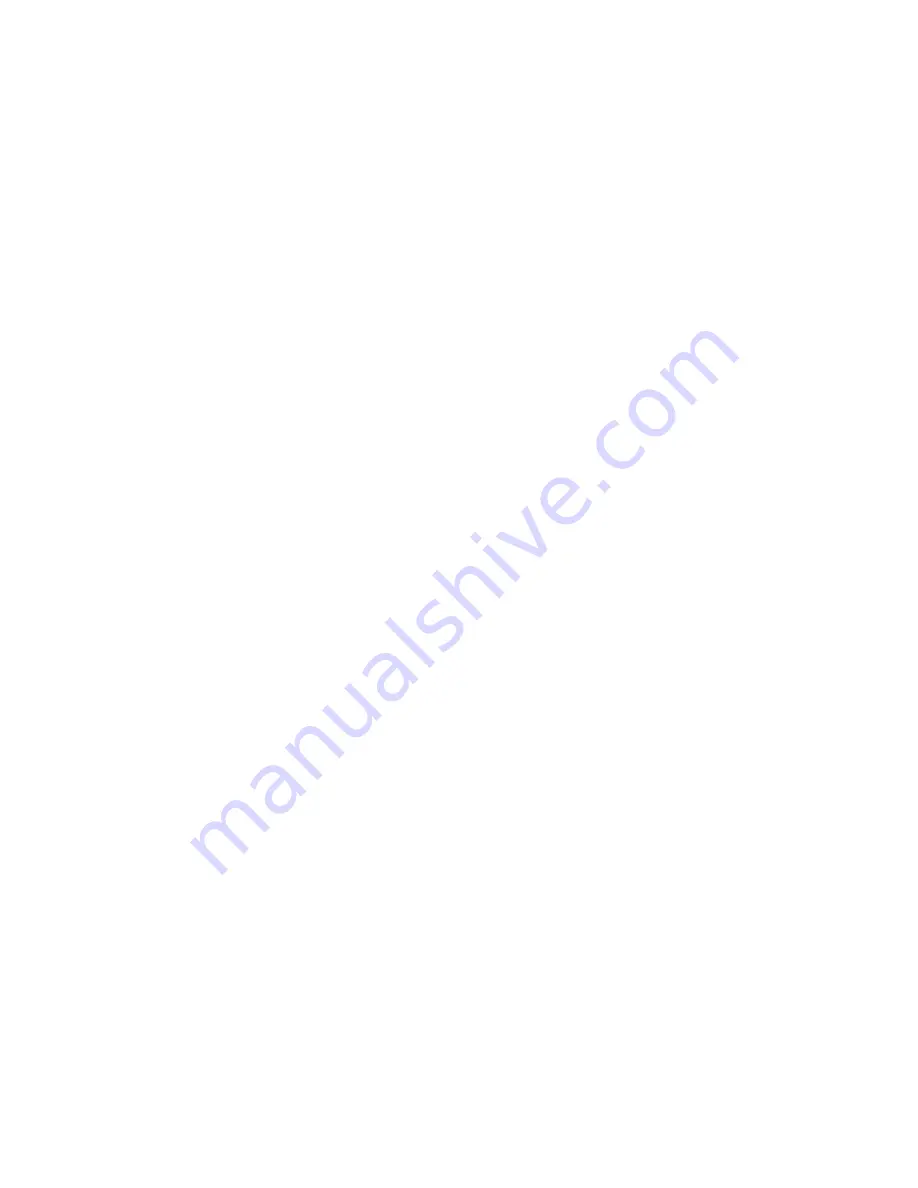
97
Visual Basic Scripting
To access an RPC using Visual Basic, first create a WACI object of type “WACI.UserAPI.1”. Then, call
the method with the object, specifying any parameters in parentheses. For example, this script in an
Active Server Page retrieves the IP address of the WACI and the settings for serial ports 1 and 2.
Dim waciUser
Dim strIP, strErr
Dim strPort1, strPort2
set waciUser = CreateObject(“WACI.UserAPI.1”)
strIP = waciUser.Net_GetIPAddress
if(strIP = ““) then
strErr = waciUser.GetLastErrorString
end if
strPort1 = waciUser.Serial_GetSettings(1)
strPort2 = waciUser.Serial_GetSettings(2)
Some notes:
•
Parameters and returned values are strings, integers, or Booleans, depending on the method.
•
The above example includes error checking for Net_GetIPAddress. Methods will report
errors based on the type of its return value. Strings will return the null string, integers will
return -1, and Booleans will return FALSE.
•
See the detailed list of methods at the end of this reference for details about the parameters
and return values for any given method. Parameter values are denoted by the text “
[in]
” in
the Syntax and Parameters sections. Similarly, the return value is denoted by the text
“
[out, retval]
”.
•
String values should be passed in as URL encoded values. Returned strings are also URL
encoded.
Note on Error Checking
The HRESULT, referred to in the Syntax of each method, ALWAYS returns “S_OK”, so it is not useful
in error checking.
Instead, use the error checking values described in the HTTP Post and Visual Basic above, and the Error
Information Methods below (see
Error Information Methods
, page
100
) to perform meaningful error
checks.
Errors are based on the type of a function’s
out/retval
value. On error, a string
retval
is returned
as a NULL string, an integer
retval
returns -1, and Booleans will return FALSE.