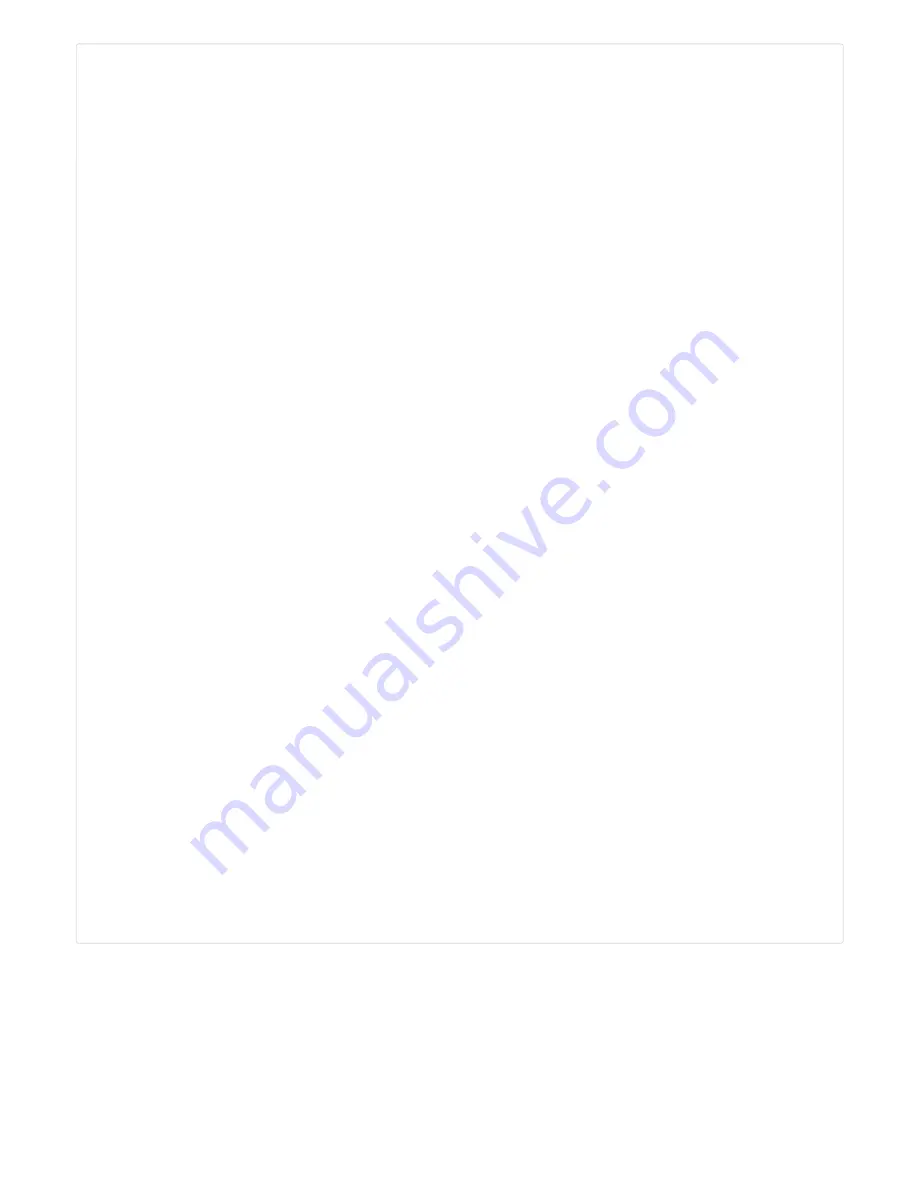
#include <DFRobot_sim808.h>
//make sure that the baud rate of SIM900 is 9600!
//you can use the AT Command(AT+IPR=9600) to set it through SerialDebug
DFRobot_SIM808 sim808(&Serial);
char http_cmd[] = "GET /media/uploads/mbed_official/hello.txt HTTP/1.0\r\n\r\n";
char buffer[512];
void setup(){
//mySerial.begin(9600);
Serial.begin(9600);
//******** Initialize sim808 module *************
while(!sim808.init()) {
delay(1000);
Serial.print("Sim808 init error\r\n");
}
delay(3000);
//*********** Attempt DHCP *******************
while(!sim808.join(F("cmnet"))) {
Serial.println("Sim808 join network error");
delay(2000);
}
//************ Successful DHCP ****************
Serial.print("IP Address is ");
Serial.println(sim808.getIPAddress());
//*********** Establish a TCP connection ************
if(!sim808.connect(TCP,"mbed.org", 80)) {
Serial.println("Connect error");
}else{
Serial.println("Connect mbed.org success");
}
//*********** Send a GET request *****************
Serial.println("waiting to fetch...");
sim808.send(http_cmd, sizeof(http_cmd)‐1);
while (true) {
int ret = sim808.recv(buffer, sizeof(buffer)‐1);
if (ret <= 0){
Serial.println("fetch over...");
break;
}
buffer[ret] = '\0';
Serial.print("Recv: ");
Serial.print(ret);
Serial.print(" bytes: ");
Serial.println(buffer);
break;
}
//************* Close TCP or UDP connections **********
sim808.close();
//*** Disconnect wireless connection, Close Moving Scene *******
sim808.disconnect();
}
void loop(){
}
Get GPS Data
This example tests SIM808 GPS/GPRS/GSM Shield's ability to read GPS data.
1. Open the `SIM808_GetGPS` example or copy the code to your project
2. Download and set the function switch to `Arduino`
3. Open the serial terminal
4. Place the shield outside, wait for a few minutes and it will send GPS data to serial terminal