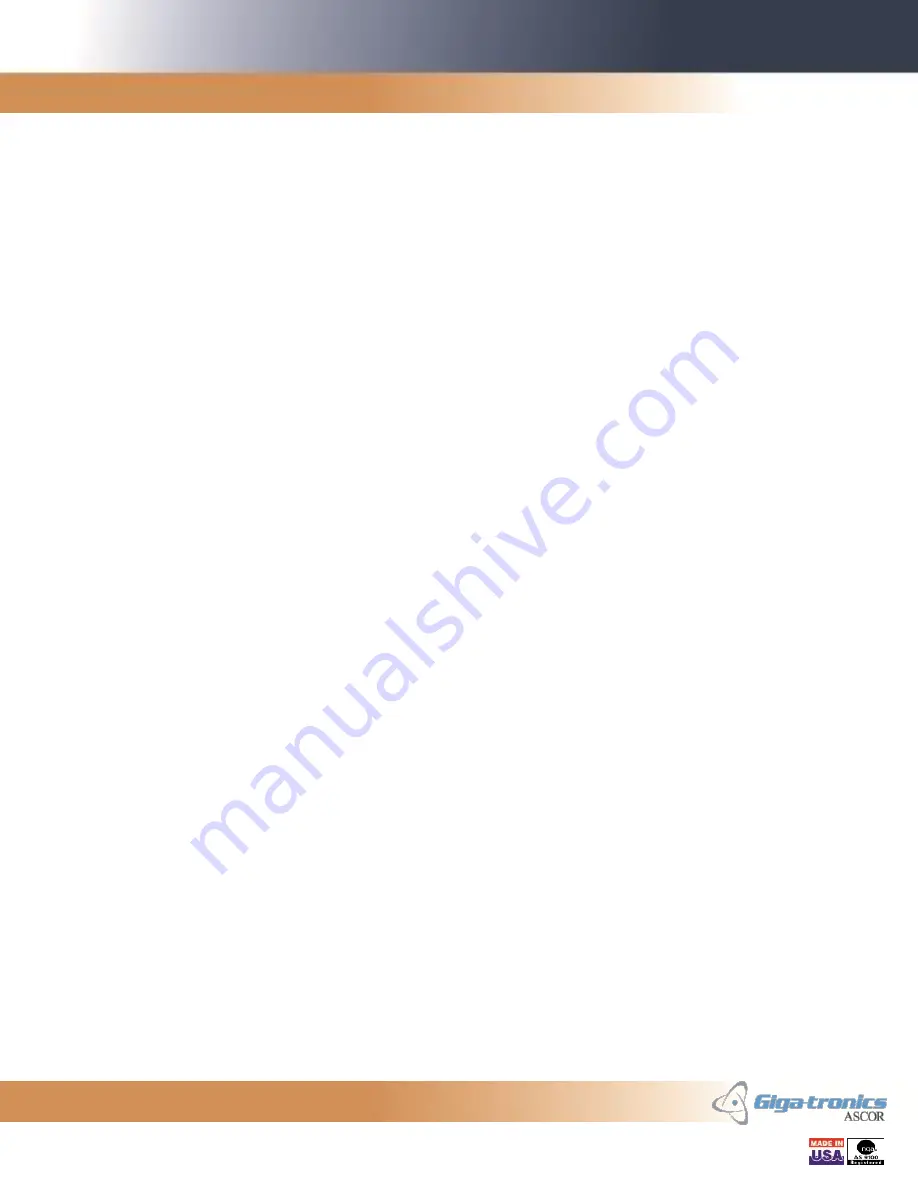
Giga-tronics ASCOR SERIES 8000 Getting Started Guide Rev. C
Getting Started Guide, Part Number 07507593, Rev C, August 14, 2013
38 of 38
// series8000.cpp : Sample console program for controlling Series 8000 Switch.
//
#include "stdafx.h"
#include "string.h"
#include "visa.h"
int _tmain(int argc, _TCHAR* argv[])
{
ViChar
resourceName[255], cmdBuf[255], rspBuf[255];
ViSession
rmSession, instrSession;
ViStatus
status;
ViUInt32
retCnt;
// Open Resource Manager Session
status = viOpenDefaultRM (&rmSession);
if (status < VI_SUCCESS) {
return status;
}
// Open Instrument Session for Series 8000 Switch at IP address 192.168.0.254
strcpy_s (resourceName, "TCPIP0::192.168.0.254::inst0::INSTR");
status = viOpen (rmSession, resourceName, VI_NULL, VI_NULL, &instrSession);
if (status != VI_SUCCESS) {
instrSession = 0;
return status;
}
// Send the command to open switch 1
strcpy_s (cmdBuf, "ROUTe:SWITch 1, 0");
status = viWrite (instrSession, (ViBuf)cmdBuf, (ViUInt32) strlen (cmdBuf), &retCnt);
if (status != VI_SUCCESS) {
return status;
}
// Send the command to close switch 1 position 1
strcpy_s (cmdBuf, "ROUTe:SWITch 1, 1");
status = viWrite (instrSession, (ViBuf)cmdBuf, (ViUInt32) strlen (cmdBuf), &retCnt);
if (status != VI_SUCCESS) {
return status;
}
// Send the command to query switch 1 position
strcpy_s (cmdBuf, "ROUTe:SWITch? 1");
status = viWrite (instrSession, (ViBuf)cmdBuf, (ViUInt32) strlen (cmdBuf), &retCnt);
if (status != VI_SUCCESS) {
return status;
}
// Read the response to the query
status = viRead (instrSession, (ViBuf)rspBuf, 100, &retCnt);
if (status != VI_SUCCESS) {
return status;
}
// Close the Instrument Session for the Series 8000 Switch
status = viClose (instrSession);
if (status != VI_SUCCESS) {
return status;
}
// Close the Resource Manager Session
status = viClose (rmSession);
if (status != VI_SUCCESS) {
return status;
}
return 0;
}
Figure 24: C++ Sample Program