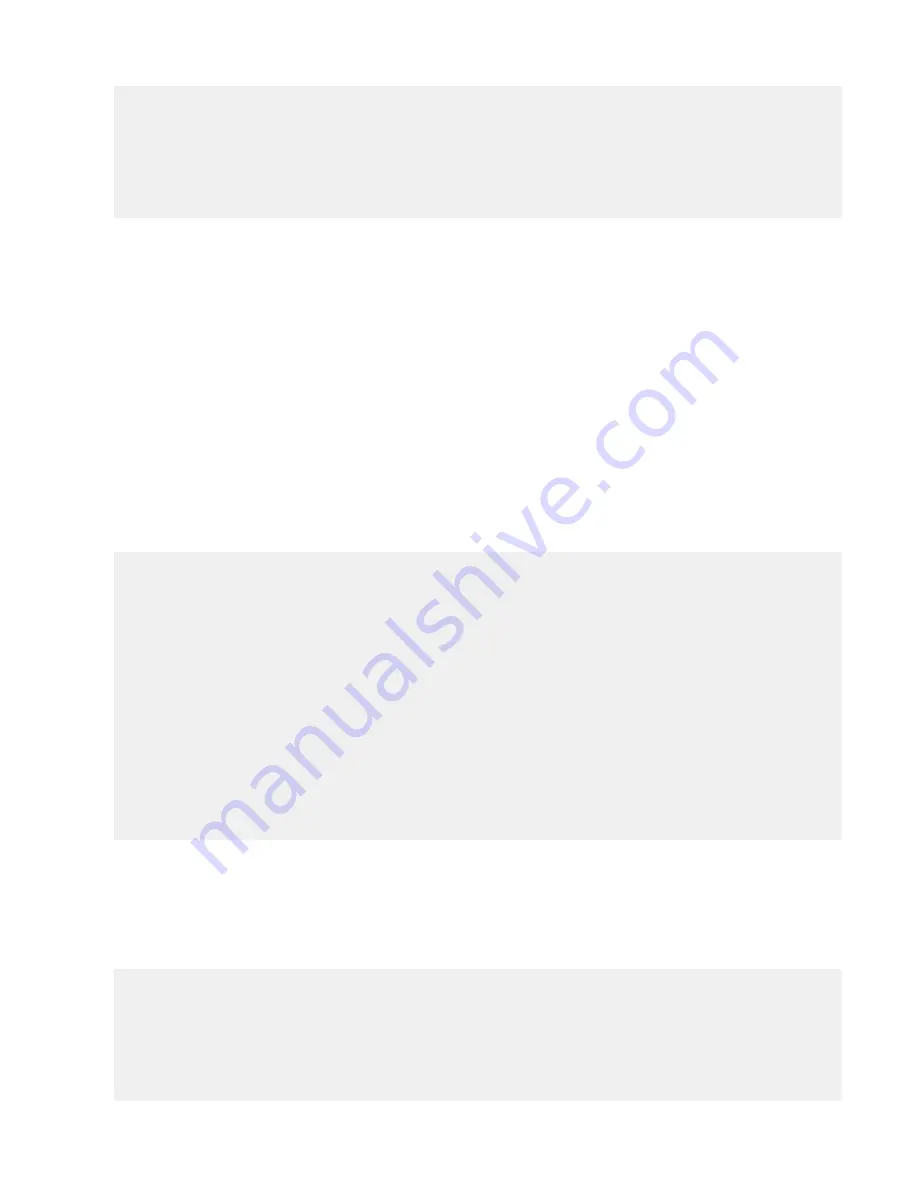
Coaxlink Programmer's Guide
Euresys::EGrabber
EGenTL gentl;
MyGrabber grabber(gentl);
while
(
true
) {
grabber.processEvent<CicData>(1000);
// 7
}
}
catch
(
const
std::exception &e) {
std::cout <<
"error: "
<< e.what() << std::endl;
}
}
1. This
using
directive allows writing
Xyz
instead of
Euresys::Xyz
. This helps keep lines relatively short.
2. Define a new class
MyGrabber
which is derived from
EGrabber<CallbackOnDemand>
.
3.
MyGrabber
's constructor initializes its base class by calling
EGrabber<CallbackOnDemand>
's constructor.
4. Run a
config.js
script which should:
• properly configure the camera and frame grabber;
• enable
notifications
for CIC events.
5. Enable
onCicEvent
callbacks.
6. The
onCicEvent
callback function receives a
const CicData &
. This structure is defined in
include/
EGrabberTypes.h
. It contains a few pieces of information about the event that occurred. Here, we display the
timestamp
and
numid
of each event. The
numid
indicates which CIC event occurred.
7. Call
processEvent<CicData>(1000)
:
• the grabber starts waiting for a CIC event;
• if an event occurs within
1000
ms, the grabber executes the
onCicEvent
callback function;
• otherwise, a
timeout
exception will be thrown.
Example of program output:
timestamp: 1502091779 us, numid: 0x8041 (Start of camera trigger)
timestamp: 1502091784 us, numid: 0x8048 (Received acknowledgement for previous CXP trigger
message)
timestamp: 1502091879 us, numid: 0x8043 (Start of light strobe)
timestamp: 1502092879 us, numid: 0x8044 (End of light strobe)
timestamp: 1502097279 us, numid: 0x8042 (End of camera trigger)
timestamp: 1502097284 us, numid: 0x8048 (Received acknowledgement for previous CXP trigger
message)
timestamp: 1502191783 us, numid: 0x8041 (Start of camera trigger)
timestamp: 1502191783 us, numid: 0x8045 (CIC is ready for next camera cycle)
timestamp: 1502191788 us, numid: 0x8048 (Received acknowledgement for previous CXP trigger
message)
timestamp: 1502191883 us, numid: 0x8043 (Start of light strobe)
timestamp: 1502192883 us, numid: 0x8044 (End of light strobe)
timestamp: 1502197283 us, numid: 0x8042 (End of camera trigger)
timestamp: 1502197288 us, numid: 0x8048 (Received acknowledgement for previous CXP trigger
message)
timestamp: 1502291788 us, numid: 0x8041 (Start of camera trigger)
timestamp: 1502291788 us, numid: 0x8045 (CIC is ready for next camera cycle)
...
Single thread and multi thread callbacks
This program displays basic information about CIC events generated by a grabber, this time using the
CallbackSingleThread
model.
#include <iostream>
#include <EGrabber.h>
using
namespace
Euresys;
class
MyGrabber :
public
EGrabber<CallbackSingleThread> {
// 1
public
:
MyGrabber(EGenTL &gentl) : EGrabber<CallbackSingleThread>(gentl) {
// 2
22