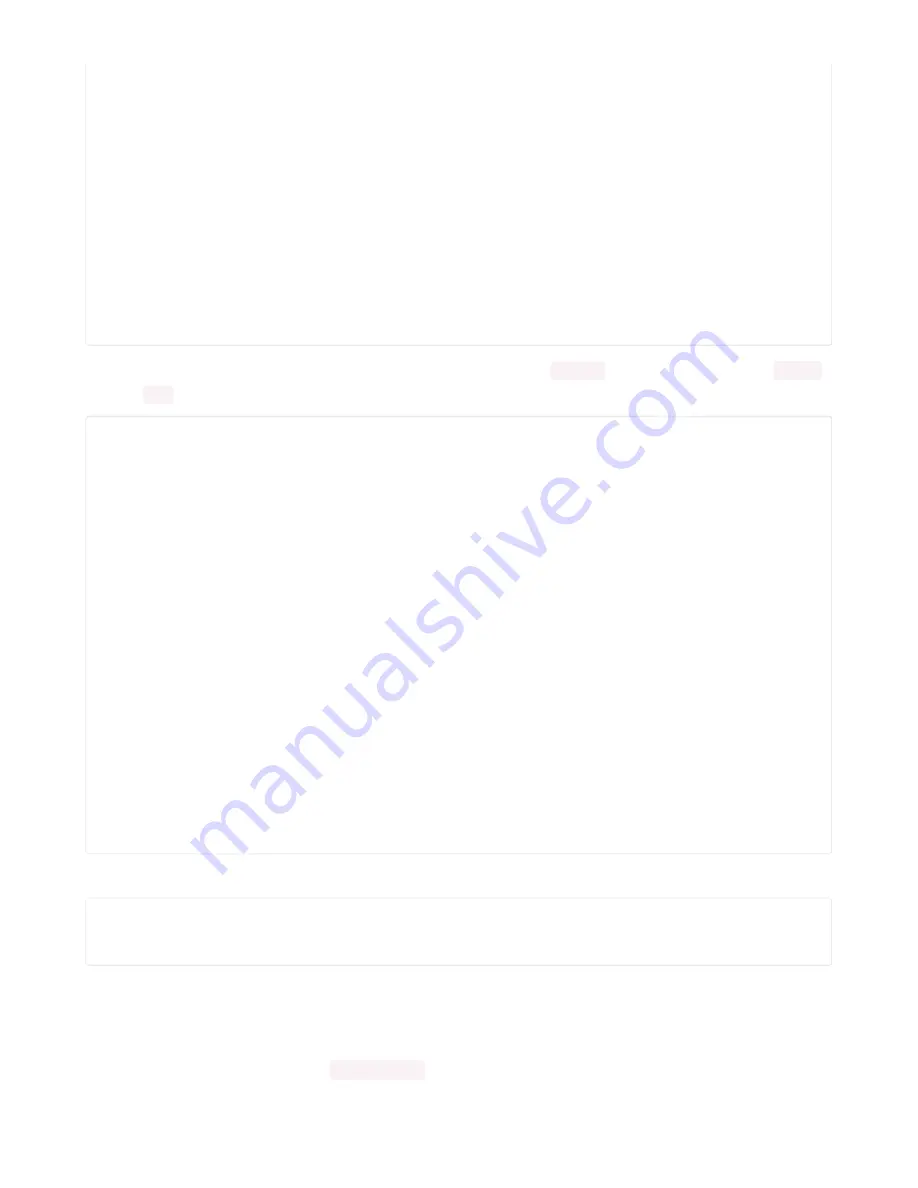
json_resp = response.json()
# Parse out the 'data' key from json_resp dict.
print("Data received from server:", json_resp["data"])
print("-" * 40)
response.close()
json_data = {"Date": "July 25, 2019"}
print("POSTing data to {0}: {1}".format(JSON_POST_URL, json_data))
response = requests.post(JSON_POST_URL, json=json_data)
print("-" * 40)
json_resp = response.json()
# Parse out the 'json' key from json_resp dict.
print("JSON Data received from server:", json_resp["json"])
print("-" * 40)
response.close()
The code first sets up the ESP32SPI interface. Then, it initializes a
request
object using an ESP32
socket
and the
esp
object.
import board
import busio
from digitalio import DigitalInOut
import adafruit_esp32spi.adafruit_esp32spi_socket as socket
from adafruit_esp32spi import adafruit_esp32spi
import adafruit_requests as requests
# If you have an externally connected ESP32:
esp32_cs = DigitalInOut(board.D9)
esp32_ready = DigitalInOut(board.D10)
esp32_reset = DigitalInOut(board.D5)
spi = busio.SPI(board.SCK, board.MOSI, board.MISO)
esp = adafruit_esp32spi.ESP_SPIcontrol(spi, esp32_cs, esp32_ready, esp32_reset)
print("Connecting to AP...")
while not esp.is_connected:
try:
esp.connect_AP(b'MY_SSID_NAME', b'MY_SSID_PASSWORD')
except RuntimeError as e:
print("could not connect to AP, retrying: ",e)
continue
print("Connected to", str(esp.ssid, 'utf-8'), "\tRSSI:", esp.rssi)
# Initialize a requests object with a socket and esp32spi interface
requests.set_socket(socket, esp)
Make sure to set the ESP32 pinout to match your AirLift breakout's connection:
esp32_cs = DigitalInOut(board.D9)
esp32_ready = DigitalInOut(board.D10)
esp32_reset = DigitalInOut(board.D5)
HTTP GET with Requests
The code makes a HTTP GET request to Adafruit's WiFi testing website
http://wifitest.adafruit.com/testwifi/index.html
(https://adafru.it/FpZ)
.
To do this, we'll pass the URL into
requests.get()
. We're also going to save the response
from the server
© Adafruit Industries
https://learn.adafruit.com/adafruit-airlift-shield-esp32-wifi-co-processor
Page 30 of 56
Содержание AirLift Shield ESP32
Страница 20: ...Adafruit Industries https learn adafruit com adafruit airlift shield esp32 wifi co processor Page 20 of 56...
Страница 40: ...Adafruit Industries https learn adafruit com adafruit airlift shield esp32 wifi co processor Page 40 of 56...
Страница 56: ...Adafruit Industries Last Updated 2021 03 29 01 04 51 PM EDT Page 56 of 56...