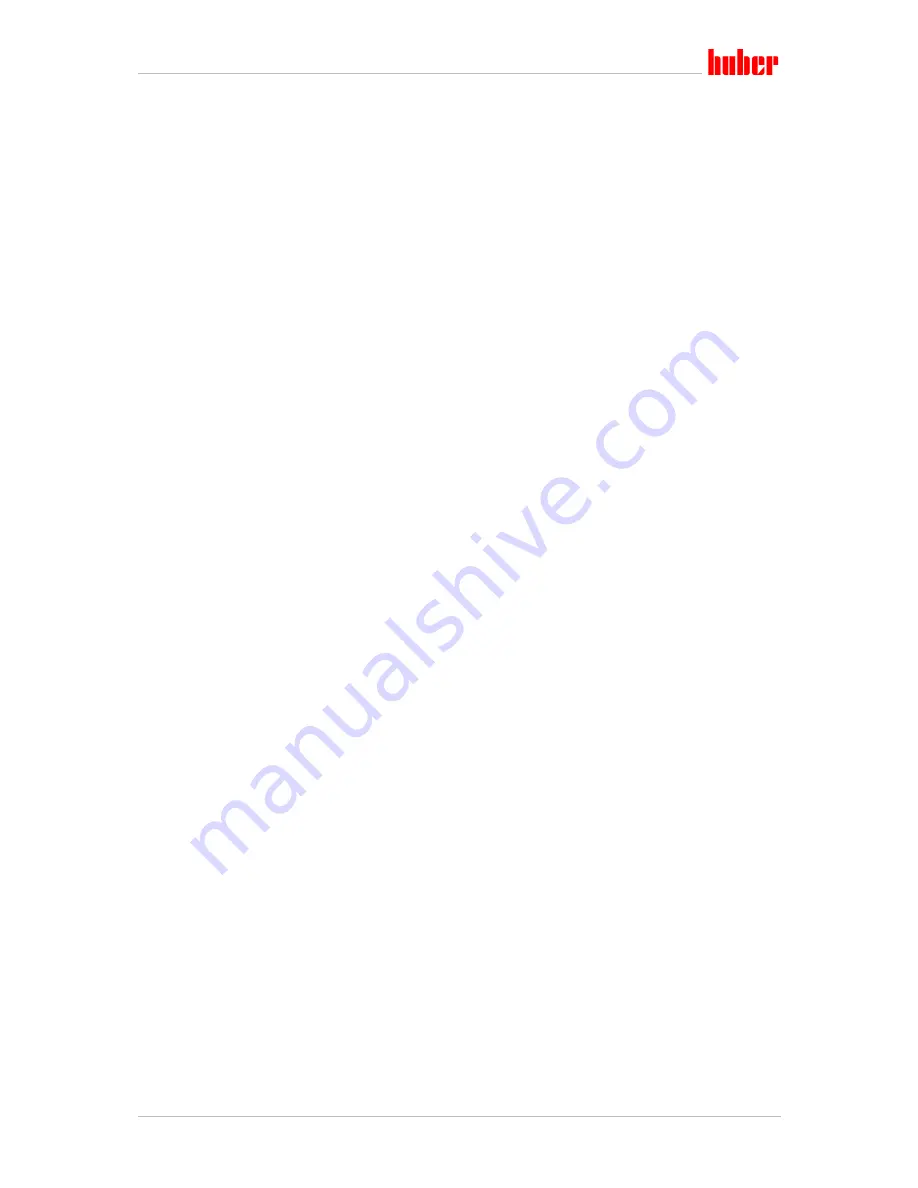
11
OPERATION MANUAL
4
Examples
The following example constantly queries the internal temperature and outputs the response via the
serial interface or checks the response in various ways.
4.1 Example 1
Program
1 from softcheck.logic import Com
2 import time
3
4 def script():
5
6 com = Com("serial", 2.5) #timeout should be specified in the constructor
7 com.open(0, 9600) #open COM1 with 9600 (address not defined)
8 # com.open("/dev/ttyACM0", 9600) #open usb port
9 # com.open("192.168.0.126", 8101) #open ethernet
10 com.set_timeout(2.3)
11
12
13 while 1:
14
15 print("")
16 print("Ask for the internal temperature and print out the answer.")
17 com.send("{M01****\r\n")
18 com.print_answer()
19
20
21 print("")
22 print("Ask for the internal temperature and check the first four chars.")
23 print("Output is only generated in case of an error.")
24 com.send("{M01****\r\n")
25 com.check("{S01")
26
27 print("")
28 print("Ask for the internal temperature and check the answer with a
regex.")
29 print("Output is only generated in case of an error.")
30 com.send("{M01****\r\n")
31 com.check_regex(r"{S01[0-9a-fA-F]*")
32
33 print("")
34 print("Ask for the internal temperature and print out a
formatted string as answer")
35 com.send("{M01****\r\n")
36 answer = com.recv()
37 answer = answer[:-2]
38 print("received answer: " + answer)
39
40 time.sleep(5)
41 com.close()
42
43 script()
Program description
The most important lines of the sample program are described below.
1: Includes the library described into the script. This call must occur at the beginning of each script.
pySoftcheck.01.20171002.2
Liability for errors and misprints excluded.
pySoftcheck
Summary of Contents for Pilot ONE
Page 1: ...Operation Manual ...
Page 2: ......