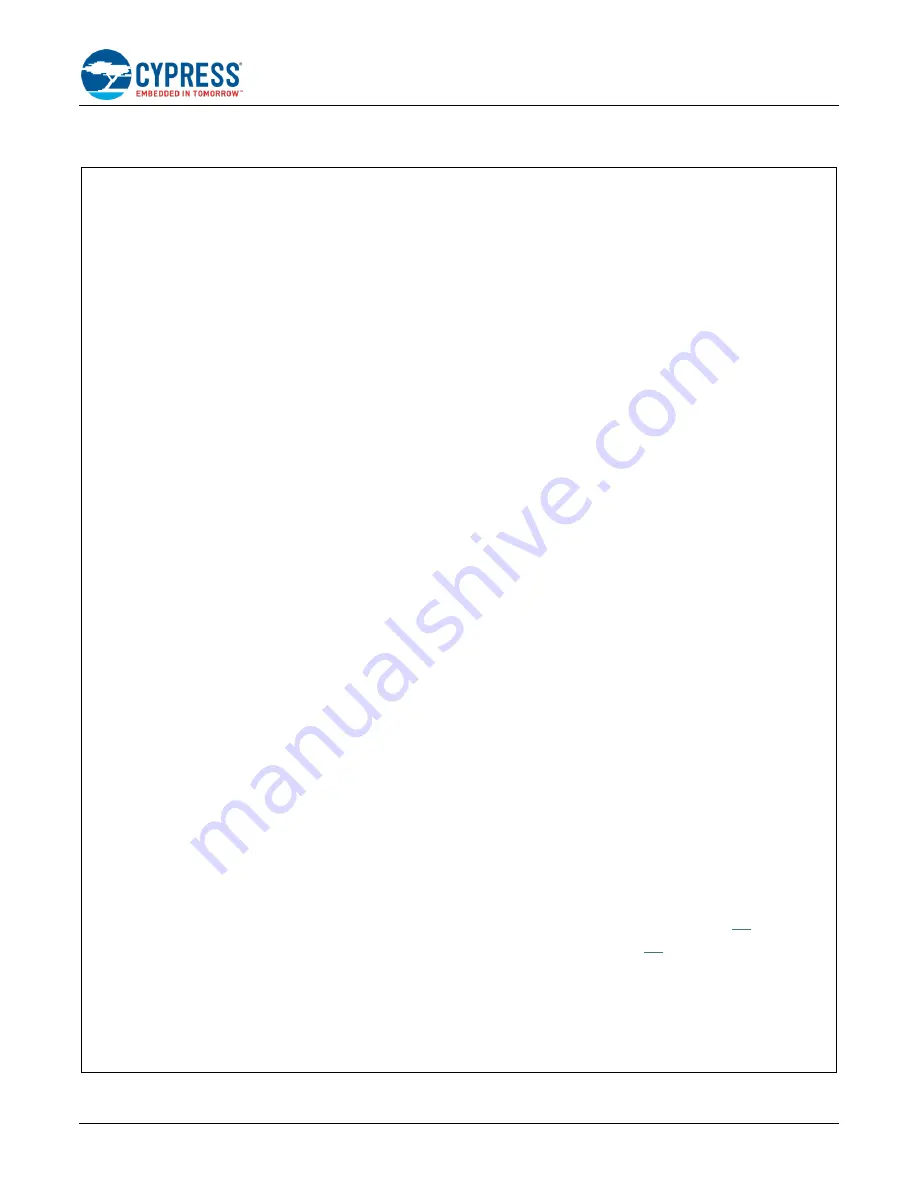
Getting Started with EZ-BT WICED Modules
Document Number: 002-23400 Rev. **
110
Appendix E.
Example Project spp.c
#include
"sparcommon.h"
#include
"wiced.h"
#include
"wiced_gki.h"
#include
"wiced_bt_dev.h"
#include
"wiced_bt_sdp.h"
#include
"wiced_bt_ble.h"
#include
"wiced_bt_uuid.h"
#include
"wiced_hal_nvram.h"
#include
"wiced_bt_app_hal_common.h"
#include
"wiced_bt_trace.h"
#include
"wiced_bt_cfg.h"
#include
"wiced_bt_spp.h"
#include
"hello_sensor.h"
#include
"wiced_timer.h"
#include
"wiced_transport.h"
#include
"wiced_hal_platform.h"
#include
"wiced_memory.h"
#define
HCI_TRACE_OVER_TRANSPORT 1
// If defined HCI traces are send over transport/WICED HCI interface
#define
SEND_DATA_ON_INTERRUPT 1
// If defined application button causes 1Meg of data to be sent
#define
SEND_DATA_ON_TIMEOUT 1
// If defined application sends 4 bytes of data every second
//#define LOOPBACK_DATA 1 // If defined application loops back received data
#define
WICED_EIR_BUF_MAX_SIZE 264
#define
SPP_NVRAM_ID 0x50
/* Max TX packet to be sent over SPP */
#define
MAX_TX_BUFFER 1017
#define
TRANS_MAX_BUFFERS 10
#define
TRANS_UART_BUFFER_SIZE 1024
#define
SPP_MAX_PAYLOAD 1007
#if
SEND_DATA_ON_INTERRUPT
#include
"wiced_hal_gpio.h"
#include
"wiced_hal_platform.h"
#define
APP_TOTAL_DATA_TO_SEND 1000000
#define
BUTTON_GPIO WICED_P30
int
app_send_offset = 0;
uint8_t
app_send_buffer[SPP_MAX_PAYLOAD];
#endif
#ifdef
SEND_DATA_ON_TIMEOUT
void
app_timeout
(
uint32_t
count);
#endif
/*****************************************************************************
** Structures
*****************************************************************************/
#define
SPP_RFCOMM_SCN 2
static
void
spp_connection_up_callback
(
uint16_t
handle,
uint8_t
* bda);
static
void
spp_connection_down_callback
(
uint16_t
handle);
static
wiced_bool_t
spp_rx_data_callback
(
uint16_t
handle,
uint8_t
* p_data,
uint32_t
data_len);
wiced_bt_spp_reg_t
spp_reg =
{
SPP_RFCOMM_SCN,
/* RFCOMM service channel number for SPP connection */
MAX_TX_BUFFER,
/* RFCOMM MTU for SPP connection */
spp_connection_up_callback,
/* SPP connection established */
NULL,
/* SPP connection establishment failed, not used because this app never
initiates connection */
NULL,
/* SPP service not found, not used because this app never initiates
connection */
spp_connection_down_callback,
/* SPP connection disconnected */
spp_rx_data_callback,
/* Data packet received */
};
wiced_transport_buffer_pool_t
* host_trans_pool;
uint16_t
spp_handle;
wiced_timer_t
app_tx_timer;
const
uint8_t
app_sdp_db[] =
// Define SDP database