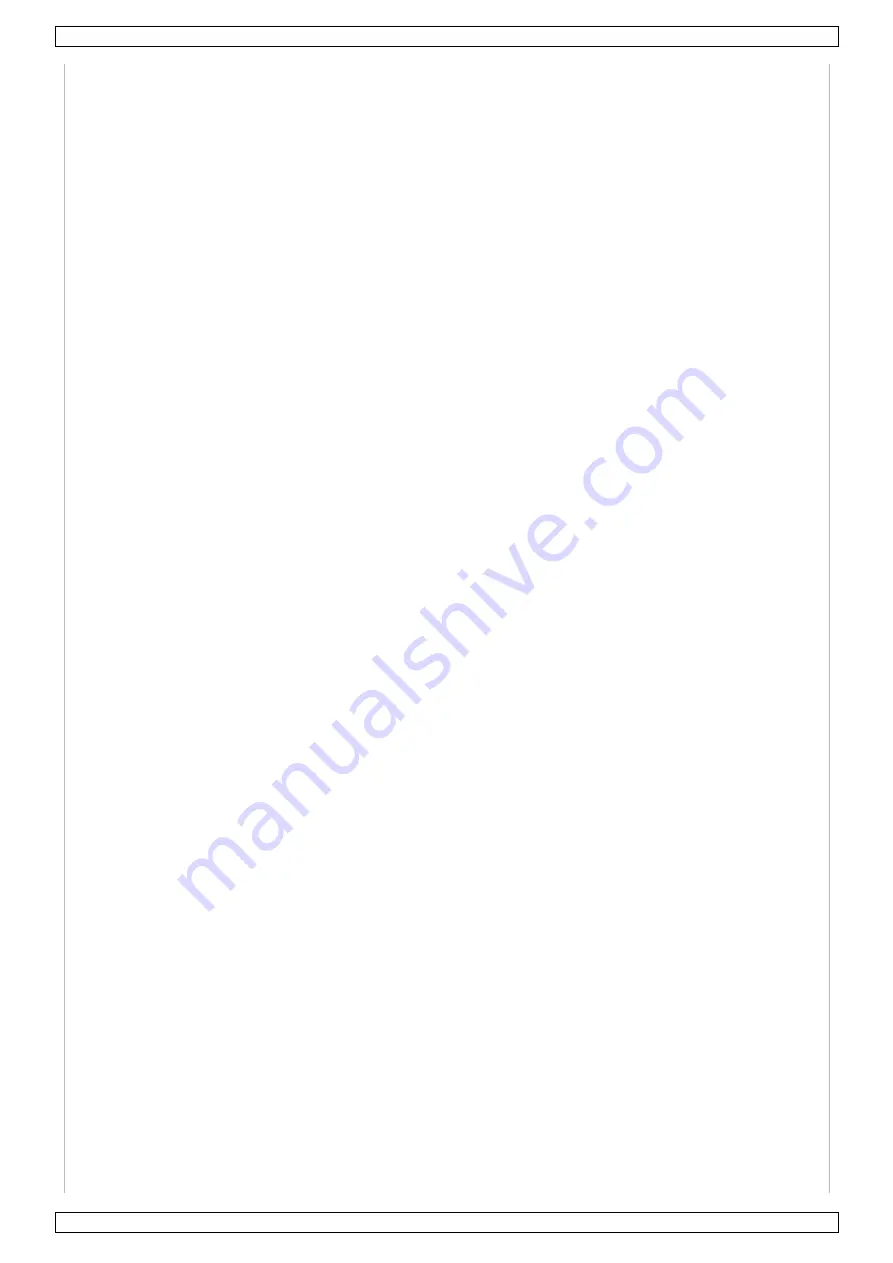
VMA322
V. 02 – 08/05/2019
6
©Velleman nv
unsigned char reg_val;
SPI_PORT&=~CSN; // CSN low, initialize SPI communication...
SPI_RW(reg); // Select register to read from..
reg_val = SPI_RW(0); // ..then read register value
SPI_PORT|=CSN; // CSN high, terminate SPI communication
return(reg_val); // return register value
}
/**************************************************/
/**************************************************
* Function: SPI_Read_Buf();
*
* Description:
* Reads 'unsigned chars' #of unsigned chars from register 'reg'
* Typically used to read RX payload, Rx/Tx address
/**************************************************/
unsigned char SPI_Read_Buf(unsigned char reg, unsigned char *pBuf, unsigned char bytes)
{
unsigned char status,i;
SPI_PORT&=~CSN; // Set CSN low, init SPI tranaction
status = SPI_RW(reg); // Select register to write to and read status unsigned char
for(i=0;i<bytes;i++)
{
pBuf[i] = SPI_RW(0); // Perform SPI_RW to read unsigned char from nRF24L01
}
SPI_PORT|=CSN; // Set CSN high again
return(status); // return nRF24L01 status unsigned char
}
/**************************************************/
/**************************************************
* Function: SPI_Write_Buf();
*
* Description:
* Writes contents of buffer '*pBuf' to nRF24L01
* Typically used to write TX payload, Rx/Tx address
/**************************************************/
unsigned char SPI_Write_Buf(unsigned char reg, unsigned char *pBuf, unsigned char bytes)
{
unsigned char status,i;
SPI_PORT&=~CSN; // Set CSN low, init SPI tranaction
status = SPI_RW(reg); // Select register to write to and read status unsigned char
for(i=0;i<bytes; i++) // then write all unsigned char in buffer(*pBuf)
{
SPI_RW(*pBuf++);
}
SPI_PORT|=CSN; // Set CSN high again