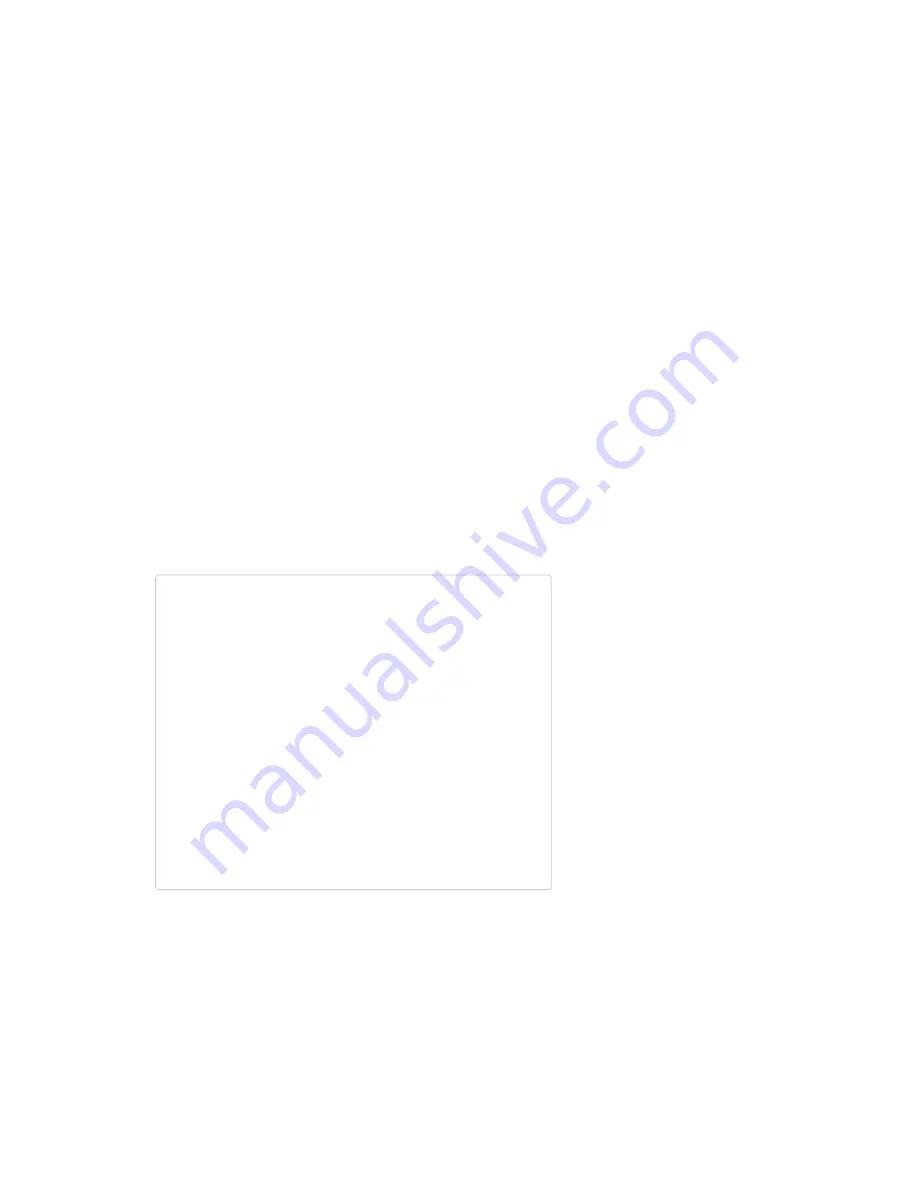
Checking for available message
An
sms.available(sms_status)
function is defined for you to check if any
read and/or unread messages have been received. The value returned by
this function may not be what you expect, though – it returns the
index of
the first requested message
. Every message stored in the module is
assigned an index number. The index is important because it identifies
which message you want to read later on.
sms.available()
expects one parameter, it can be any of:
•
sms.available(REC_UNREAD)
– Returns the index of the first unread
message.
•
sms.available(REC_READ)
– Returns the index of the first read
message.
•
sms.available(REC_ALL)
– Returns the index of the first read
or
unread message.
If
sms.available()
doesn’t find any of the requested message type, it will
return 0.
Reading an SMS
After calling
sms.available()
and finding a message index to read, use
sms.read(index)
to read it.
Aside from an error code (1 for success, negative number for an error),
sms.read()
doesn’t return the message. Instead use
sms.getMessage()
,
sms.getSender()
, and
sms.getDate()
to read the message, sending
phone, and timestamp the message was sent. These values are only
updated after an
sms.read()
.
Here’s an example based on the sketch:
// Get first available unread message:
int messageIndex = sms.available(REC_UNREAD);
// If an index was returned, proceed to read that message:
if (messageIndex > 0)
{
// Call sms.read(index) to read the message:
sms.read(messageIndex);
Serial.print(F("Reading message ID: "));
Serial.println(messageIndex);
// Print the sending phone number:
Serial.print(F("SMS From: "));
Serial.println(sms.getSender());
// Print the receive timestamp:
Serial.print(F("SMS Date: "));
Serial.println(sms.getDate());
// Print the contents of the message:
Serial.print(F("SMS Message: "));
Serial.println(sms.getMessage());
}
The strings in these “get” functions will remain intact until you call
sms.read()
on a different index.
Sending an SMS
There are at least three steps to sending an SMS message:
sms.start(phoneNumber)
,
sms.print(message)
, and
sms.send()
. All
three are required to send any message.
sms.start(phoneNumber)
indicates that you’re beginning to write a message and sets the destination
phone number.
sms.print()
can be used to send any data type – string,
integer, float, you name it in the body of the text. Just take care not to send
anything besides
sms.print()
between your
start()
and
send()
calls.
Page 14 of 22