieve data from the input FIFO, the script must call the EM405-8 Extensions Library
function
input
. The EM405-8 Extensions Library functions are detailed in section 3.1. The
input
function takes a single parameter specifying the number of bytes to input. The
input
function is non-blocking meaning that if the specified number of bytes is not available in the
FIFO, the function will return anyway. In addition, being non-blocking also means that the
script must poll for input data either on a regular basis or when data is expected. The return
value of
input
specifies the number of bytes retrieved from the FIFO. There is only one input
FIFO, therefore only one script should input data at any given time. If more than one script calls
input
at the same time, the behavior is indeterminate.
To output data from a script to the host computer, the script can use the EM405-8 Extensions
Library function
output
to place data into the output FIFO. The host computer can in-turn use
the VISA function viRead to retrieve the data from the output FIFO. Unlike
input
, more than
one script can output data to the VXI-11 interface; however, there is no built-in indication as to
which script a piece of data is from nor is there a defined delimiter to separate data from one
script with data from another script. All data is dumped into a single buffer to be read from the
host. It is guaranteed however, assuming no errors, that all data written within a single call to
em405.output
will be contiguous in the buffer. With that said, there is nothing preventing the
scripts themselves from adding an ID tag and a delimiter to the data itself. In this case, it will be
up to the host application to parse the data. Also, in contrast to
input
, there is always something
(i.e. the VXI-11 firmware) waiting to retrieve data from the output FIFO, therefore the user may
call
output
at any time without error.
To illustrate data passing we have created an example script that simply retrieves data from the
VXI-11 interface using the
input
function then echo’s it back to the VXI-11 interface using the
output
function. The Lua source code is found in Figure 6. The host side simply uses viWrite
to send the data and viRead to retrieve the echo.
Figure 6 Data Passing Example
em405 = require "lua_em405"
io.write("Echo Example\n")
io.write("VXI-11 data will be echoed via VXI-11\n")
io.flush()
while 1 do
num_read, buffer = em405.input(256)
if num_read > 0 then
em405.output(buffer, num_read);
end
end