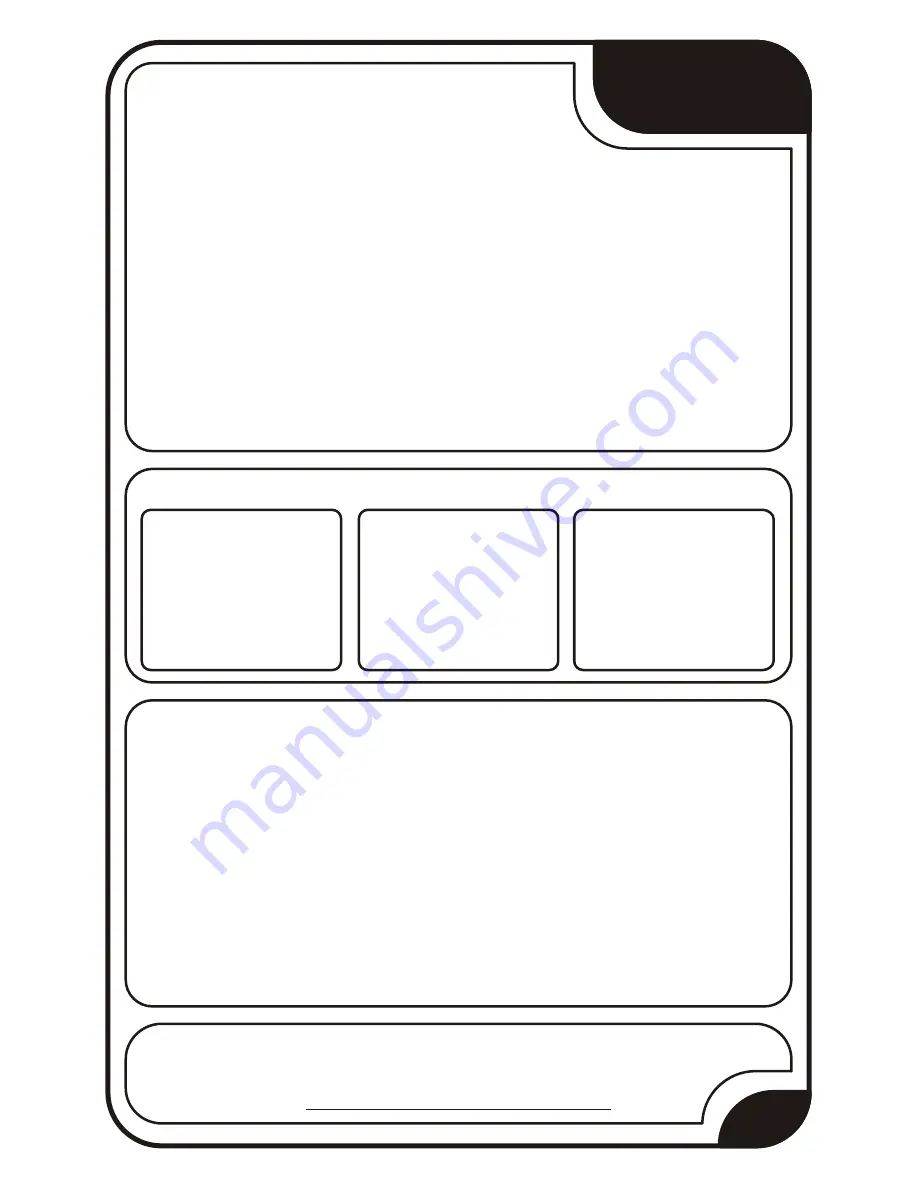
23
CIRC-08
Code
(no need to type everything in just)
File > Examples > Analog > AnalogInput
(example from the great arduino.cc site check it out for other great ideas)
/* Analog Input
* Demonstrates analog input by reading an analog sensor on analog
* pin 0 and turning on and off a light emitting diode(LED) connected to digital pin 13.
* The amount of time the LED will be on and off depends on the value obtained by
* analogRead().
