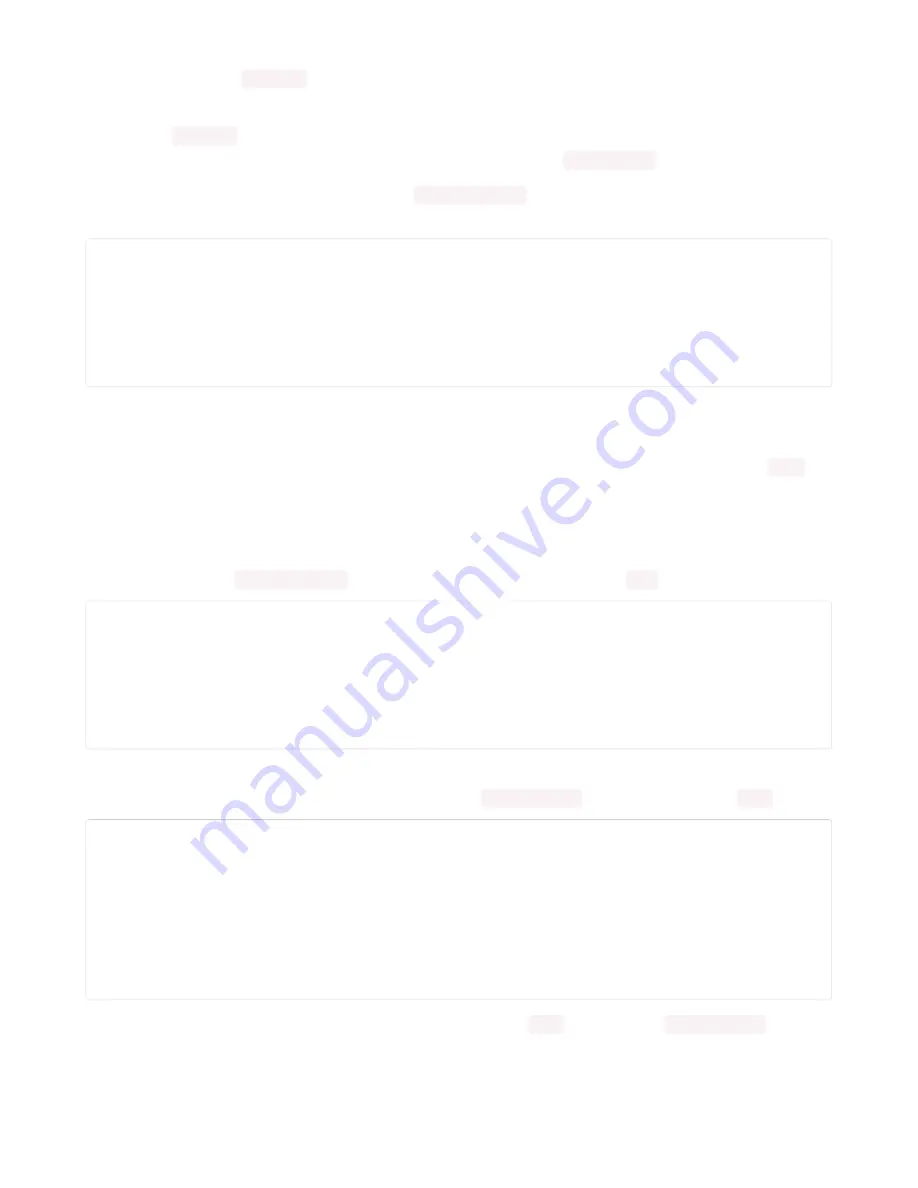
into a variable named
response
.
While we requested data from the server, we'd what the server responded with. Since we already saved
the server's
response
, we can read it back. Luckily for us, requests automatically decodes the server's
response into human-readable text, you can read it back by calling
response.text
.
Lastly, we'll perform a bit of cleanup by calling
response.close()
. This closes, deletes, and collect's the
response's data.
print("Fetching text from %s"%TEXT_URL)
response = requests.get(TEXT_URL)
print('-'*40)
print("Text Response: ", response.text)
print('-'*40)
response.close()
While some servers respond with text, some respond with json-formatted data consisting of attribute–
value pairs.
CircuitPython_Requests can convert a JSON-formatted response from a server into a CPython
dict.
object.
We can also fetch and parse json data. We'll send a HTTP get to a url we know returns a json-formatted
response (instead of text data).
Then, the code calls
response.json()
to convert the response to a CPython
dict
.
print("Fetching JSON data from %s"%JSON_GET_URL)
response = requests.get(JSON_GET_URL)
print('-'*40)
print("JSON Response: ", response.json())
print('-'*40)
response.close()
HTTP POST with Requests
Requests can also POST data to a server by calling the
requests.post
method, passing it a
data
value.
data = '31F'
print("POSTing data to {0}: {1}".format(JSON_POST_URL, data))
response = requests.post(JSON_POST_URL, data=data)
print('-'*40)
json_resp = response.json()
# Parse out the 'data' key from json_resp dict.
print("Data received from server:", json_resp['data'])
print('-'*40)
response.close()
You can also post json-formatted data to a server by passing
json
data into the
requests.post
method.
© Adafruit Industries
https://learn.adafruit.com/adafruit-airlift-shield-esp32-wifi-co-processor
Page 31 of 56