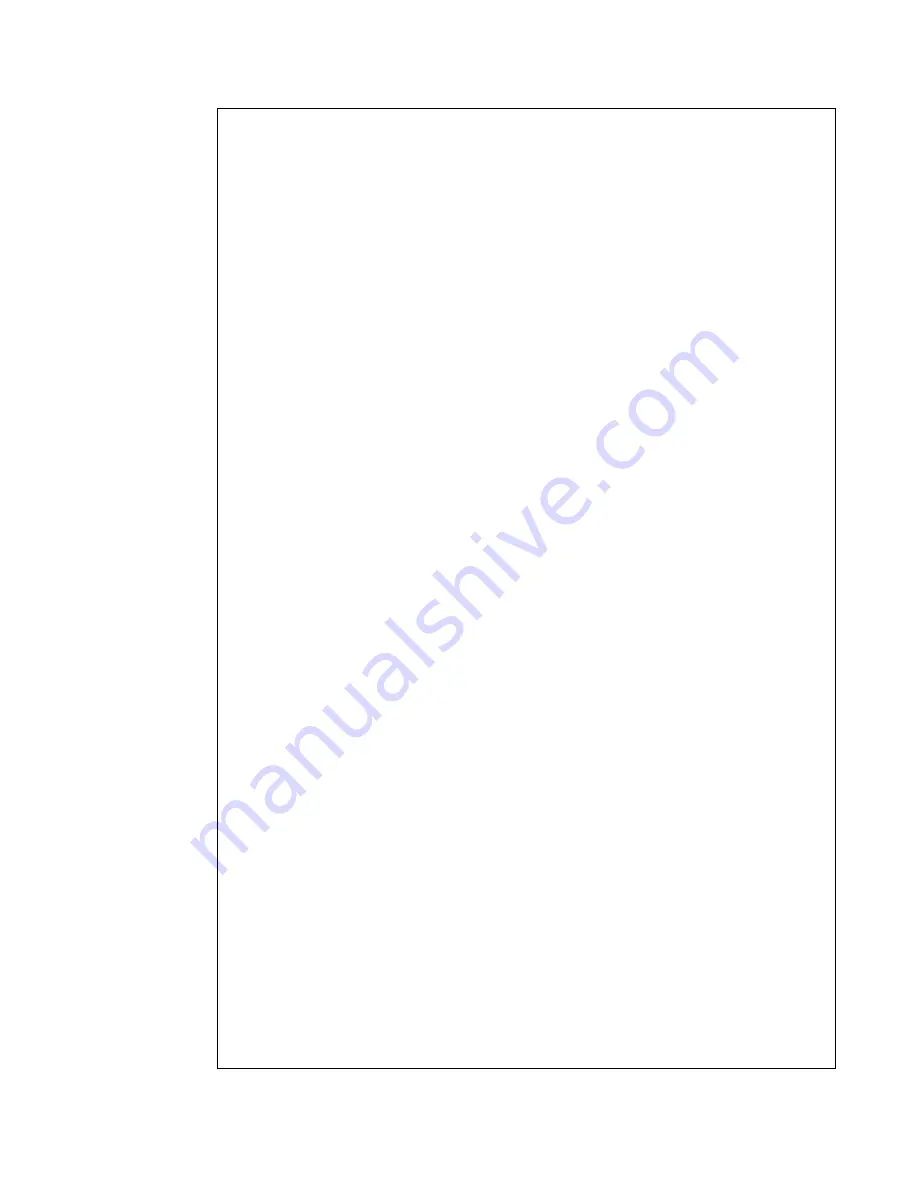
27
' This program demonstrates how to use the CR1000 serial instructions to send an SMS
' using a Intelimax modem
'
' === WIRING ==========================================================================
'
' ComRS232 Intelimax
'
' 1H Thermo, (red stripe)
' 1L Thermocouple -, (blue wire)
'
' === DECLARATIONS ====================================================================
' -- Constants ------------------------------------------------------------------------
Const COMPORT = ComME ' Comport Used for Serial I/O
' -- Public Variables -----------------------------------------------------------------
Public Batt_Volt ' Battery supply voltage
Public P_Temp ' The logger's wiring panel temperature
Public AirTemp_TC ' Air temperature as measured by a thermocouple
Units Batt_Volt = "V"
Units P_Temp = "degC"
Units AirTemp_TC = "degC"
' === SUBROUTINES =====================================================================
Public SMS_PhNo As String ' The cellphone number of the recipient
Public SMS_Msg As String * 160 ' The SMS message to send
Public SMS_Result ' Set to 0 when there has been an error sending an sms
' === DATA TABLES =====================================================================
'Data table to store ten minute temperature data
DataTable (TenMin,1,-1)
DataInterval (0,10,Min,10)
Minimum (1,Batt_Volt,FP2,0,False)
Sample (1,P_Temp,FP2)
Average (1,AirTemp_TC,FP2,False)
Maximum (1,AirTemp_TC,FP2,False,False)
Minimum (1,AirTemp_TC,FP2,False,False)
EndTable
' Datatable to store the last 100 messages sent by the modem
DataTable (SMSSent,True,100)
Sample (1,SMS_Result,FP2)
Sample (1,SMS_PhNo,String)
Sample (1,SMS_Msg,String)
EndTable
Sub SMSSend
' Open the serial port
SerialOpen (COMPORT,115200,3,0,1000)
' Send the SMS command to the modem (eg. AT+CMGS=0412345678) and wait for ">" to be
' returned
SMS_Result = SerialOut (COMPORT,"AT+CMGS=" + CHR(34) + SM CHR(34) +
CHR(13),">",1,100)
' Check to see if we received the response we expected (0 indicates an error)
If SMS_Result <> 0 Then
' Send the SMS message to the modem
SerialOut (COMPORT,SMS_Msg,"",1,100)
' Terminate the message with a CTRL-Z character and wait for CMGS to be returned
SMS_Result = SerialOut (COMPORT,CHR(26),"+CMGS:",1,500)
EndIf
' Close the serial port
SerialClose (COMPORT)