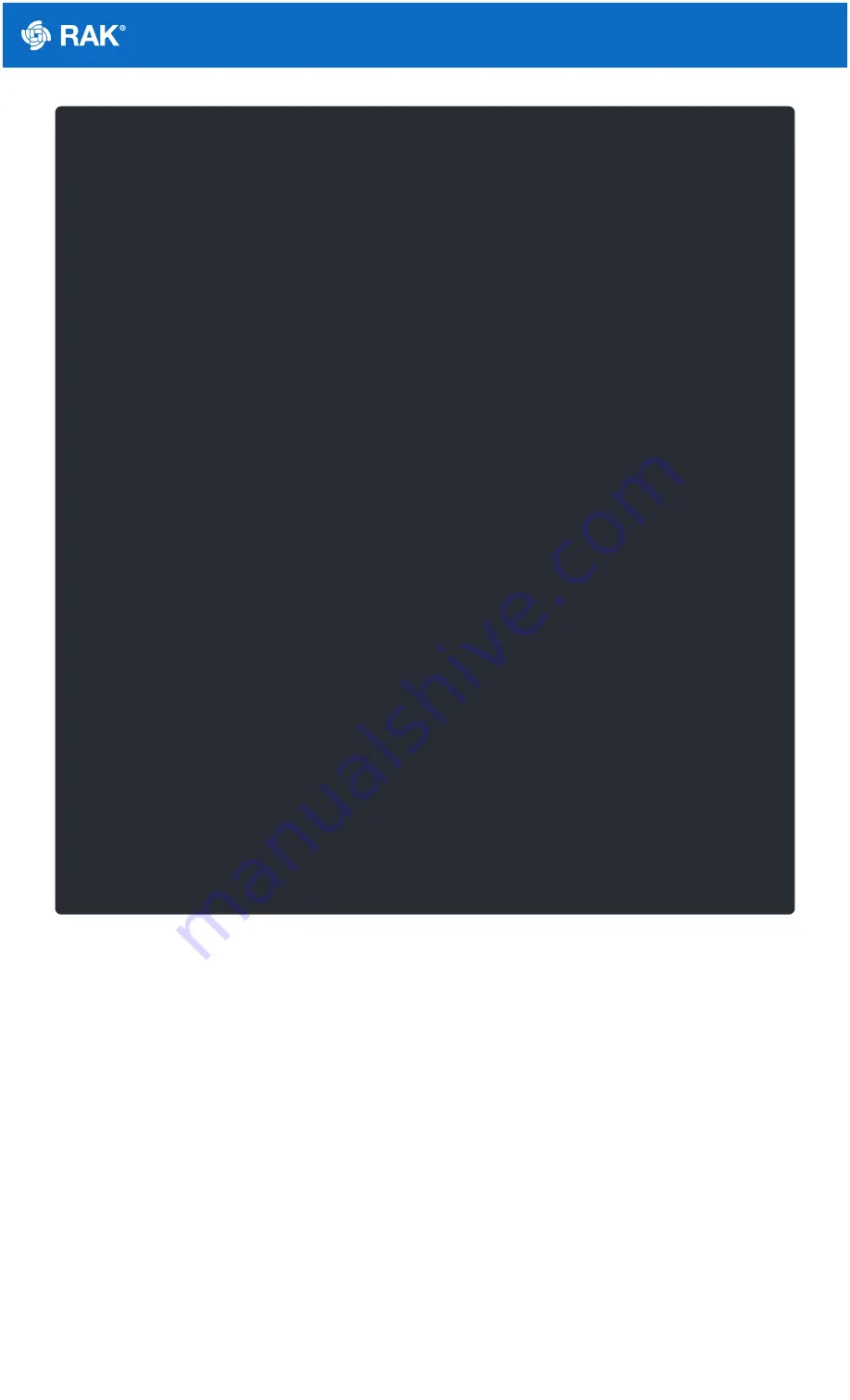
Documentation Center
3. Then select the right Serial Port and upload the code, as shown in Figure 19 and Figure 20.
/*
* Reading Long Press and Short Press on a Button using RAK13002
*
*/
// constants won't change. They're used here to set pin numbers:
const
int
BUTTON_PIN
=
WB_IO1
;
// the number of the pushbutton pin
const
int
SHORT_PRESS_TIME
=
500
;
// 500 milliseconds
// Variables will change:
int
lastState
=
LOW
;
// the previous state from the input pin
int
currentState
;
// the current reading from the input pin
unsigned
long
pressedTime
=
0
;
unsigned
long
releasedTime
=
0
;
void
setup
()
{
Serial
.
begin
(
9600
);
pinMode
(
BUTTON_PIN
,
INPUT_PULLUP
);
}
void
loop
()
{
// read the state of the switch/button:
currentState
=
digitalRead
(
BUTTON_PIN
);
if
(
lastState
==
HIGH
&&
currentState
==
LOW
)
// button is pressed
pressedTime
=
millis
();
else
if
(
lastState
==
LOW
&&
currentState
==
HIGH
)
{
// button is released
releasedTime
=
millis
();
long
pressDuration
=
releasedTime
-
pressedTime
;
if
(
pressDuration
<
SHORT_PRESS_TIME
)
Serial
.
println
(
"A short press is detected"
);
}
// save the the last state
lastState
=
currentState
;
}
c