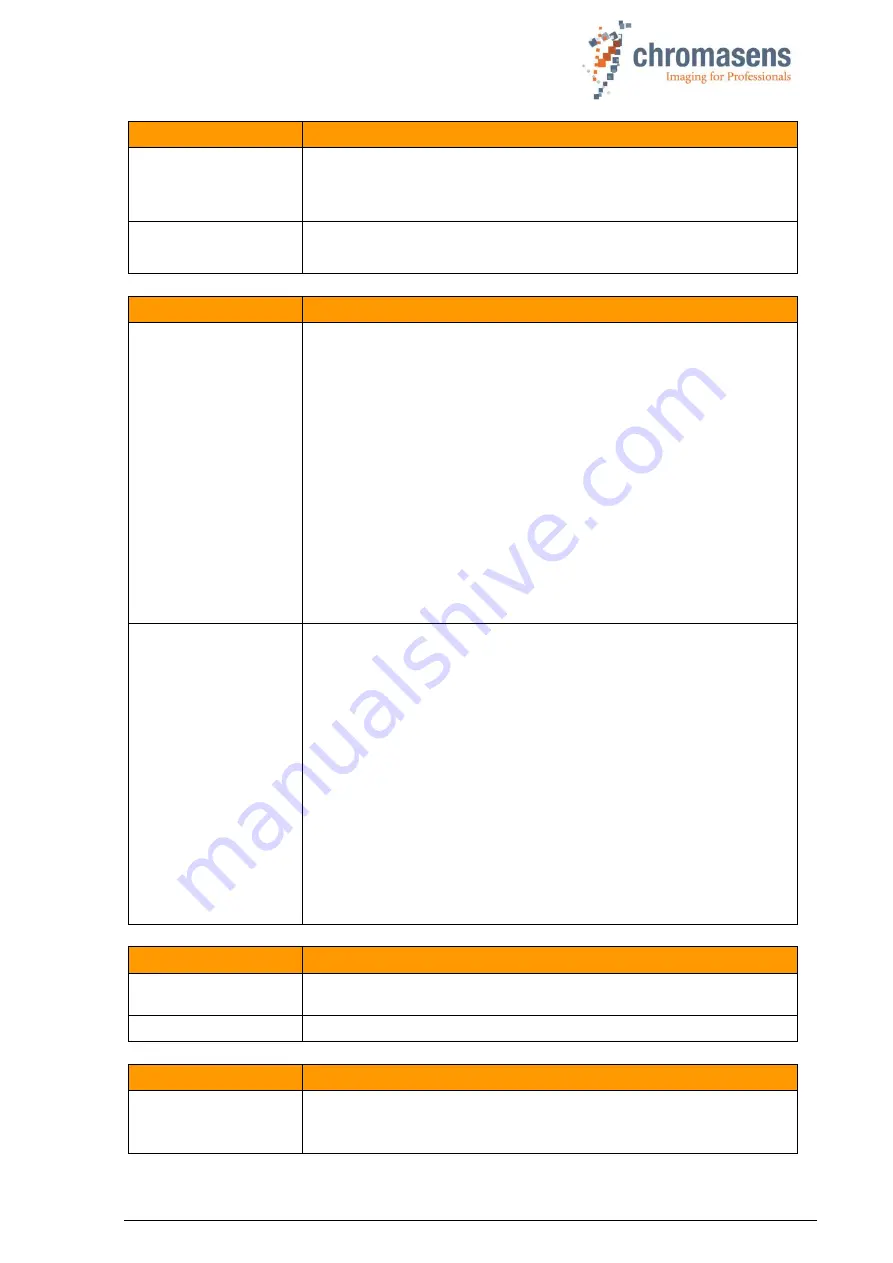
GenICam_SDK.docx
31
csiLogLevel
Defines the severity of log messages coming from the SDK
Definition
typedef enum csiLogLevel {
CSI_LOGLEVEL_ERROR = 1,
CSI_LOGLEVEL_WARN = 2,
CSI_LOGLEVEL_INFO = 4,
CSI_LOGLEVEL_DEBUG = 8,
CSI_LOGLEVEL_TRACE = 16,
} csiLogLevel;
Elements
CSI_LOGLEVEL_ERROR
CSI_LOGLEVEL_WARN
CSI_LOGLEVEL_INFO
CSI_LOGLEVEL_DEBUG
CSI_LOGLEVEL_TRACE
csiErr
Defines possible error values
Definition
typedef enum csiErr {
csiSuccess = 0,
csiNotInitialized = -100,
csiInvalidState = -101,
csiNotOpened = -102,
csiNoImageDataAvailable = -103,
csiNotFound = -104,
csiInvalidParameter = -105,
csiNotAvailable = -106,
csiFunctionNotAvailable = -107,
csiTimeout = -108,
csiAborted = -109,
csiFileOperationFailure = -110,
csiFileOperationFatalError = -111,
csiNoAccess = -112,
csiWrongBufferSize = -113,
csiInvalidBuffer = -114,
csiResourceInUse = -115,
csiNotImplemented = -116,
csiInvalidHandle = -117,
csiIOError = -118,
csiParsingError = -119,
csiInvalidValue = -120,
csiResourceExhausted = -121,
csiOutOfMemory = -122,
csiBusy = -123,
csiUnknown = -200,
csiCustomErr = 0x0f0000000
} csiErr;
Elements
csiSuccess
No error
csiNotInitialized
System is not initialized, call
csiInit()
first
csiInvalidState
An invalid state occurred, see log output for more information
csiNotOpened
There was an action that requires the device / network / stream to be opened
csiNoImageDataAvailable
There was no image data available
csiNotFound
General error that the requested information was not found, see log for more
detailed info on this error.
csiInvalidParameter
A parameter had an invalid value
csiNotAvailable
An expected result or a resource was not available
csiFunctionNotAvailable
The called function or a sub-function is not available
csiTimeout
