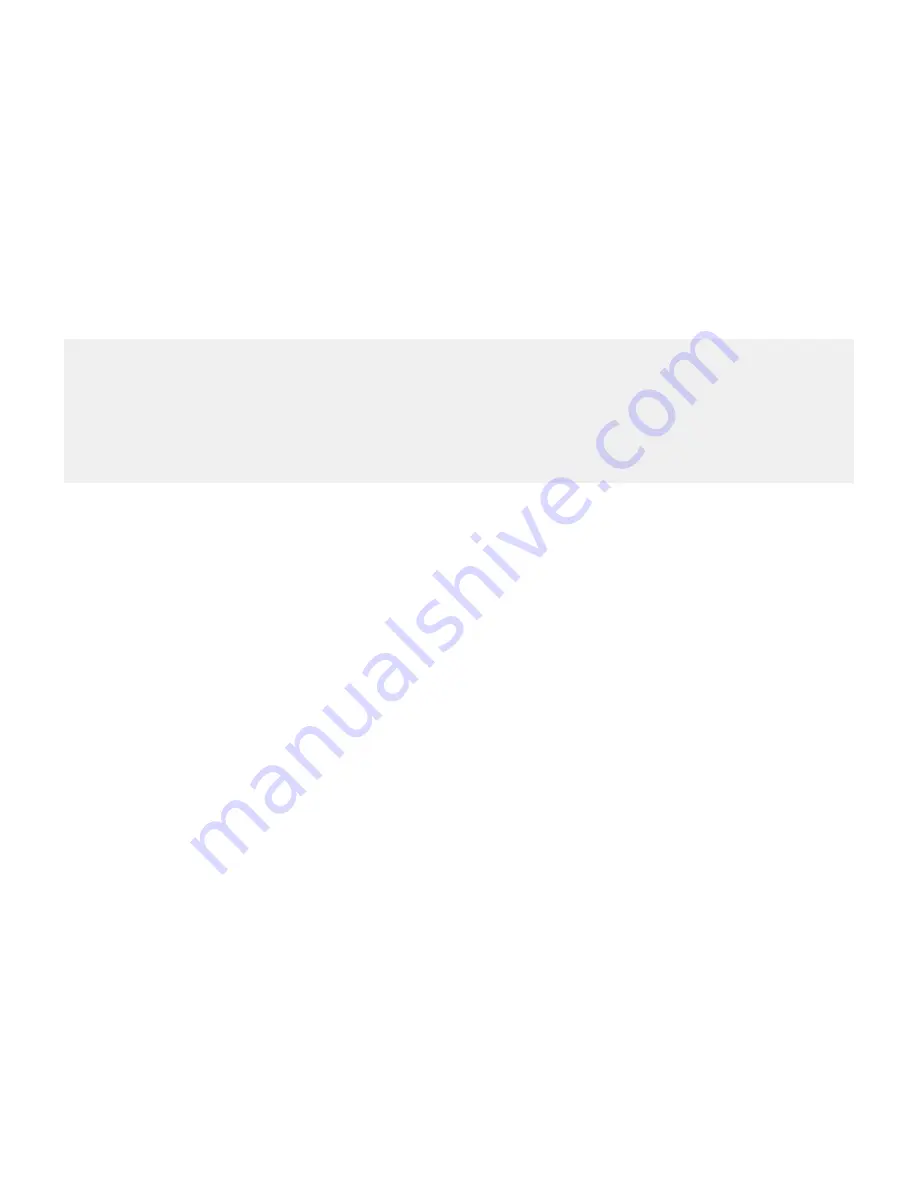
Avoiding StringBuffer.append (StringBuffer)
To append a
String
buffer to another, a BlackBerry® Java Application should use
net.rim.device.api.util.StringUtilities.append
( StringBuffer dst, StringBuffer src[, int offset, int length ] )
.
Code sample
public synchronized StringBuffer append(Object obj) {
if (obj instanceof StringBuffer) {
StringBuffer sb = (StringBuffer)obj;
net.rim.device.api.util.StringUtilities.append( this, sb, 0, sb )
return this;
}
return append(String.valueOf(obj));
}
Avoiding returning null
If you write a public method that returns an object, the method should return null only under the following conditions:
•
Your application expects a null value to occur during normal application operation.
•
The Javadoc™
@return
parameter for the method states that null is a possible return value.
If your application does not expect a null return value, the method should throw an appropriate exception, which forces the caller
of the method to deal explicitly with the problem. The caller of the method might not need to check for a null return value unless
the caller of the method throws a null exception.
Avoiding passing null into methods
Do not pass null parameters into an API method unless the API Reference states explicitly that the method supports them.
Using caution when passing null into a constructor
To avoid ambiguity when passing null into a constructor, cast null to the appropriate object.
If a class has two or more constructors, passing in a null parameter might not uniquely identify which constructor to use. As a
result, the compiler reports an error.
By casting null to the appropriate object, you indicate precisely which constructor the compiler should use. This practice also
provides forward compatibility if later releases of the API add new constructors.
Code sample
Fundamentals Guide
Best practices for writing an efficient BlackBerry Java Application
17