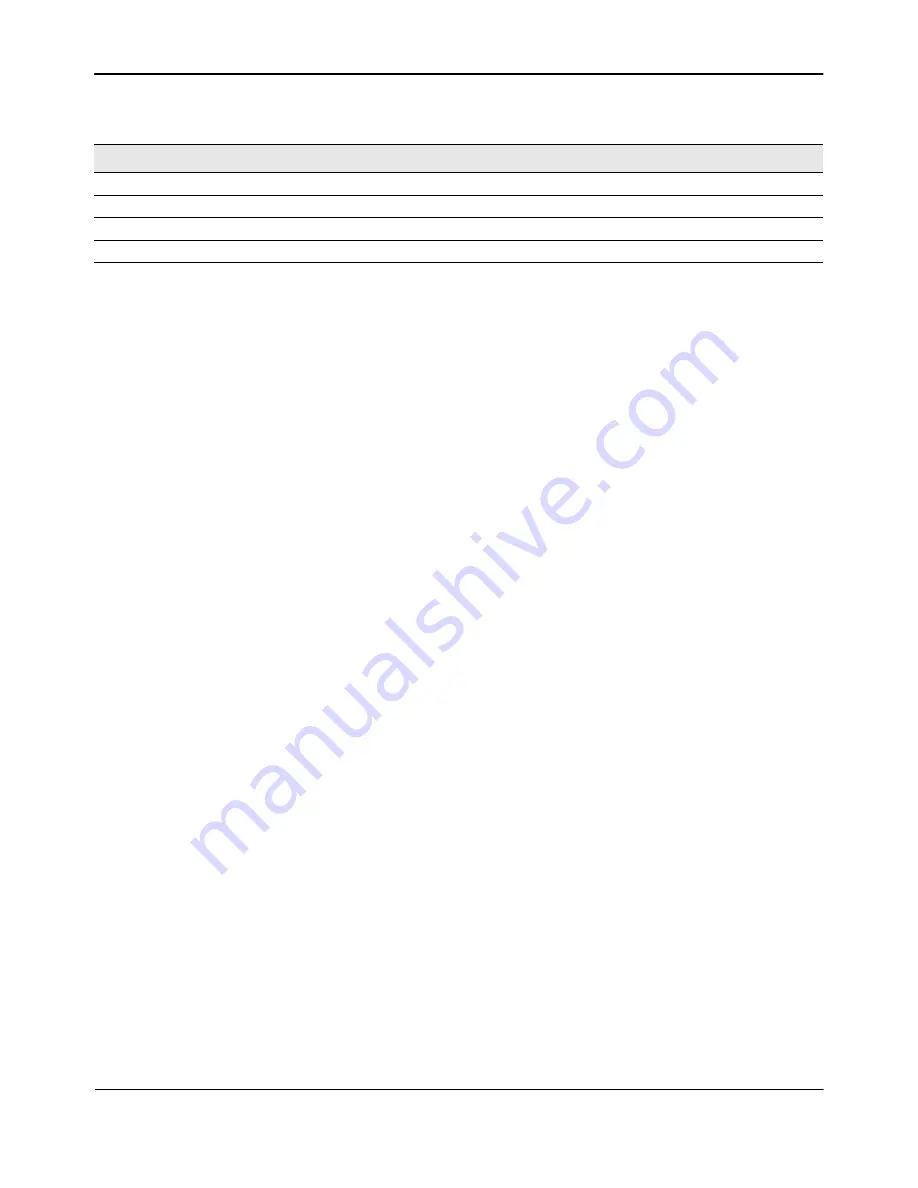
Packet Filtering
BCM5718 Programmer’s Guide
Broadcom
®
January 29, 2016 • 5718-PG108-R
Page 137
The following C code fragment illustrates how to initialize the multicast hash table registers. The code fragment
computes the indices into hash table from a given list of multicast addresses and initializes the multicast hash
registers.
Unsigned long HashReg[4];
Unsigned long j, McEntryCnt;
Unsigned char McTable[32][6];
// List of multicast addresses to accept.
// Initialize the McTable here.
McEntryCnt = 32;
// Initialize the multicast table registers.
HashReg[0] = 0;
// Mac_Hash_Regsiter_0 at offset 0x0470.
HashReg[1] = 0;
// Mac_Hash_Register_1 at offset 0x0474.
HashReg[2] = 0;
// Mac_Hash_Register_2 at offset 0x0478.
HashReg[3] = 0;
// Mac_Hash_Register_3 at offset 0x047c.
for(j = 0; j < McEntryCnt; j++)
{
unsigned long RegIndex;
unsigned long Bitpos;
unsigned long Crc32;
Crc32 = ComputeCrc32(McTable[j], 6);
// The most significant 7 bits of the CRC32 (no inversion),
// are used to index into one of the possible 128 bit positions.
Bitpos = ~Crc32 & 0x7f;
// Hash register index.
RegIndex = (Bitpos & 0x60) >> 5;
// Bit to turn on within a hash register.
Bitpos &= 0x1f;
// Enable the multicast bit.
HashReg[RegIndex] |= (1 << Bitpos);
}
The following C routine computes the Ethernet CRC32 value from a given byte stream. The routine is called
from the above code fragment.
// Routine for generating CRC32.
unsigned long
ComputeCrc32(
unsigned char *pBuffer, // Buffer containing the byte stream.
unsigned long BufferSize) // Size of the buffer.
{
unsigned long Reg;
unsigned long Tmp;
unsigned long j, k;
Reg = 0xffffffff;
for(j = 0; j < BufferSize; j++)
{
Reg ^= pBuffer[j];
Table 37: Multicast Hash Table Registers
Register Name
Offset
Description
Mac_Hash_Register_0
0x0470
Most significant 32-bit of the 128-bit hash table
Mac_Hash_Register_1
0x0474
Bits 64:93 of the 128-bit hash table
Mac_Hash_Register_2
0x0478
Bits 32:63 of the 128-bit hash table
Mac_Hash_Register_3
0x047c
Least significant 32-bit of the 128-bit hash table