A timeout occurred
csiAborted
A pending operation was aborted
csiFileOperationFailure
There was an error during file operation, see log for more information
csiFileOperationFatalError
There was a fatal error during file operation, see log for more information
csiNoAccess
Access denied (e.g., when trying to write a read only feature)
csiWrongBufferSize
A given buffer was too small to store the requested data
csiInvalidBuffer
The requested buffer is not valid
csiResourceInUse
The requested resource is already in use by the transport layer
csiNotImplemented
A function that was called is not yet implemented
csiInvalidHandle
A handle passed as parameter is not valid
csiIOError
The was an error during an IO operation (e.g. file or network)
csiParsingError
An error occurred when parsing an XML node map file
csiInvalidValue
A value that was passed parameter is not valid
csiResourceExhausted
A requested resource is exhausted (e.g. hard disk space)
csiOutOfMemory
Memory allocation failed, there is no more memory available
csiBusy
The requested operation cannot be executed because the system is busy
csiUnknown
Generic error, see log for more information
csiCustomErr = 0x0f0000000
Custom error codes defined by specific transport layers
csiAcquisitionMode
Defines acquisition mode
Definition
typedef enum csiAcquisitionMode {
CSI_ACQUISITION_SINGLE_FRAME = 0x00000001,
CSI_ACQUISITION_CONTINUOUS = 0xFFFFFFFF
} csiAcquisitionMode;
Elements
CSI_ACQUISITION_SINGLE_FRAME Acquire a single frame only
CSI_ACQUISITION_CONTINUOUS
Perform continuous frame acquisition
csiEventType
Defines event types that the user application can listen for
Definition
typedef enum csiEventType {
CSI_EVT_NEWIMAGEDATA = 0x00,
CSI_EVT_ERROR = 0x01,
CSI_EVT_MODULE = 0x02,
CSI_EVT_CUSTOM = 0x1000
} csiEventType;