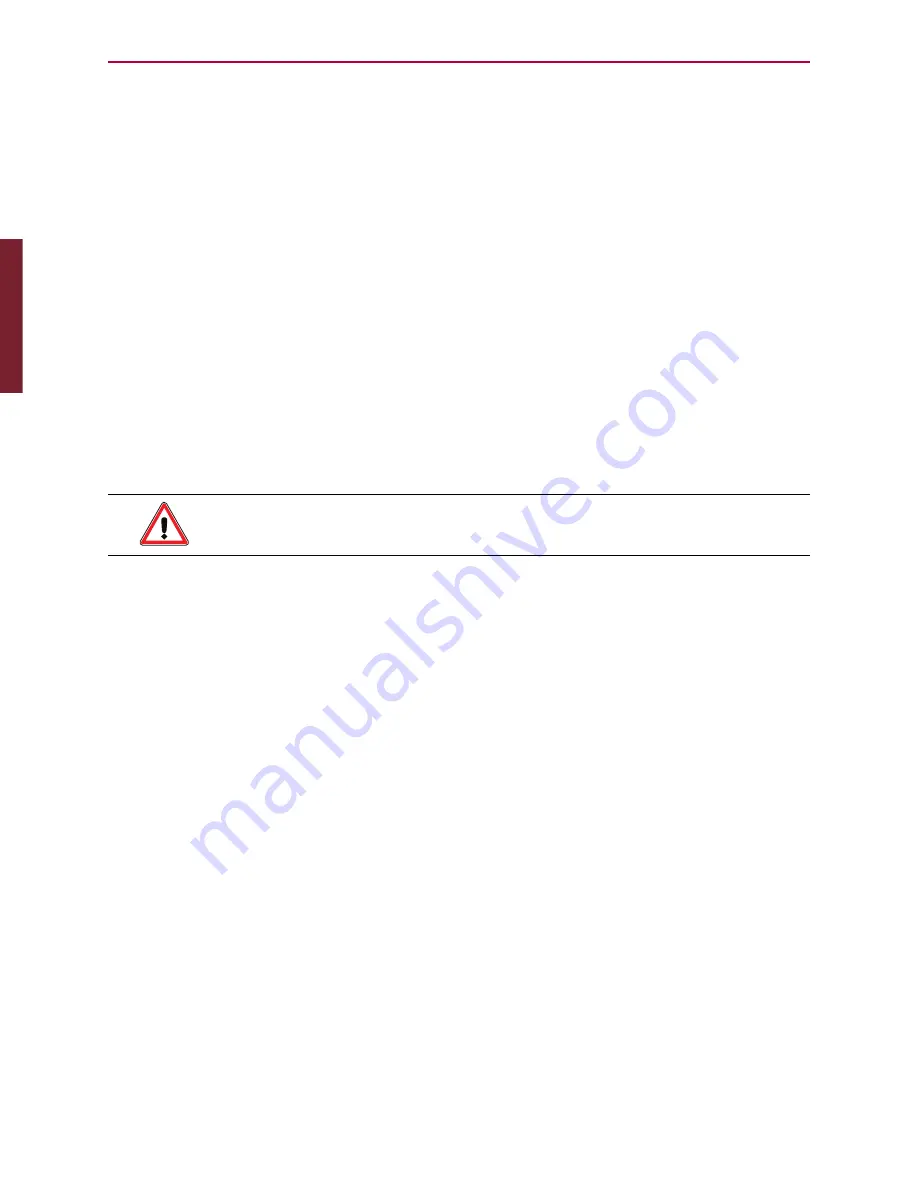
P
ar
t
1: P
rog
ra
m
ming
Moog Animatics SmartMotor™ Developer's Guide, Rev. L
Page 184 of 909
C10 label near the beginning of the program but after the initialization code, and then place a
GOTO10 at the end. Then, every time the GOTO10 is reached, the program will loop back to
label C10 and start over from that point until the GOTO10 is reached again, which will start the
process at C10 again, and so on. This will make the program run continuously.
Any program can be written with only one GOTO. It might be a little harder, but it will force
better program organization. If you organize your program using the GOSUB command
instead of multiple GOTO commands, it will be much easier to read and support.
GOSUB#, GOSUB(label), RETURN
Execute a Subroutine and Return
Just like the GOTO# command, the GOSUB# command, used in conjunction with a C# label,
redirects program execution to the location of the label. However, unlike the GOTO#
command, the C# label needs to eventually be followed by a RETURN command. This returns
the program execution to the location of the original GOSUB# command that initiated the
redirection.
There may be many sections of a program that need to perform the same basic group of
commands. By encapsulating these commands between a C# label and a RETURN, they
become a subroutine that can be called any time from anywhere with a GOSUB# or GOSUB
(label), rather than being repeated. There can be as many as one thousand (0 - 999) different
subroutines, and they can be accessed as many times as the application requires.
CAUTION:
Calling subroutines from the host can crash the stack if not done
carefully.
By pulling sections of code out of a main loop and encapsulating them into subroutines, the
main code can also be easier to read. Therefore, organizing code into multiple subroutines is a
good practice.
C10
'Place label
IF
IN
(0)==0
'Check Input 0
GOSUB20
'If Input 0 low, call Subroutine 20
ENDIF
'End check Input 0
IF
IN
(1)==0
'Check Input 1
a=30
'as example for below
GOSUB
(a)
'If Input 1 low, call Subroutine 30
ENDIF
'End check Input 1
GOTO
(10)
'Will loop back to C10
IF, ENDIF
Conditional Test
When the execution of the code reaches the IF command, the code between that IF and the
following ENDIF executes only when the condition directly following the IF command is true.
For example:
a=IN(0)
'Variable "a" set 0,1
a=a+IN(1)
'Variable "a" 0,1,2
IF
a==1
'Use double "=" to test
b=1
'Set "b" to one
ENDIF
'End IF
Part 1: Programming: GOSUB#, GOSUB(label), RETURN