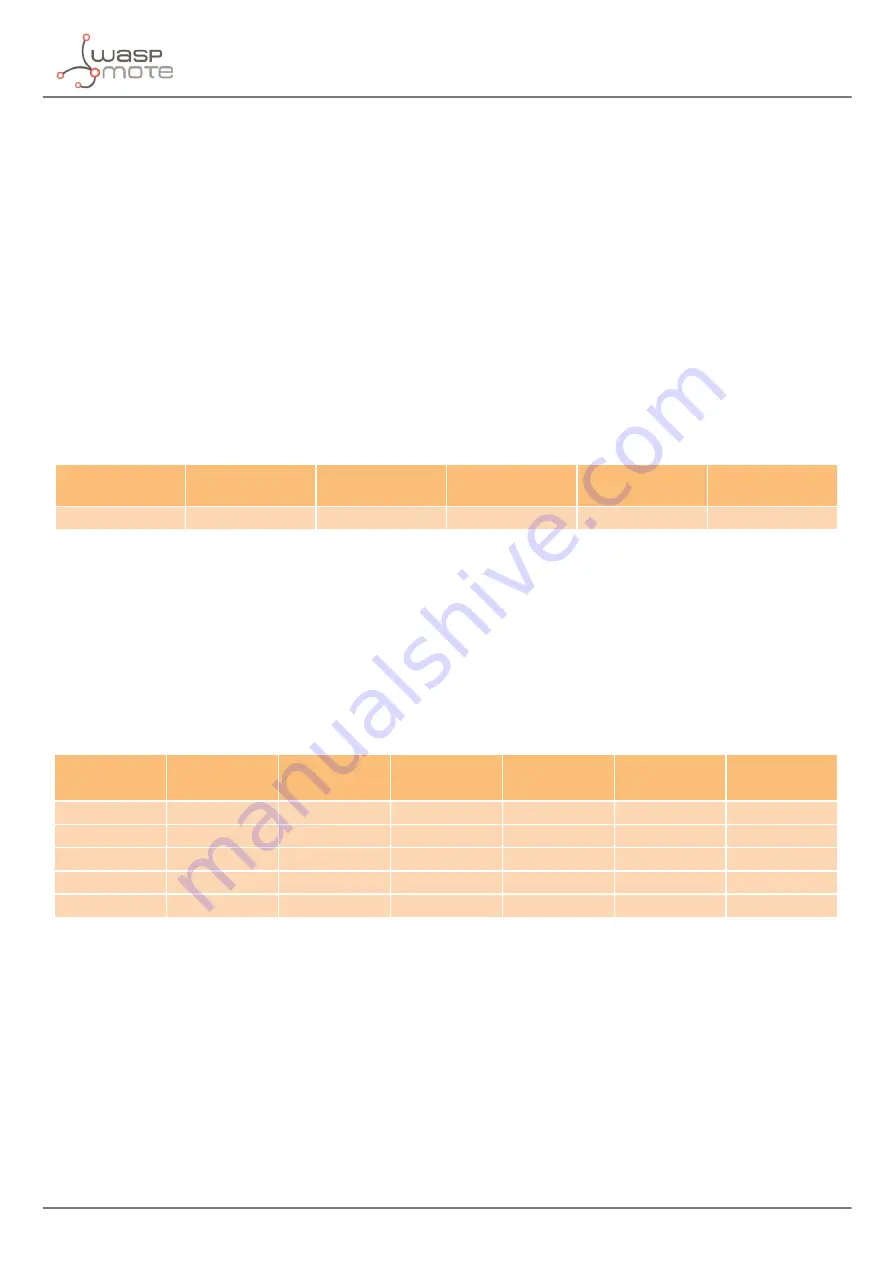
-7-
v7.0
Integrity
2.2. Calculating Message Digest
This section will describe how calculate a message digest depends on the selected algorithm. The different algorithms provide
different messages digest size.
For example, the Message Digest (MD5) hash is a mathematical algorithm which produces a unique 128 bit number (a hash)
created from the data input. If even one bit of data changes, the hash value will change.
2.2.1. Calculating MD5 hash
Previously, a variable to store the message digest must be declared. This variable must be correctly dimensioned to contain the
calculated hash message.
{
uint8_t hash_message[16];
}
The features that must be met in order to calculate the MD5 algorithm are:
Algorithms
Output size
(bits)
Internal state
size (bits)
Block size
(bits)
Max message
size (bits)
Word size
(bits)
MD5
128
128
512
2⁶⁴− 1
32
The next code shows how to calculate the message digest with
HASH.md5()
. The inputs expected are: the pointer to the buffer
where the output is stored, the input message pointer and the length of the input message.
{
char message[] = “Libelium”;
HASH.md5(hash_message_md5, (uint8_t*)message, strlen(message)*8);
}
2.2.2. Calculating SHA hash
The features that must be met in order to calculate the SHA algorithm are:
Algorithms
Output size
(bits)
Internal state
size (bits)
Block size
(bits)
Max message
size (bits)
Word size
(bits)
Rounds
SHA-1
160
160
512
2⁶⁴− 1
32
80
SHA-224
224
256
512
2⁶⁴− 1
32
64
SHA-256
256
256
512
2⁶⁴− 1
32
64
SHA-384
384
512
512
2¹²⁸− 1
64
80
SHA-512
512
512
1024
2¹²⁸− 1
64
80
Previously, a variable to store the message digest must be declared. This variable must be correctly dimensioned to contain the
calculated hash message.
If SHA-1 is used, the variable that stores the hash message is defined:
{
uint8_t hash_message[20];
}
If SHA-384 is used, the variable that stores the hash message is defined:
{
uint8_t hash_message[48];
}