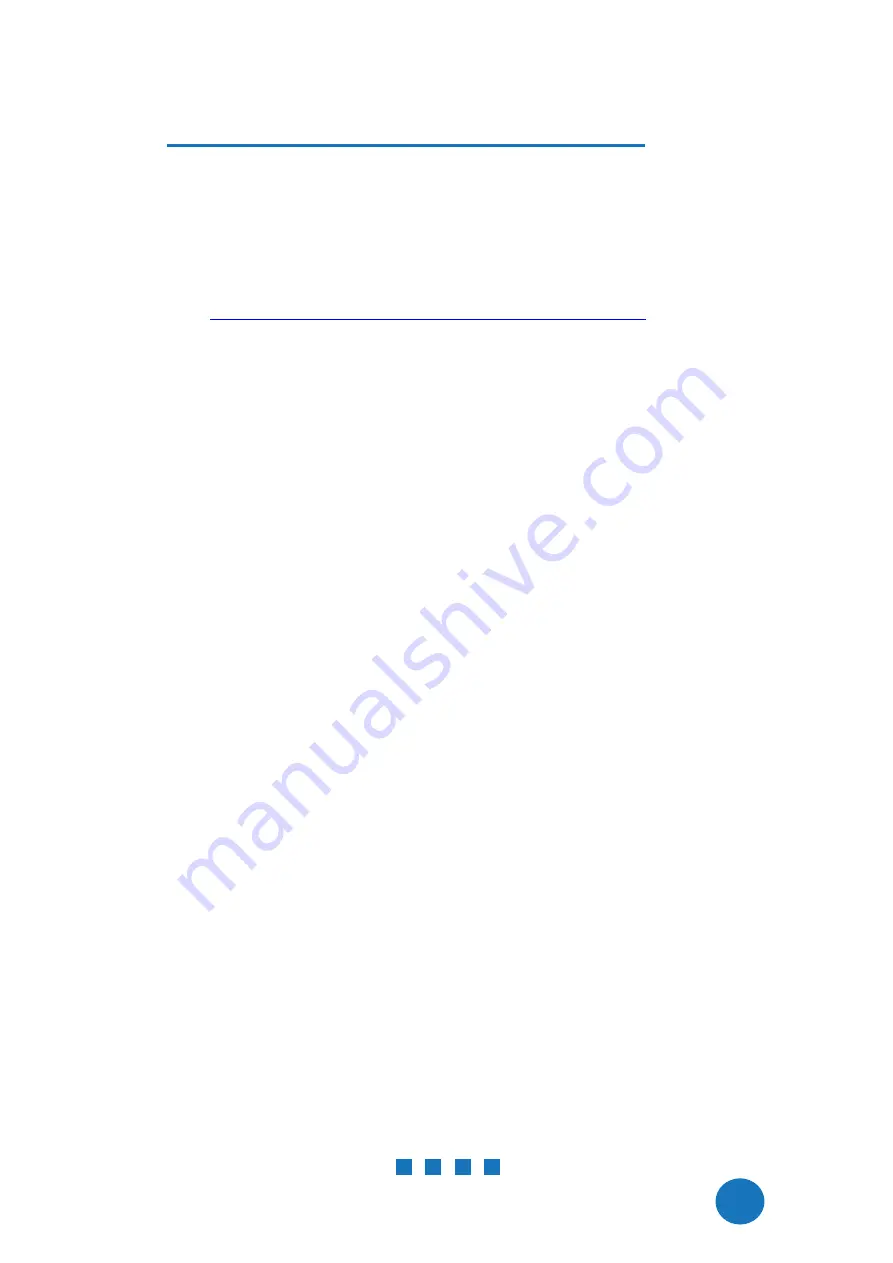
DroneScout Receiver Manual - version 1.0 May 2022 - © BlueMark Innovations BV 2022
22/28
4 MQTT
SUBSCRIBER
(
REFERENCE CODE
)
You need to write your own MQTT subscriber application to process the MQTT messages published
by the DroneScout receivers.
Below reference Python3 source code is published to process such MQTT messages. It can also be
found here:
https://github.com/BluemarkInnovations/RemoteID-MQTT-subscriber
#!/usr/bin/python3
# (c) Bluemark Innovations BV
# MIT license
import random
import ssl
import json
import base64
from paho.mqtt import client as mqtt_client
from pymavlink import mavutil
from bitstruct import *
import lzma
import datetime
broker = 'myserver'
port = 8883
topic = "#"
# generate client ID with pub prefix randomly
client_id = f'mqtt-subscriber-{random.randint(0, 100)}'
#optional user/password for connecting to the MQTT broker, uncomment if used.
#username = ''
#password = ''
#file containing full SSL chain, uncomment when MQTT broker uses encrypted messages
[preferred]
client_pem = "./certs/client.pem"
def connect_mqtt() -> mqtt_client:
def on_connect(client, userdata, flags, rc):
if rc == 0:
print("Connected to MQTT Broker!")
else:
print("Failed to connect, return code %d\n", rc)
client = mqtt_client.Client(client_id)
#if username and password is set
if 'username' in globals():
print("username/password enabled")
client.username_pw_set(username, password)
#if ssl is enabled
if 'client_pem' in globals():
print("ssl enabled")
client.tls_set(client_pem, tls_version=ssl.PROTOCOL_TLSv1_2)
client.tls_insecure_set(True)