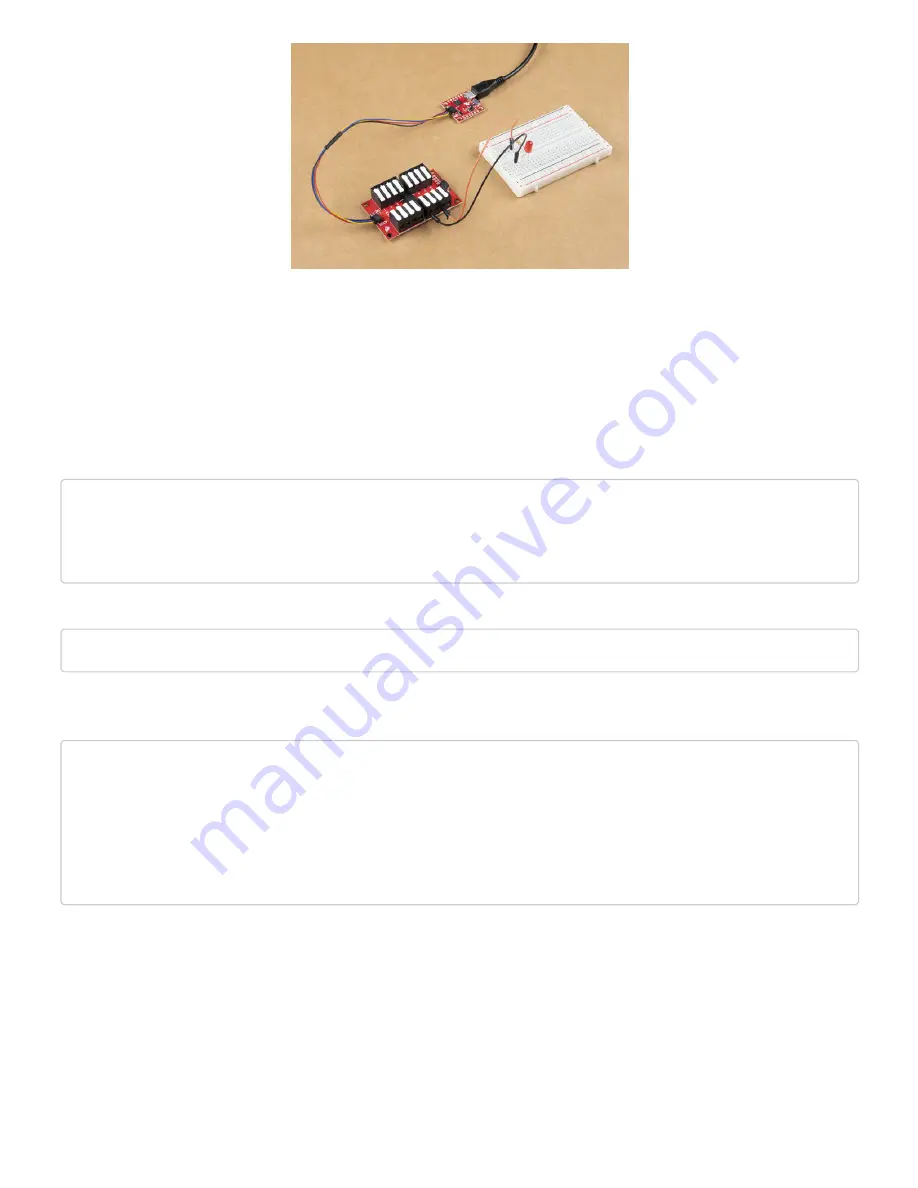
The demo circuit above shows a visual representation of GPIO 0 being toggled HIGH and LOW using an LED. If
you want to control more pins, simply add another
myGPIO.pinMode();
for whichever pin you want to use to set it
as an output and then control it using
myGPIO.digitalWrite();
.
Example 1B: Write GPIO - Port
This version of writing to the Qwiic GPIO controls the entire port (all eight GPIO pins). In order to set up the port
we need to define the number of GPIO pins we are using as well as construct a boolean to define each pin as
either an input or output. Since this example is for writing to the port, all eight pins are defined as outputs:
#define NUM_GPIO 8
bool currentPinMode[NUM_GPIO] = {GPIO_OUT, GPIO_OUT, GPIO_OUT, GPIO_OUT, GPIO_OUT, GPIO_OUT, GPI
O_OUT, GPIO_OUT};
Along with the pin mode, we also need to define the initial state of each GPIO:
bool gpioConfig[NUM_GPIO] = {HIGH, LOW, HIGH, LOW, HIGH, LOW, HIGH, LOW}
With the GPIO port configured, the code initializes the Qwiic GPIO on the I C bus and then alternates the state of
each pin every second using a function specific to this example called
flipGPIO();
:
void flipGPIO()
{
for (uint8_t arrayPosition = 0; arrayPosition < NUM_GPIO; arrayP+)
{
gpioConfig[arrayPosition] = !gpioConfig[arrayPosition];
}
}
Example 2A: Read GPIO
Example 2A demonstrates how to read an individual GPIO pin on the TCA9534. It starts by setting up GPIO 0 as
an input, reads its state and prints out whether it is HIGH or LOW via serial. Similar Example 1, GPIO 0 is set up
but in this case is set as an input using
pinMode(0, GPIO_IN);
. The code then monitors the pin's state every
250ms and prints the state via serial. You can view the main loop below:
2