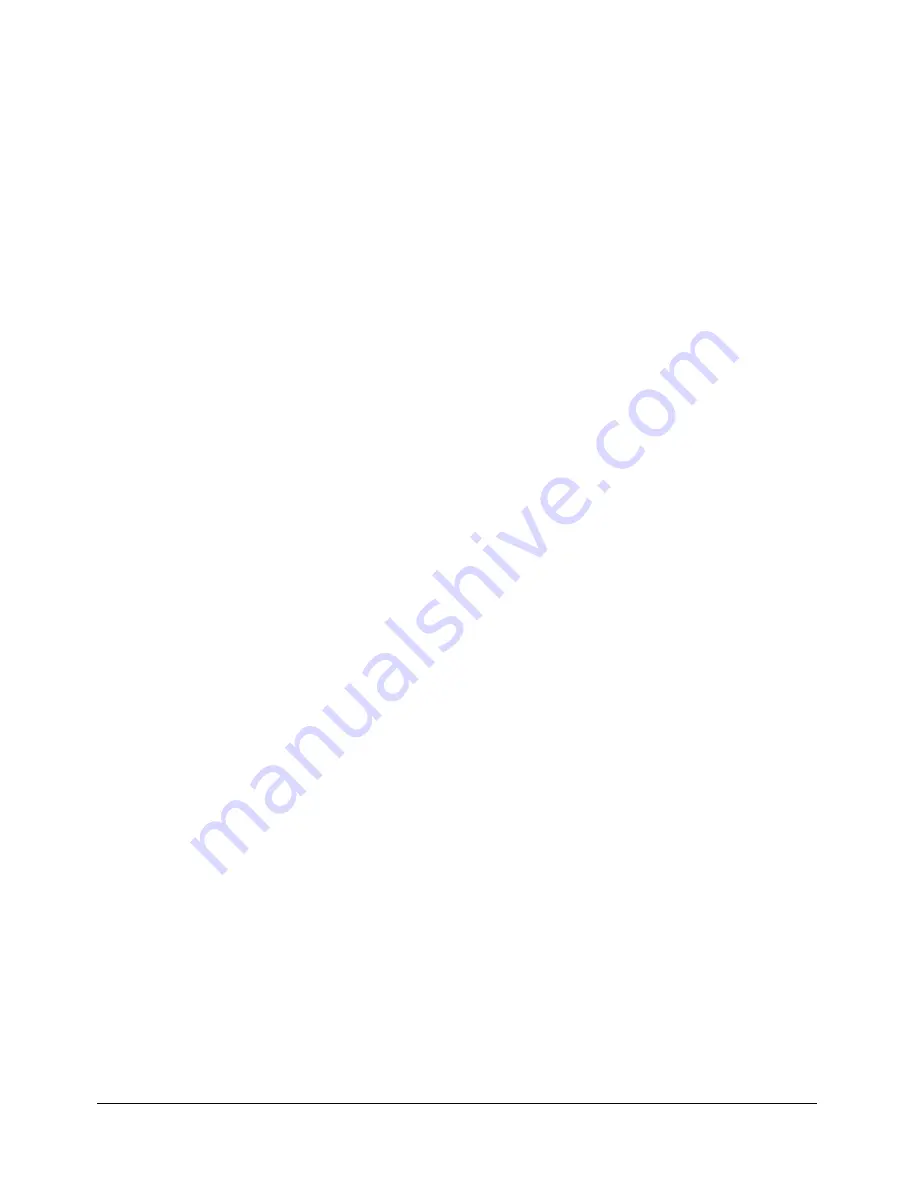
Creating and using interfaces
59
Interfaces cannot contain any variable declarations or assignments. Functions declared in an
interface cannot contain curly braces. For example, the following interface won’t compile:
interface BadInterface{
// Compiler error. Variable declarations not allowed in interfaces.
var illegalVar;
// Compiler error. Function bodies not allowed in interfaces.
function illegalMethod(){
}
}
You can also use the
extends
keyword to create subclasses of an interface:
interface iA extends interface iB {}
The rules for naming interfaces and storing them in packages are the same as those for classes; see
“Creating and using classes” on page 51
and
“Using packages” on page 57
.
Interfaces as data types
Like a class, an interface defines a new data type. Any class that implements an interface can be
considered to be of the type defined by the interface. This is useful for determining if a given
object implements a given interface. For example, consider the following interface:
interface Movable {
function moveUp():Void;
function moveDown():Void;
}
Now consider the class Box, which implements the Movable interface.
class Box implements Movable {
var xpos:Number;
var ypos:Number;
function moveUp():Void {
trace("moving up");
// method definition
}
function moveDown():Void {
trace("moving down");
// method definition
}
}
In another script, such as the following code, where you create an instance of the Box class, you
could declare a variable to be of the Movable type:
import Box;
var newBox:Movable = new Box();
At runtime, you can cast an expression to an interface type. Unlike Java interfaces, ActionScript
interfaces exist at runtime, which allows type casting. If the expression is an object that
implements the interface or has a superclass that implements the interface, the object is returned.
Otherwise,
null
is returned. This is useful if you want to make sure that a particular object
implements a certain interface.
Содержание FLEX-FLEX ACTIONSCRIPT LANGUAGE
Страница 1: ...Flex ActionScript Language Reference...
Страница 8: ......
Страница 66: ...66 Chapter 2 Creating Custom Classes with ActionScript 2 0...
Страница 76: ......
Страница 133: ...break 133 See also for for in do while while switch case continue throw try catch finally...
Страница 135: ...case 135 See also break default strict equality switch...
Страница 146: ...146 Chapter 5 ActionScript Core Language Elements See also break continue while...
Страница 229: ...while 229 i 3 The following result is written to the log file 0 3 6 9 12 15 18 See also do while continue for for in...
Страница 808: ...808 Chapter 7 ActionScript for Flash...
Страница 810: ...810 Appendix A Deprecated Flash 4 operators...
Страница 815: ...Other keys 815 Num Lock 144 186 187 _ 189 191 192 219 220 221 222 Key Key code...
Страница 816: ...816 Appendix B Keyboard Keys and Key Code Values...
Страница 822: ...822 Index...