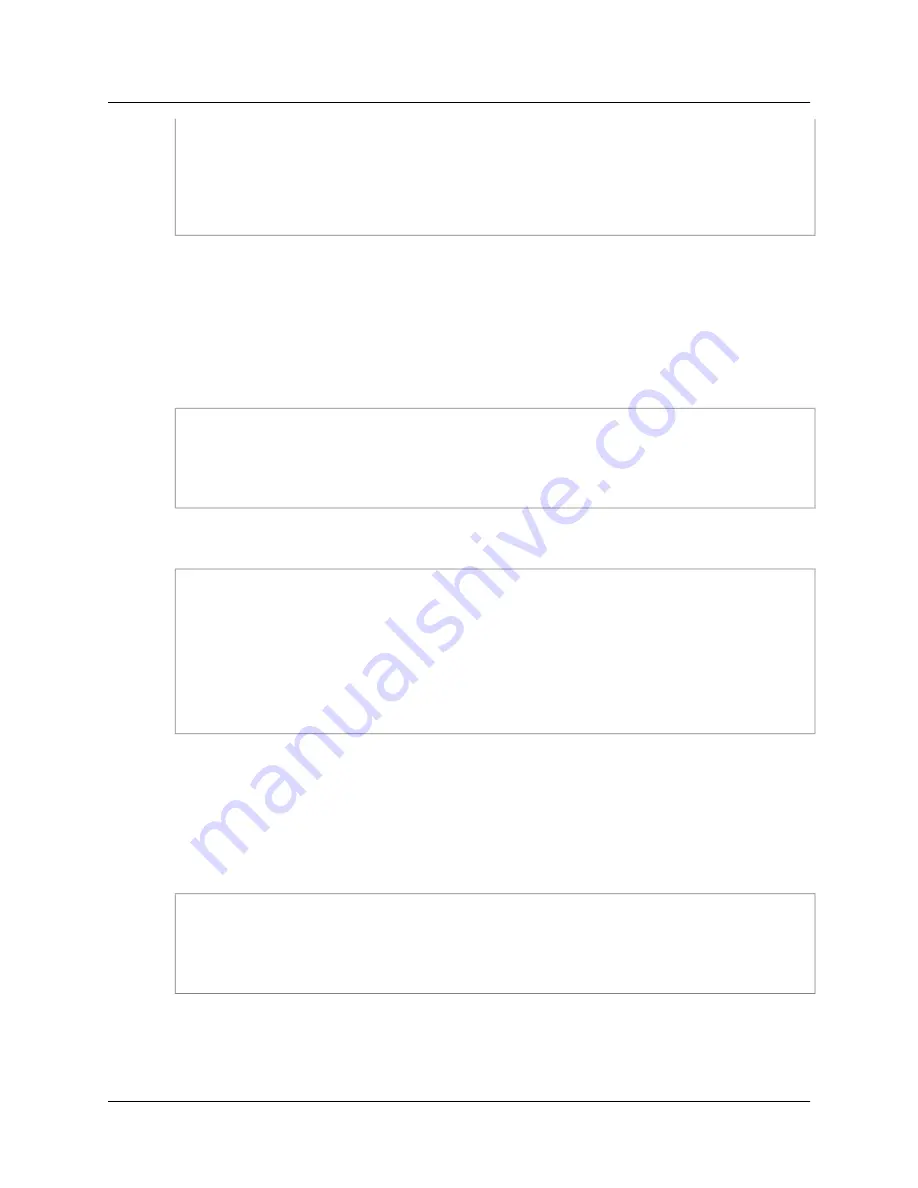
s3.client.createBucket({Bucket: 'myBucket'}, function() {
var data = {Bucket: 'myBucket', Key: 'myKey', Body: 'Hello!'};
s3.client.putObject(data, function() {
console.log("Successfully uploaded data to myBucket/myKey");
});
});
Amazon S3: Streaming Objects to Files on Disk
(getObject)
You can use the
createReadStream()
method on a request object to get a handle to a stream object
which supports piping raw HTTP body data to a file. This is especially useful when streaming objects to
streams like filesystem objects. The following example shows how you can stream an object from Amazon
S3 directly to a file on disk:
var s3 = new AWS.S3();
var params = {Bucket: 'myBucket', Key: 'myImageFile.jpg'};
var file = require('fs').createWriteStream('/path/to/file.jpg');
s3.client.getObject(params).createReadStream().pipe(file);
Alternatively, you can register an 'httpData' event listener on the request object to access each chunk of
data received across the wire (as Buffer objects):
var s3 = new AWS.S3();
var params = {Bucket: 'myBucket', Key: 'myImageFile.jpg'};
var file = require('fs').createWriteStream('/path/to/file.jpg');
s3.client.getObject(params).
on('httpData', function(chunk) { file.write(chunk); }).
on('httpDone', function() { file.end(); }).
send();
Amazon DynamoDB
Amazon DynamoDB: Listing Tables (listTables)
The following example will list all tables in a DynamoDB instance:
var db = new AWS.DynamoDB();
db.client.listTables(function(err, data) {
console.log(data.TableNames);
});
Version 0.9.1-pre.2 : Preview
15
AWS SDK for Node.js Getting Started Guide
Amazon S3: Streaming Objects to Files on Disk
(getObject)