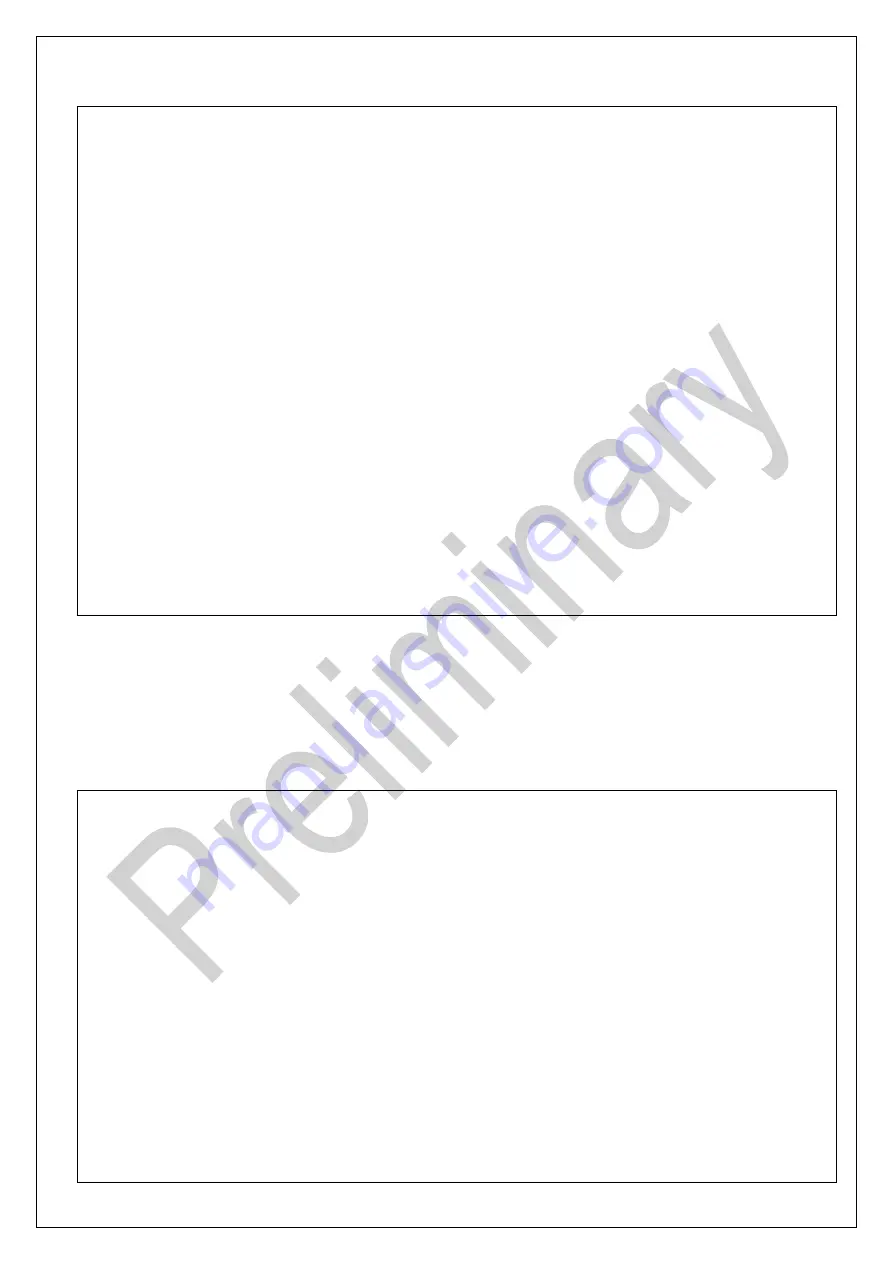
event.value = 1;
write(key_fd,&event,sizeof(event));
}
/*
* Function name : Keyup_Event
* Description : This function is keyup event.
*/
void Serial_Daemon::Keyup_Event(char key_up[10])
{
struct input_event event;
char Receive_Packetf[10] = {0, };
strcpy(Receive_Packetf,key_up);
//Input key event
memset(&event,0,sizeof(event));
event.type = EV_KEY;
event.code = Receive_Packetf[4];
event.value = 0;
write(key_fd,&event,sizeof(event));
//Sync event
memset(&event,0,sizeof(event));
event.type = EV_SYN;
event.code = SYN_REPORT;
event.value = 0;
write(key_fd,&event,sizeof(event));
}
4.4.
Examples using event filter
Control LED and Buzzer of DTP10-L by using event filter of serial daemon program provided by Daincube.
If you makes keyboard event of predefined key code, you can control LED and buzzer.
However, it will work only active mode. Do not work inactive mode (hide mode, hidden mode,
etc.)
Therefore you can refer to the event filter section of serial daemon ini your application to control
the LE and buzzer through keyboard events.
/* Function name : eventFilter
* description : This function is receive the keyboard events and control the LED or Buzzer.
* It is possible only when serial daemon application is active mode.
* If you want to control the LED or Buzzer using keyboard events in your
application, refer to this function.
*/
bool Serial_Daemon::eventFilter(QObject *object, QEvent *event)
{
char sel, data;
QKeyEvent *Keyevent = static_cast <QKeyEvent *>(event);
if(event->type() == QEvent::KeyPress)
{
if((Keyevent->key() == Qt::Key_A) ||
(Keyevent->key() == Qt::Key_B) ||
(Keyevent->key() == Qt::Key_C) ){
data = 0x1; //blue
}
else if((Keyevent->key() == Qt::Key_D) ||
(Keyevent->key() == Qt::Key_E) ||
(Keyevent->key() == Qt::Key_F) ){