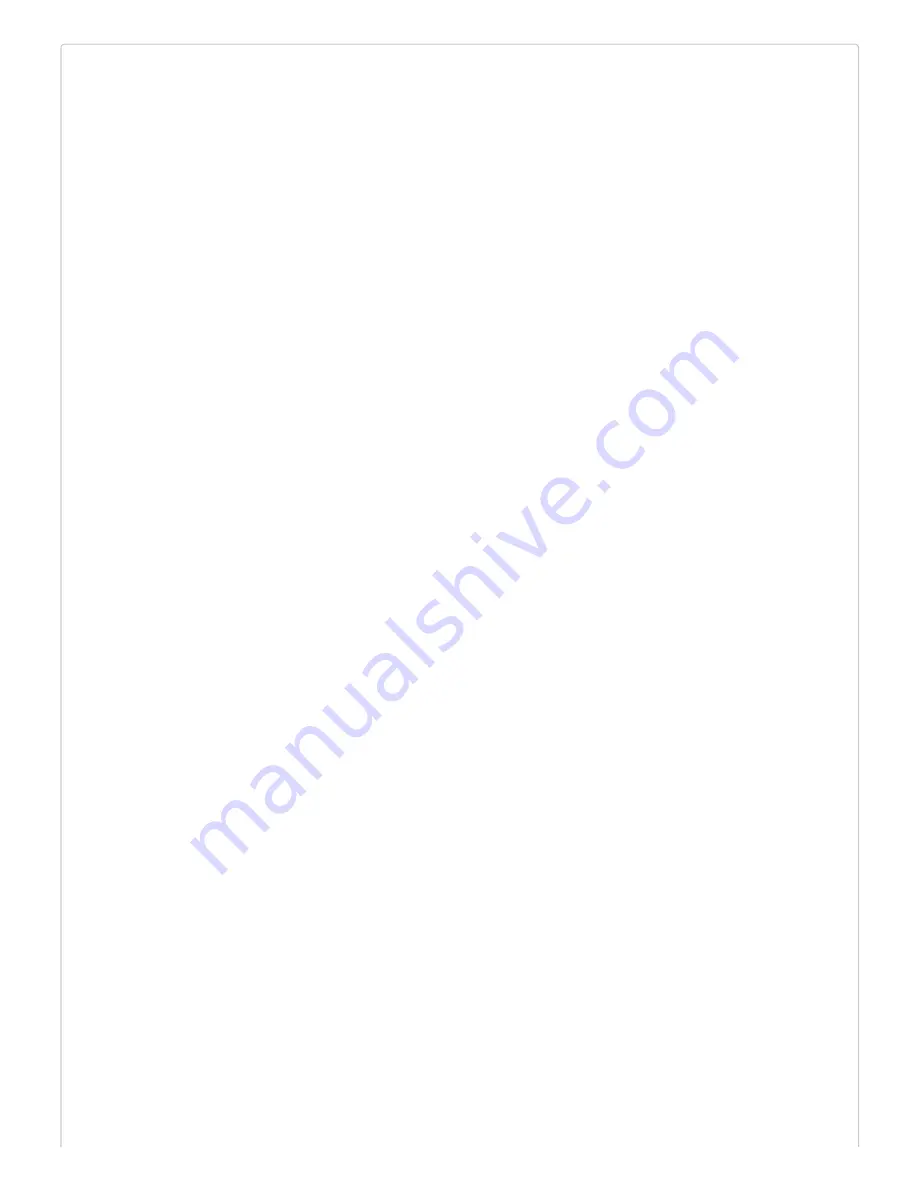
/******************************************************************************
Example_3b_Qwiic_Keypad_HID_Keyboard.ino
Written by: Ho Yun "Bobby" Chan
Date: February 6, 2020
Development Environment Specifics:
Arduino IDE 1.8.9
Description:
Based on the Jim's Pro Micro "HID Mouse" and Pete' Qwiic Keypad "read button"
examples, this example outputs keyboard presses associated with the keypad.
Libraries:
Keyboard.h (included with Arduino IDE)
Wire.h (included with Arduino IDE)
SparkFun_Qwiic_Keypad_Arduino_Library.h (included in the src folder) http://librarymanager/Al
l#SparkFun_keypad
License:
This code is released under the MIT License (http://opensource.org/licenses/MIT)
******************************************************************************/
#include <Keyboard.h>
#include <Wire.h>
#include "SparkFun_Qwiic_Keypad_Arduino_Library.h" //Click here to get the library: http://libra
rymanager/All#SparkFun_keypad
KEYPAD keypad1; //Create instance of this object
void setup() {
Serial.begin(9600);
Serial.println("Qwiic KeyPad Example");
if (keypad1.begin() == false) // Note, using begin() like this will use default I2C address,
0x4B.
// You can pass begin() a different address like so: keypad1.begin(Wire, 0x4A).
{
Serial.println("Keypad does not appear to be connected. Please check wiring. Freezing...");
while (1);
}
Serial.print("Initialized. Firmware Version: ");
Serial.println(keypad1.getVersion());
Serial.println("Press a button: * to do a space. # to go to next line.");
Keyboard.begin(); //Init keyboard emulation
}
void loop() {
keypad1.updateFIFO(); // necessary for keypad to pull button from stack to readable register
char button = keypad1.getButton();
if (button == -1)
{
Serial.println("No keypad detected");