* Created by David Cuartielles
* Modified 16 Jun 2009
* By Tom Igoe
* http://arduino.cc/en/Tutorial/AnalogInput
*/
int sensorPin = 0; // select the input pin for the potentiometer
int ledPin = 13; // select the pin for the LED
int sensorValue = 0; // variable to store the value coming from the sensor
void setup() {
pinMode(ledPin, OUTPUT); //declare the ledPin as an OUTPUT:
}
void loop() {
sensorValue = analogRead(sensorPin);// read the value from the sensor:
digitalWrite(ledPin, HIGH); // turn the ledPin on
delay(sensorValue); // stop the program for <sensorValue> milliseconds:
digitalWrite(ledPin, LOW); // turn the ledPin off:
delay(sensorValue); // stop the program for for <sensorValue> milliseconds:
}
Not Working?
(3 things to try)
More, More, More:
More details, where to buy more parts, where to ask more questions.
http://tinyurl.com/cva3kq
Making it Better
Sporadically Working
This is most likely due to a
slightly dodgy connection with
the potentiometer's pins. This
can usually be conquered by
taping the potentiometer down.
int ledPin = 13; ----> int ledPin = 9;
Threshold Switching:
Then change the loop code to.
Sometimes you will want to switch an output when a
void loop() {
int value = analogRead(potPin) / 4;
value exceeds a certain threshold. To do this with a
analogWrite(ledPin, value);
potentiometer change the
loop()
code to.
}
Upload the code and watch as your LED fades in relation to
void loop() {
int threshold = 512;
your potentiometer spinning. (Note: the reason we divide the
if(analogRead(potPin) > threshold){
digitalWrite(ledPin, HIGH);}
value by 4 is the analogRead() function returns a value from 0
else{ digitalWrite(ledPin, LOW);}
to 1024 (10 bits), and analogWrite() takes a value from 0 to
}
This will cause the LED to turn on when the value is
255 (8 bits) )
above 512 (about halfway), you can adjust the sensitivity
Controlling a Servo:
by changing the
threshold
value.
This is a really neat example and brings a couple of circuits
together. Wire up the servo like you did in CIRC-04, then open
Fading:
Lets control the brightness of an LED directly from the
the example program Knob (
File > Examples > Library-
potentiometer. To do this we need to first change the pin
Servo > Knob
), Upload to your Arduino and then watch as
the LED is connected to. Move the wire from pin 13 to
the servo shaft turns as you turn the potentiometer.
pin 9 and change one line in the code.
22
CIRC-08
What We’re Doing:
.:Twisting:.
.:Potentiometers:.
Along with the digital pins the Arduino has it also
has 6 pins which can be used for analog input.
These inputs take a voltage (from 0 to 5 volts) and
convert it to a digital number between 0 (0 volts) and
1024 (5 volts) (10 bits of resolution). A very useful device that exploits these
inputs is a potentiometer (also called a variable resistor). When it is connected
with 5 volts across its outer pins the middle pin will read some value between 0
and 5 volts dependent on the angle to which it is turned (ie. 2.5 volts in the
middle). We can then use the returned values as a variable in our program.
The Circuit:
Wire
Potentiometer
10k ohm
x1
560 Ohm Resistor
Green-Blue-Brown
x1
2 Pin Header
x4
CIRC-08
Breadboard sheet
x1
Parts:
Green LED
x1
Schematic:
Arduino
pin 13
LED
(light
emitting
diode)
resistor
(560ohm)
(blue-green-brown)
gnd
(ground) (-)
Potentiometer
+5 volts
Arduino
analog
pin 2
.:download:.
breadboard layout sheet
http://tinyurl.com/d62o7q
.:view:.
assembling video
http://tinyurl.com/cormru
The Internet
Working Backwards
There are two ways to fix this,
either switch the red and black
wires connected to the
potentiometer, or turn the
potentiometer around. (sorry
sometimes the factory ships us a
backwards potentiometer)
Not Working
Make sure you haven't accidentally
connected the potentiometer's
wiper to digital pin 2 rather than
analog pin 2. (the row of pins
beneath the power pins)