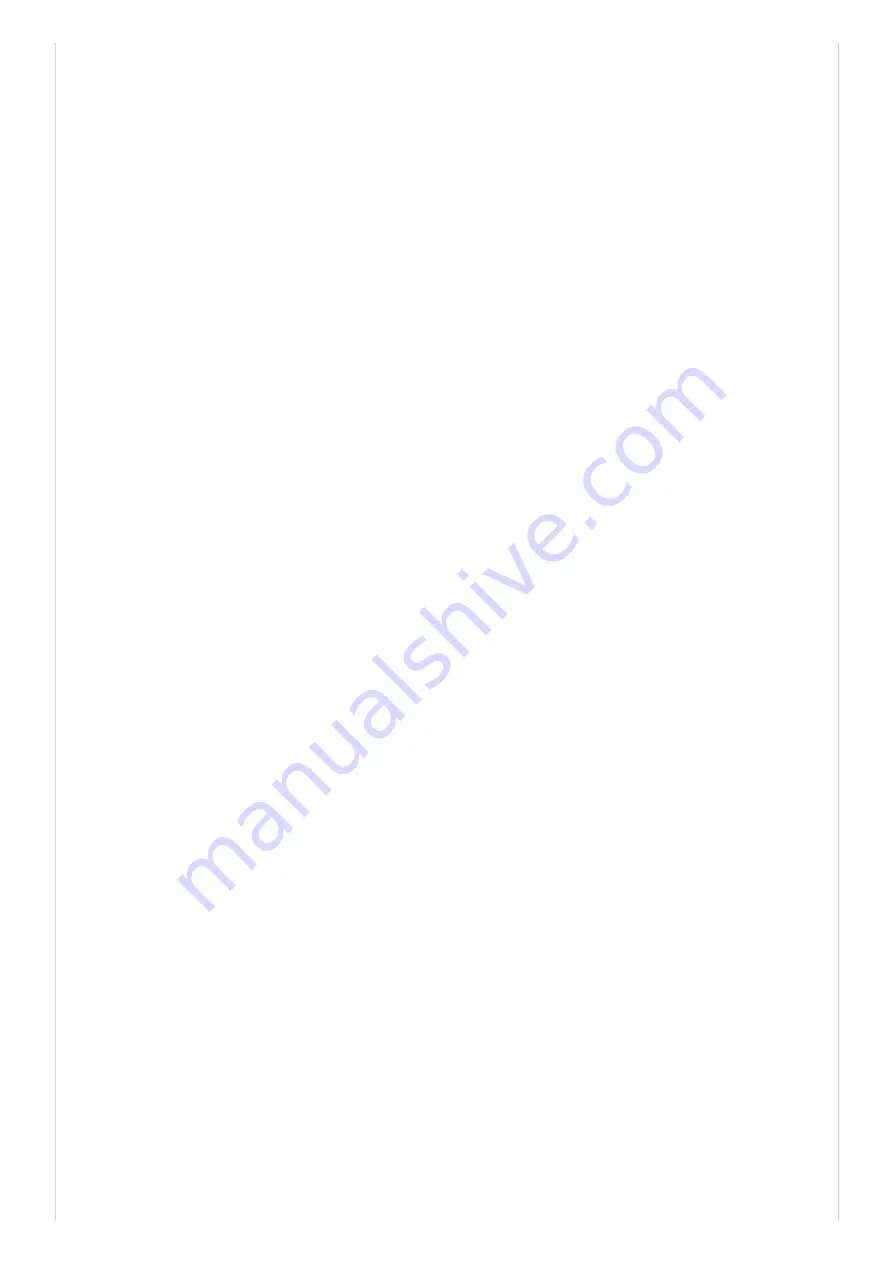
dailyrainin = 0; //Reset daily amount of rain
windgustmph = 0; //Zero out the windgust for the day
windgustdir = 0; //Zero out the gust direction for the day
minutes = 0; //Reset minute tracker
seconds = 0;
lastSecond = millis(); //Reset variable used to track minutes
minutesSinceLastReset = 0; //Zero out the backup midnight reset variable
}
//Calculates each of the variables that wunderground is expecting
void calcWeather()
{
//current winddir, current windspeed, windgustmph, and windgustdir are calculated every 100ms throughout the day
//Calc windspdmph_avg2m
float temp = 0;
for(int i = 0 ; i < 120 ; i++)
temp += windspdavg[i];
temp /= 120.0;
windspdmph_avg2m = temp;
//Calc winddir_avg2m, Wind Direction
//You can't just take the average. Google "mean of circular quantities" for more info
//We will use the Mitsuta method because it doesn't require trig functions
//And because it sounds cool.
//Based on: http://abelian.org/vlf/bearings.html
//Based on: http://stackoverflow.com/questions/1813483/averaging-angles-again
long sum = winddiravg[0];
int D = winddiravg[0];
for(int i = 1 ; i < WIND_DIR_AVG_SIZE ; i++)
{
int delta = winddiravg[i] - D;
if(delta < -180)
D += delta + 360;
else if(delta > 180)
D += delta - 360;
else
D += delta;
sum += D;
}
winddir_avg2m = sum / WIND_DIR_AVG_SIZE;
if(winddir_avg2m >= 360) winddir_avg2m -= 360;
if(winddir_avg2m < 0) winddir= 360;
//Calc windgustmph_10m
//Calc windgustdir_10m
//Find the largest windgust in the last 10 minutes
windgustmph_10m = 0;
windgustdir_10m = 0;
//Step through the 10 minutes
for(int i = 0; i < 10 ; i++)
{
if(windgust_10m[i] > windgustmph_10m)
{
windgustmph_10m = windgust_10m[i];
windgustdir_10m = windgustdirection_10m[i];
}
}
//Calc humidity
humidity = myHumidity.readHumidity();
//float temp_h = myHumidity.readTemperature();
//Serial.print(" TempH:");
//Serial.print(temp_h, 2);
//Calc tempf from pressure sensor
tempf = myPressure.readTempF();
//Serial.print(" TempP:");
//Serial.print(tempf, 2);
//Total rainfall for the day is calculated within the interrupt
//Calculate amount of rainfall for the last 60 minutes
rainin = 0;
for(int i = 0 ; i < 60 ; i++)
= rainHour[i];
//Calc pressure
pressure = myPressure.readPressure();
//Calc dewptf