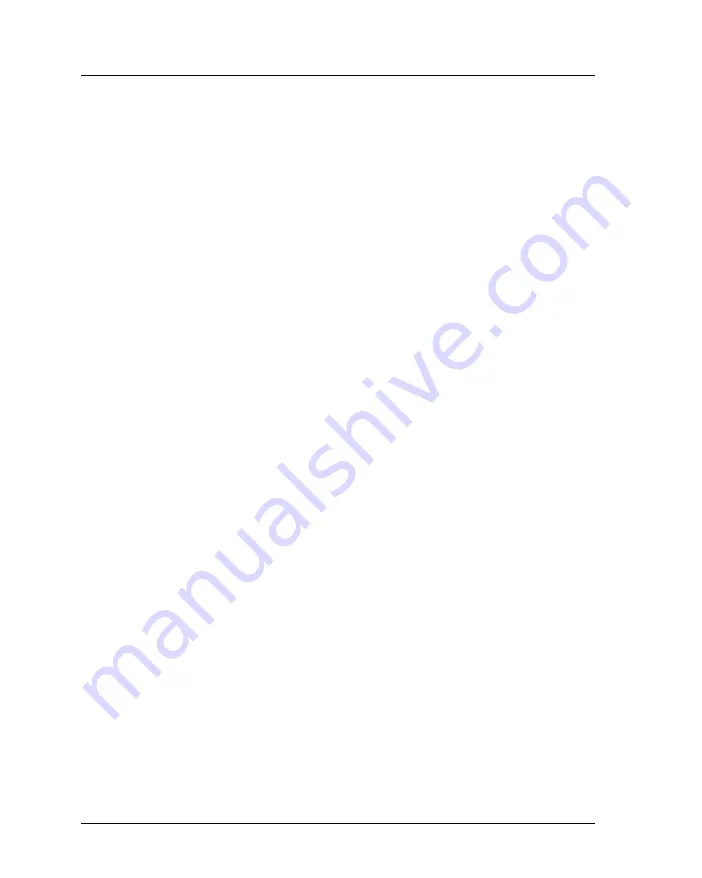
Chapter 4 — Sample Applications
41
FMTestApp
Using the #import Directive
This section describes the steps required to create a COM object using Smart
Pointers and the
#import
compiler directive. The
#import
directive is a
Microsoft-specific compiler directive that creates a Smart Pointer wrapper class
from a type library; that class can be used to create an instance of the required
COM object and to use its services. To use the import directive for an instance
of the Filter Manager interface, you would insert the following code in the
Stdafx.h file after the “#include <Afxwin.h>” directive.
#include <afxdisp.h>// for AfxOleInit
…
#include <atlbase.h>
extern CComModule _Module;
#include <atlcom.h>
#include <objbase.h>
#import “FilterManager.tlb” no_namespace named_guids
The CComModule class implements a COM server. This allows a client to
access the module’s components. When you open your application, you should
call
_Module.Init( NULL, AfxGetInstanceHandle())
. When you close the app, call
_Module.Term()
. For an example, please examine the
InitInstance()
method in
FMTestApp.cpp.
The
#import
directive creates two header files that reconstruct the type library
contents in C++ source code. In this case, the files would be named
FilterMan-
ager.tlh
and
FilterManager.tli
. The primary header file,
FilterManager.tlh
,
contains a typedef macro that expands to the following format:
typedef com_ptr_t< com_IIID<IArgusFM, __uuidof(IArgusFM)> > IArgusFMPtr
The C++ template class
_com_ptr_t
used in the above typedef creates a Smart
Pointer (in this case, IArgusFMPtr) that can be used to access the interface passed
in as the template argument (in this case, IArgusFM).
Once the Smart Pointer interface is defined in FilterManager.tlh, we can create
an instance of a Smart Pointer in our sample application. Note that the only
argument to the
CreateInstance()
method of the Smart Pointer is the class ID of
the Filter Manager component. Because we specified the named_guids modi-
fier, the
#import
directive created the required CLSID in the header file for us,
eliminating the need to call CLSIDFromProgID.
m_pIFilterMgr.CreateInstance ( CLSID_ArgusFM ) ;
Once an instance of IArgusFMPtr has been created, it can be used to access the