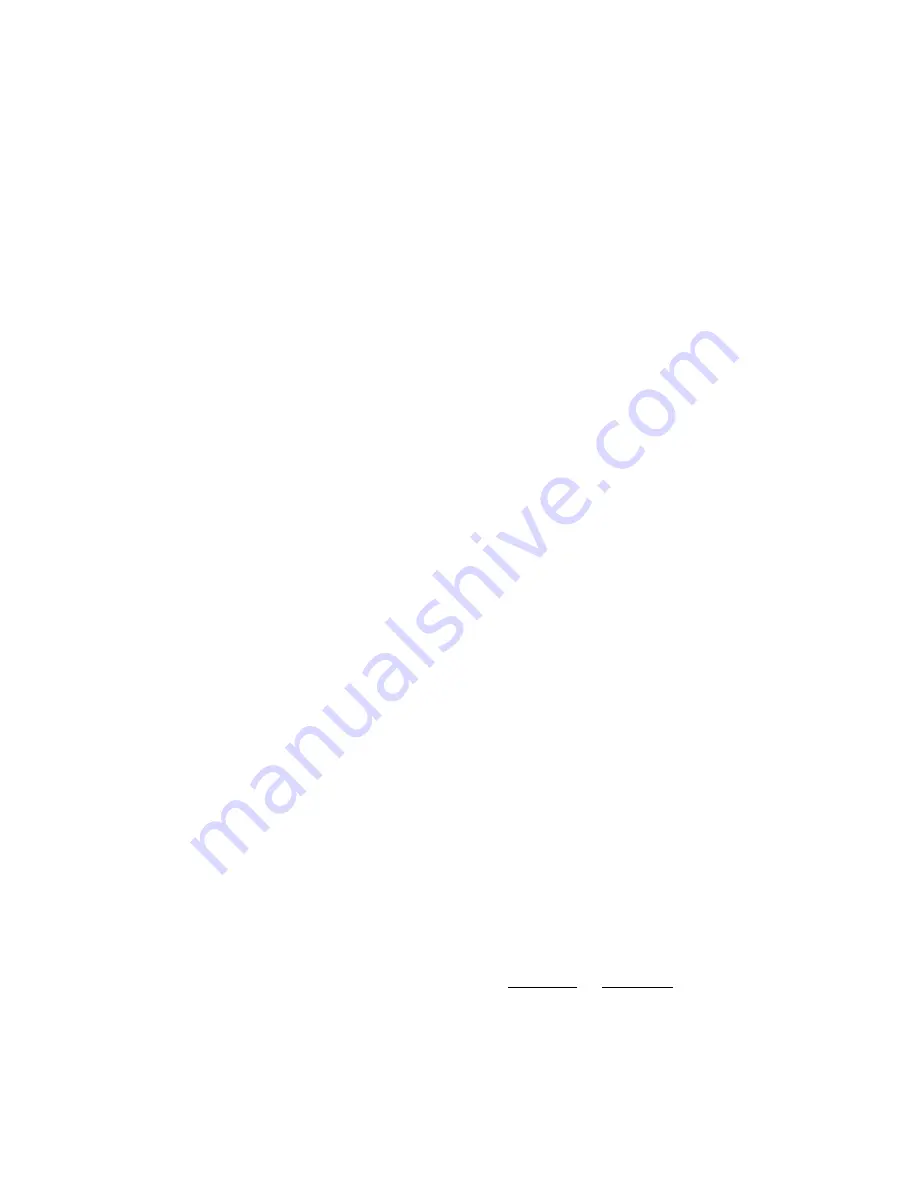
4.3 - Example Pseudocode
The following pseudocode examples are simplified for clarity, and in particular no error checking is shown. The language used for
the pseudocode is C.
4.3.1 - Open
The initial step is to open the LabJack and get a handle that the driver uses for further interaction. The DeviceType for the UE9 is:
LJ_dtUE9
There are two choices for ConnectionType for the UE9:
LJ_ctUSB
LJ_ctETHERNET
Following is example pseudocode to open a UE9 over USB:
//Open the first found LabJack UE9 over USB.
OpenLabJack (LJ_dtUE9, LJ_ctUSB, "1", 1, &lngHandle);
Following is example pseudocode to open a UE9 over Ethernet:
//Open a specified LabJack UE9 over Ethernet.
OpenLabJack (LJ_dtUE9, LJ_ctETHERNET, "192.168.1.209", 0, &lngHandle);
The reason for the quotes around the address is because the address parameter is a string in the OpenLabJack function.
The ampersand (&) in front of lngHandle is a C notation that means we are passing the address of that variable, rather than the
value of that variable. In the definition of the OpenLabJack function, the handle parameter is defined with an asterisk (*) in front,
meaning that the function expects a pointer, i.e. an address.
In general, a function parameter is passed as a pointer (address) rather than a value, when the parameter might need to output
something. The parameter value passed to a function in C cannot be modified in the function, but the parameter can be an
address that points to a value that can be changed. Pointers are also used when passing arrays, as rather than actually passing
the array, an address to the first element in the array is passed.
Talking to multiple devices from a single application is no problem. Make multiple open calls to get a handle to each device and be
sure to set FirstFound=FALSE:
//Open UE9s over USB with Local ID #2 and #3.
OpenLabJack (LJ_dtUE9, LJ_ctUSB, "2", FALSE, &lngHandleA);
OpenLabJack (LJ_dtUE9, LJ_ctUSB, "3", FALSE, &lngHandleB);
… then when making further calls use the handle for the desired device.
4.3.2 - Configuration
There are two IOTypes used to write or read UE9 configuration parameters:
LJ_ioPUT_CONFIG
LJ_ioGET_CONFIG
The following constants are then used in the channel parameter of the config function call to specify what is being written or read:
LJ_chLOCALID
LJ_chHARDWARE_VERSION
LJ_chSERIAL_NUMBER
LJ_chCOMM_POWER_LEVEL
LJ_chIP_ADDRESS
LJ_chGATEWAY
LJ_chSUBNET
LJ_chPORTA
LJ_chPORTB
LJ_chDHCP
LJ_chPRODUCTID
LJ_chMACADDRESS
LJ_chCOMM_FIRMWARE_VERSION
LJ_chCONTROL_POWER_LEVEL
LJ_chCONTROL_FIRMWARE_VERSION
LJ_chCONTROL_BOOTLOADER_VERSION
LJ_chCONTROL_RESET_SOURCE
More information about these parameters can be found in documentation for the low-level CommConfig and ControlConfig
functions.
Following is example pseudocode to write and read the IP address:
//Convert an address string in dot notation to a double.
//The general form of the StringToDoubleAddress function is:
//StringToDoubleAddress (String, *Number, HexDot)
StringToDoubleAddress (“192.168.1.210”, &dblAddress, 0);
//Write a new IP address. Like all Ethernet parameters, this
//will not take effect until the UE9 is reset.
ePut (lngHandle, LJ_ioPUT_CONFIG, LJ_chIP_ADDRESS, dblAddress, 0);
40
Содержание UE9
Страница 84: ...84 ...