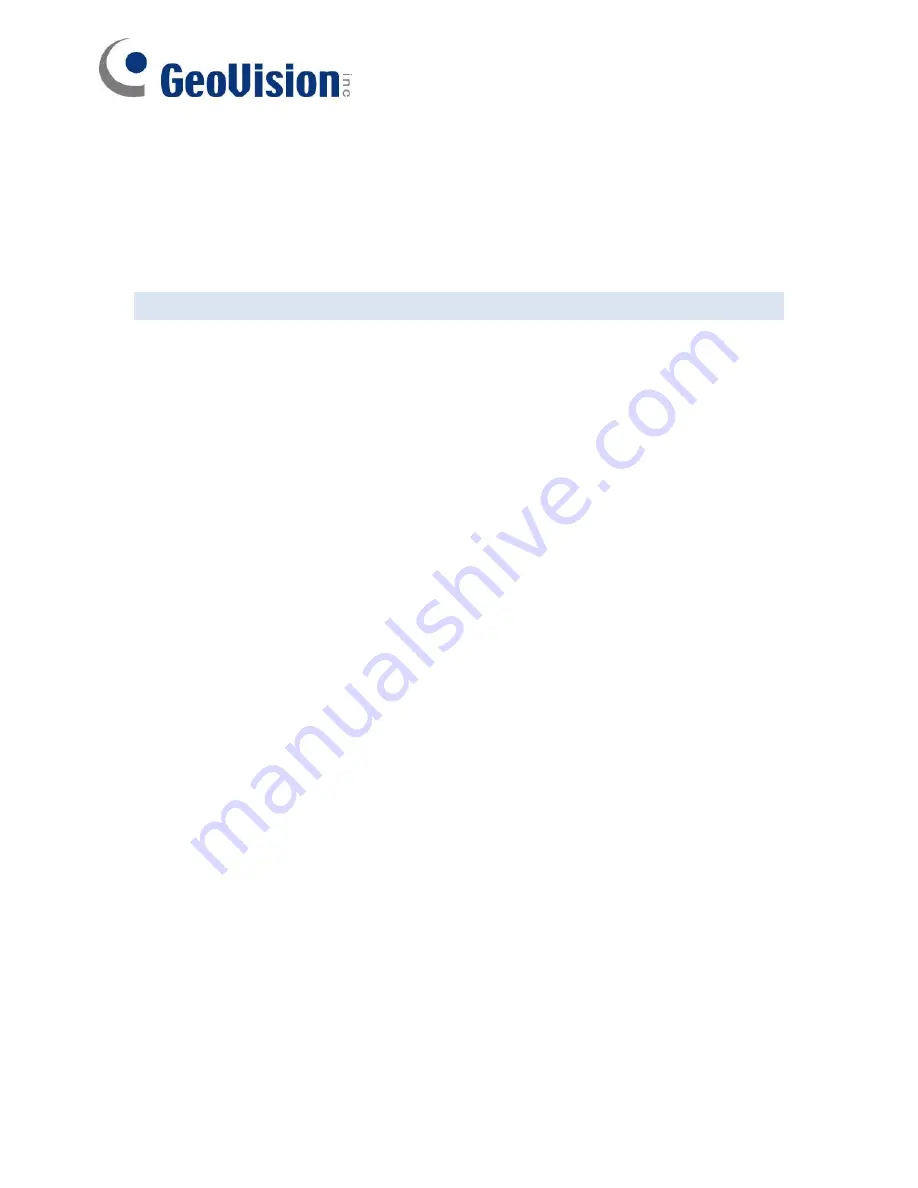
/* Perform the private ioctl */
if(ioctl(skfd,
RTPRIV_IOCTL_SET_WSC_PROFILE_STRING_ITEM
, &lwreq) < 0)
{
fprintf(stderr, "Interface doesn't accept private ioctl...\n");
return -1;
}
5.4
WPS IOCTL Sample Program
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <assert.h>
#include <netinet/in.h> /* for sockaddr_in */
#include <fcntl.h>
#include <time.h>
#include <sys/times.h>
#include <unistd.h>
#include <sys/socket.h> /* for connect and socket*/
#include <sys/stat.h>
#include <err.h>
#include <errno.h>
#include <asm/types.h>
#include </usr/include/linux/wireless.h>
#include <sys/ioctl.h>
#define IFNAMSIZ 16
#define RTPRIV_IOCTL_SET_WSC_PROFILE_U32_ITEM (SIOCIWFIR 0x14)
#define RTPRIV_IOCTL_SET_WSC_PROFILE_STRING_ITEM (SIOCIWFIR 0x16)
enum {
WSC_CREDENTIAL_COUNT = 1,
WSC_CREDENTIAL_SSID = 2,
WSC_CREDENTIAL_AUTH_MODE = 3,
WSC_CREDENTIAL_ENCR_TYPE = 4,
WSC_CREDENTIAL_KEY_INDEX = 5,
WSC_CREDENTIAL_KEY = 6,
WSC_CREDENTIAL_MAC = 7,
WSC_SET_DRIVER_CONNECT_BY_CREDENTIAL_IDX = 8,
WSC_SET_DRIVER_AUTO_CONNECT = 9,
WSC_SET_CONF_MODE = 10, // Enrollee or Registrar
WSC_SET_MODE = 11, // PIN or PBC
WSC_SET_PIN = 12,
WSC_SET_SSID = 13,
WSC_START = 14,
WSC_STOP = 15,
WSC_GEN_PIN_CODE = 16,
};
int main()
{
struct iwreq lwreq;
char
buffer[2048] = {0};
int
cred_count;
int
offset = 0;
/* Space for sub-ioctl index */
int
skfd, i = 0;
/* generic raw socket desc. */
skfd = socket(AF_INET, SOCK_DGRAM, 0);
if (skfd < 0)
return -1;
//////////// WSC_STOP ////////////
33/75