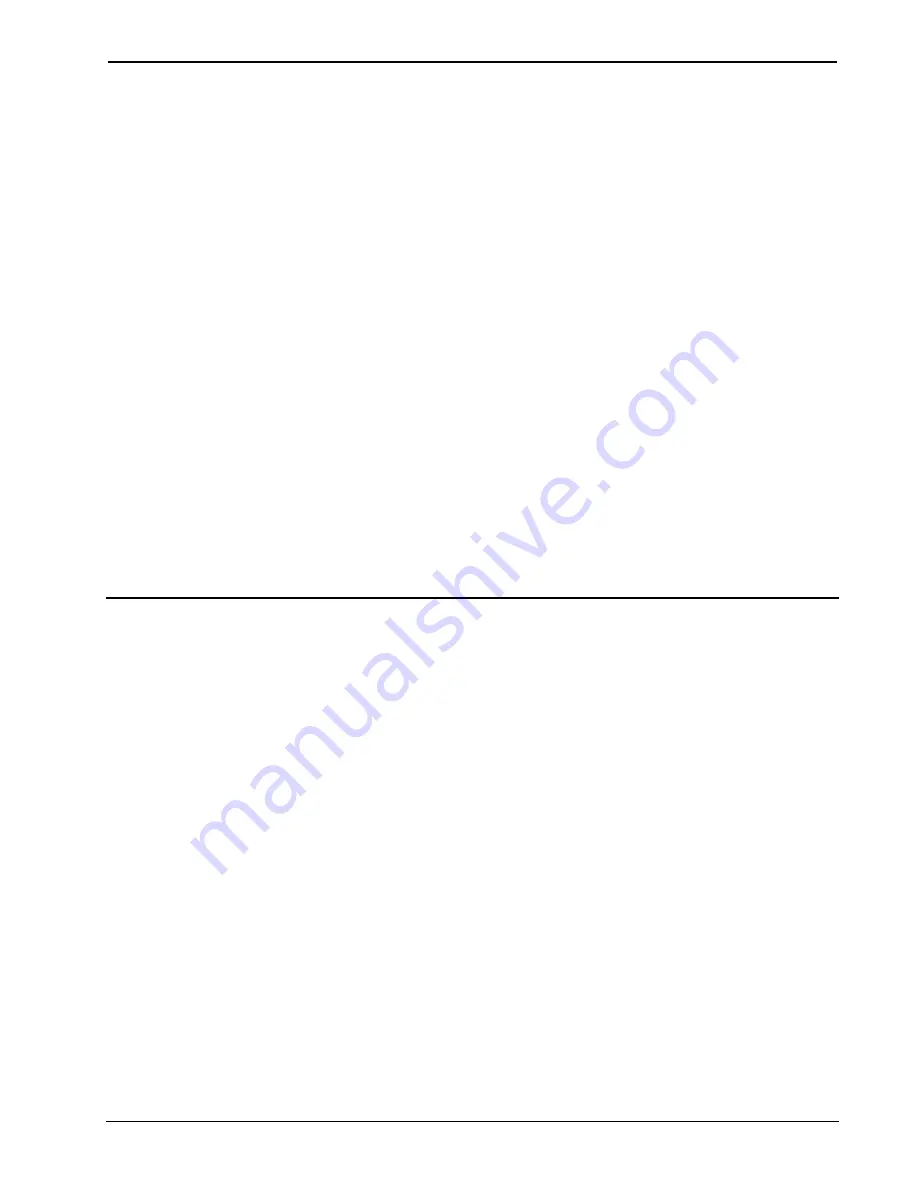
Crestron
SIMPL+
Software
Examine an example using the
switch-case
construct. Perhaps there is a variable that
should hold the number of days in the current month. The following example uses
switch-case
to set the value of this variable.
switch (getMonthNum())
{
case (2): //February
{
if (leapYear) // this variable was set elsewhere
numdays
=
29;
else
numdays
=
28;
}
case (4): // April
numdays
=
30;
case (6): // June
numdays
=
30;
case (9): // September
numdays
=
30;
case (11): // November
numdays
=
30;
default: // Any other month
numdays
=
31;
}
Notice that curly braces did not enclose many of the statements in the previous
example. For most SIMPL+ constructs, the braces are only needed when more than
one statement is to be grouped together. If the program has only a single statement
following the case keyword, then the braces are optional.
Controlling Program Flow: Loops
“Controlling Program Flow: Branching” (on page 24) discussed constructs for
controlling the flow of a program by making decisions and branching. Sometimes a
program should execute the same code a number of times. This is called looping.
SIMPL+ provides three looping constructs: the
for
loop, the
while
loop, and the
do-
until
loop.
for Loops
The
for
loop is useful to cause a section of code to execute a specific number of
times. For example, consider clearing each element of a 15-element string array (set
it to an empty string). Use a
for
loop set to run 15 times and clear one element each
time through the loop.
Control the number of loops a
for
loop executes through the use of an index variable,
which must be an integer variable previously declared in the variable declaration
section of the program. Specify the starting and ending values for the index variable,
and an optional step value (how much the variable increments by each time through
the loop). Inside the loop, the executing code can reference this index.
The syntax of the
for
loop is as follows.
for (<variable> = <start> to <end> step <stepValue>)
{
// code in here executes each time through the loop
}
Programming Guide – DOC. 5789A
SIMPL+
•
27