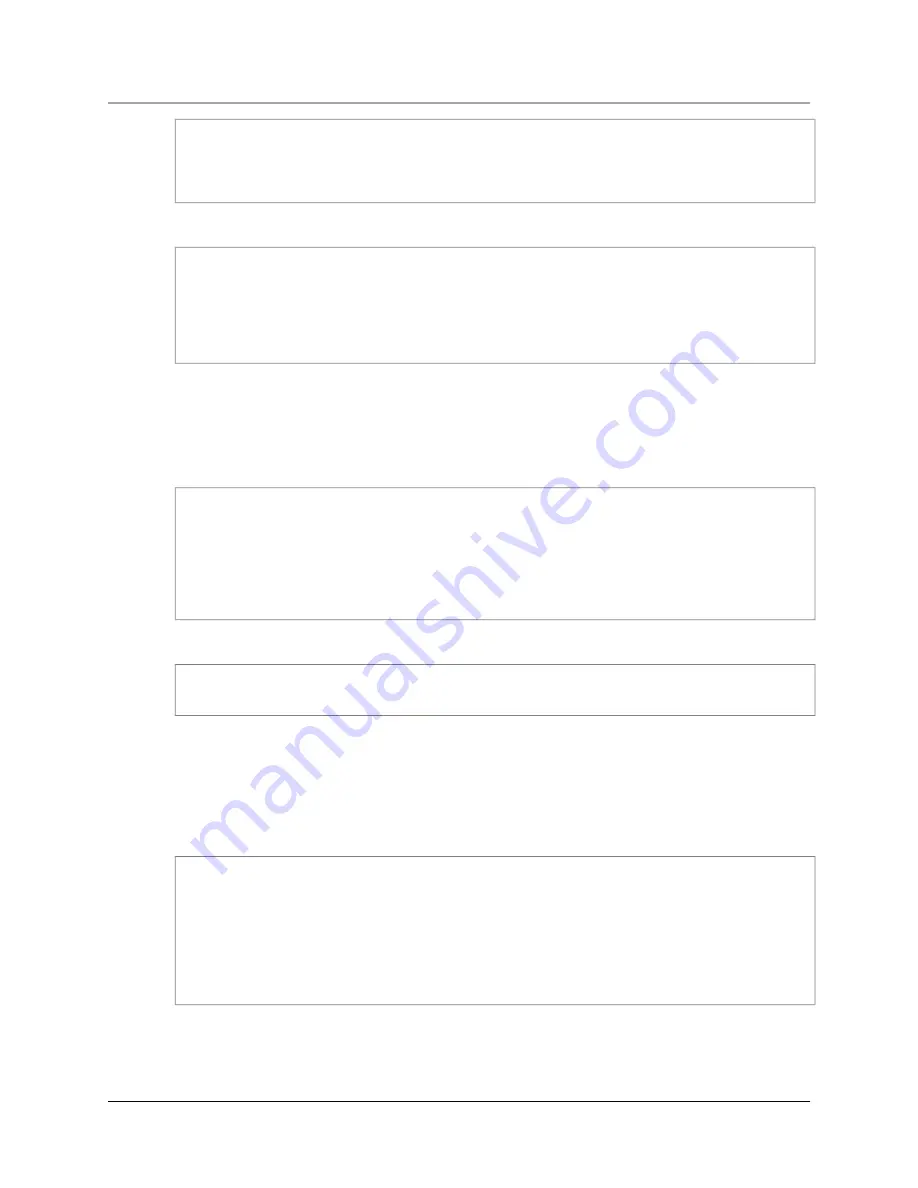
s3.client.listBuckets().done(function(response) {
console.log(response.data);
}).send();
Prints:
{ Owner: { ID: '...', DisplayName: '...' },
Buckets:
[ { Name: 'someBucketName', CreationDate: someCreationDate },
{ Name: 'otherBucketName', CreationDate: otherCreationDate } ],
RequestId: '...' }
on('error', function(error, response) { ... })
The
error
event works similarly to the
success
event, except that it triggers in the case of a request
failure. In this case,
response.data
will be
null
and the
response.error
field will be filled with the
error data. Note that the
error
object is also passed directly as the first parameter to the event:
s3.config.credentials.accessKeyId = 'invalid';
s3.client.listBuckets().fail(function(error, response) {
console.log(error);
// or:
console.log(response.error);
}).send();
Prints:
{ code: 'Forbidden', message: null }
on('complete', function(response) { ... })
The
complete
event triggers a callback in any final state of a request, i.e., both
success
and
error
.
Use this callback to handle any request cleanup that must be executed regardless of the success state.
Note that if you do intend to use response data inside of this callback, you must check for the presence
of
response.data
or
response.error
before attempting to access either property. For example:
request.on('complete', function(response) {
if (response.error) {
// an error occurred, handle it
} else {
// we can use response.data here
}
}).send();
Version 0.9.1-pre.2 : Preview
12
AWS SDK for Node.js Getting Started Guide
on('error', function(error, response) { ...
})