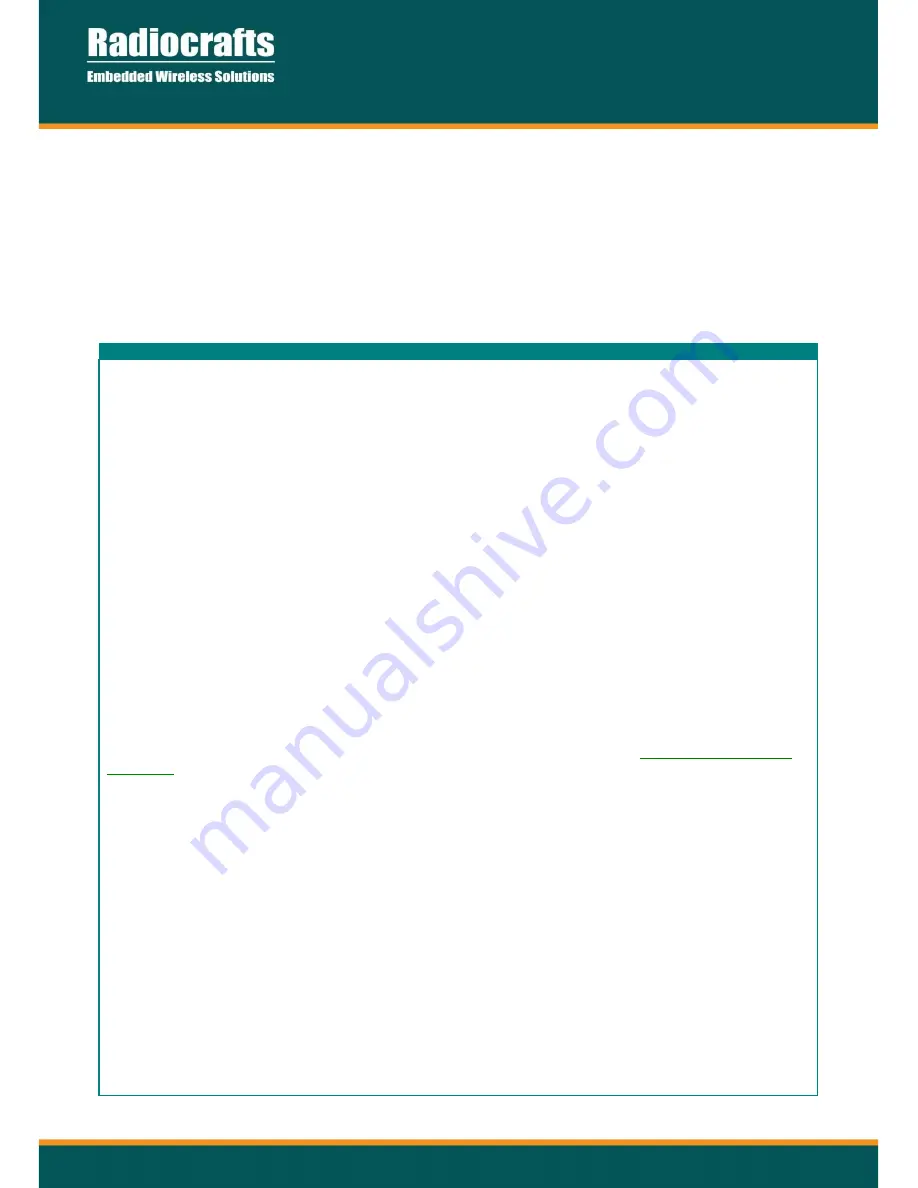
USER MANUAL RC188x-GPR
2019 Radiocrafts AS
RIIM User Manual (rev.1.2)
Page
7
of
23
5
An example
We will show you an example of a wireless voltage sensor using the built in ADC.
This sensor will have the following behaviors:
Finds and joins a network automatically
Every 10 seconds, read data from the ADC
Periodically, send a CoAP PUT message to a CoAP server – in this case the root node
Take a look at the code implementation below. Only basic C knowledge is required. And keep in mind that the
entire code is composed of RIIM_SETUP() and a list of event handlers.
Example : ICI code
#include "RIIM_UAPI.h"
static
const
uint32_t
TimerPeriod
=
10
;
static
uint8_t
CoAP_timer_handler
,
Sensor_timer_handler
;
static
const
uint8_t
Resource_Name
[]=
"data"
;
static
uint32_t
milliVolts
;
static
void
ReadSensor
()
{
ADC
.
convertToMicroVolts
(
ADC0
,
&
milliVolts
);
milliVolts
/=
1000
;
return
;
}
static
void
SendCoAP
()
{
ipAddr RootNodeIPAddr
;
// We first check if we are part of a network and the address of the root node
if
(
Network
.
getRootNodeAddress
(&
RootNodeIPAddr
)!=
UAPI_OK
){
return
;
}
CoAP.connectToServer(&RootNodeIPAddr, false);
// Send temperature and humidity to root node. The URI is "coap://[root-node-
ip]/data"
// For this demo, we'll use JSON formatting. JSON is not size efficient,
// but easy to read and parse
uint8_t
payload
[
100
];
int
payload_length
;
payload_length
=
Util
.
sprintf
((
char
*)
payload
,
"{\"mV\": %i}"
,
milliVolts
);
CoAP
.
send
(
CoAP_PUT
,
false,
Resource_Name
,
payload
,
payload_length
);
return
;
}
void
ResponseHandler
(
const
uint8_t
*
payload
,
uint8_t
payload_size
)
{
Util
.
printf
(
"# Got CoAP Response. Doing nothing with it...\n"
);
return
;
}