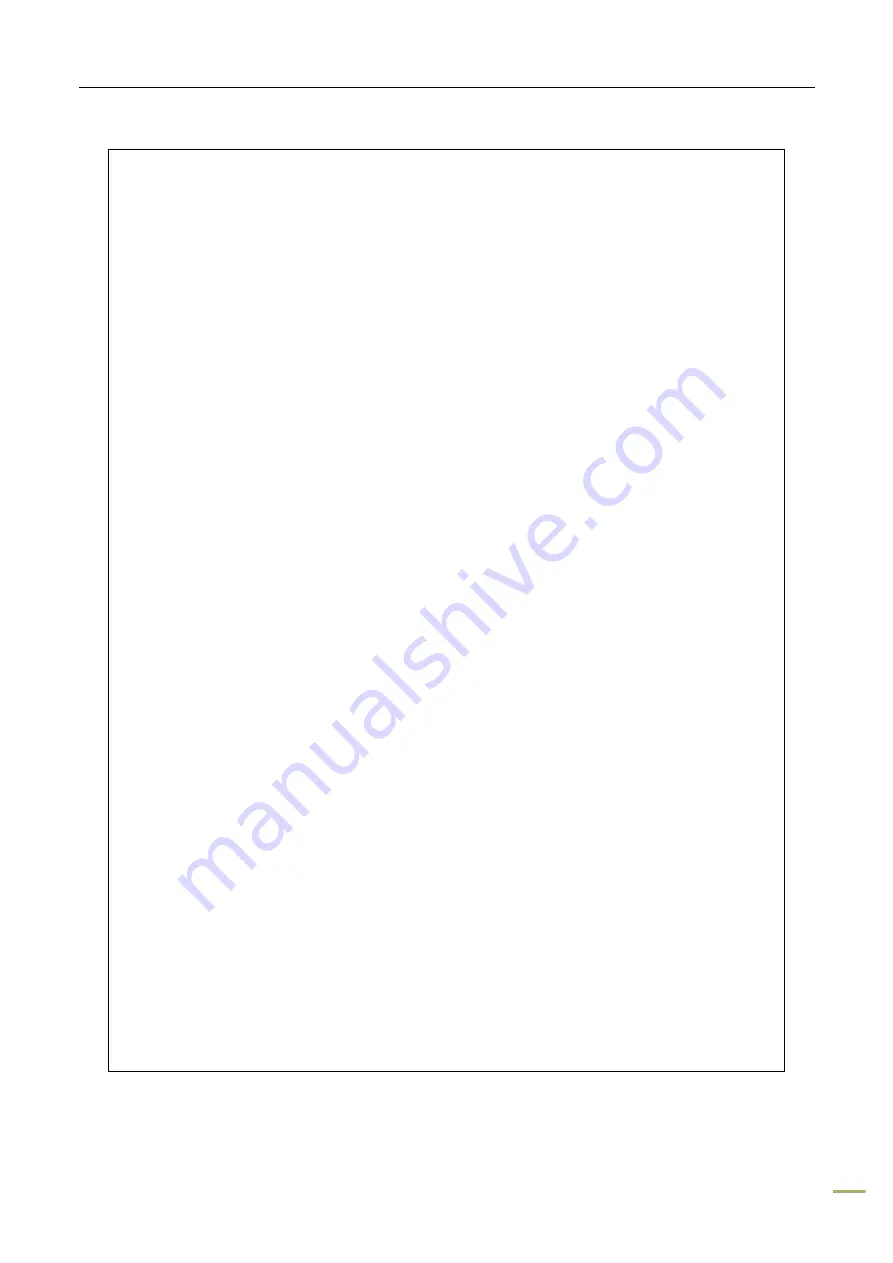
©2014 Seeed Technology Inc.
MB_2014_D02
18
Example of Mode operation
Arduino sketch:
#include <Arduino.h>
/* LED Pin */
int led = 13;
/* declaration */
void sendDataPkt();
void setup() {
pinMode(led, OUTPUT);
/* open the serial port at 115200 bps */
Serial.begin(115200);
}
void loop() {
digitalWrite(led, LOW);
delay(500);
digitalWrite(led, HIGH);
delay(500);
sendDataPkt();
}
void sendDataPkt() {
unsigned char data_pkt[] = {
0x01, //frame ID
0x00, //option
0xf5, //unicast addr high byte
0x28, //unicast addr low byte
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00, //unicast long addr
0x07, //data length
's', //data begin
'e',
'e',
'e',
'd',
'\r',
'\n'
};
int frm_len = sizeof(data_pkt);
unsigned char sum = 0;
for (int i=0; i<frm_len; i++)
{
sum += data_pkt[i];
}
Serial.write(0x7e); //start delimiter
Serial.write(frm_len); //length
Serial.write(0x02); //API identifier: API_DATA_PACKET
Serial.write(data_pkt, frm_len); //API_DATA_PACKET frame
Serial.write(sum); //check sum
}
Notice that the length of the data block can be flexible but with the right
data length specified.