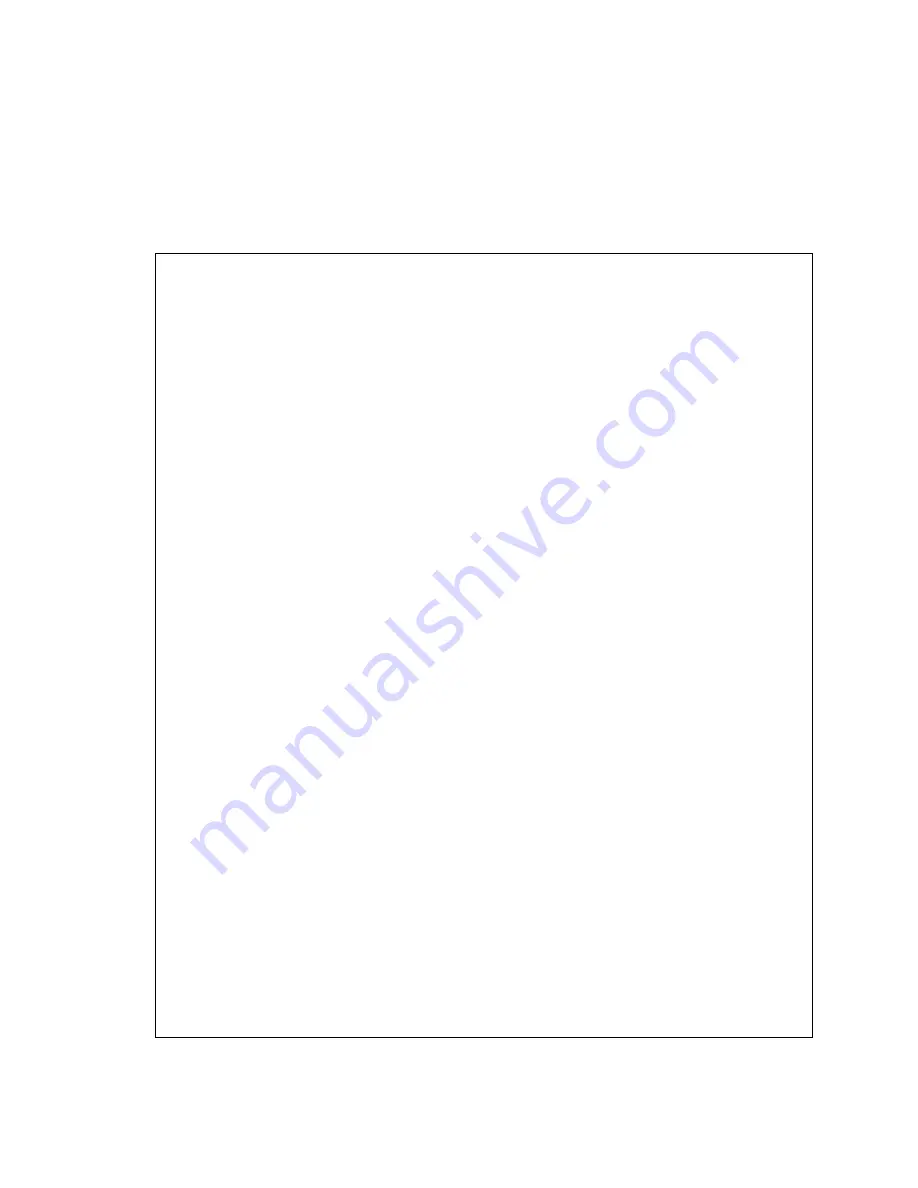
TME-EPIC-HURLX-R3V5.docx
Rev 3.5
Page 43 of 49
4.3
Programming GPIO Signals
The Hurricane LX800 provides 26 General Purpose Input/Output signals (GPIOs) that are part of the ITE8712
SuperIO. These can be programmed either as input or output. Their output voltage is 5V, a 40 Kohm internal
pullup resistor is optional enabled by software. They GPIOs are located in Logical Device 7 of the SuperIO and can
be accessed easily with the SuperIO’s ‘simple IO’ function using port accesses.
The following proramming example is meant to be compiled using gcc under Linux.
#include <sys/io.h>
#include <stdio.h>
#define CONF_ADDR 0x2E
#define CONF_DATA 0x2F
#define GPIO_ADDR 0x1223
//**************************************************************
// InitGPIO: initialize GPIO Bank #4
// Parameter: mode: bit=1 -> set to GPIO
// dir: bit=1/0 -> set to output/input
// (char = 8 bit)
// Returns: -
//*************************************************************
void InitGPIO(char mode,char dir)
{
// To set the SuperIO into configuration mode, the sequence
// 0x87, 0x01, 0x55, 0x55 must be written to the configuration address.
outb(0x87, CONF_ADDR);
outb(0x01, CONF_ADDR);
outb(0x55, CONF_ADDR);
outb(0x55, CONF_ADDR);
// Enable Logical Device 7 for programming by writing 07h to
// register 07h of the SuperIO:
outb(7, CONF_ADDR);
//Set to logic device
outb(7, CONF_DATA); //Number of logic device
// Set GPIO-Set 4 Multifunction Pin Selection Register 28 to GPIO function
// and enable the "simple I/O" function
// Input: mode – each set bit represents a GPIO function
outb(0x28, CONF_ADDR); // set bank #4 to GPIO
outb(inb(CONF_DATA)|mode, CONF_DATA); // BIT: 1=GPIO , 0=other function
outb(0xC3, CONF_ADDR); // set to simple I/O
outb(mode, CONF_DATA); // BIT: 1=simple I/O , 0=not simple I/O
// Define the GPIO's data direction
// Input: dir – each set bit represents an output
outb(0xCB, CONF_ADDR); // set direction: output/input
outb(dir, CONF_DATA); // BIT: 1=output, 0=input
outb(0xBB, CONF_ADDR); // enable pull-ups if acting as output
outb(dir, CONF_DATA); // BIT: 1=pull up, 0=no pull up
// Set the logical I/O address 0x1223 for reading and writing values
outb(0x62, CONF_ADDR); //IO base MSB
outb((GPIO_ADDR & 0xFF00) >> 8, CONF_DATA); // -> 0x03
outb(0x63, CONF_ADDR); //IO base LSB
outb((GPIO_ADDR & 0x00FF), CONF_DATA); // -> 0x93
// Reset configuration mode to "wait for Key"
outb(0x02, CONF_ADDR);
outb(0x02, CONF_DATA);
}
int main()
{
char value1=0x55,value2; //8 bit values
iopl(3); //get all I/O rights
InitGPIO(0xff,0xff); //Initialize GPIO:
//set all to GPIO and all to output
outb(value1, GPIO_ADDR); //write out value1
printf("Write=%x", value1);
value2 = inb(GPIO_ADDR); //read in value2
printf(", Read=%x\n", value2);
return 0;
}
For further information, please refer to chapter 8 of the ITE8712 datasheet.