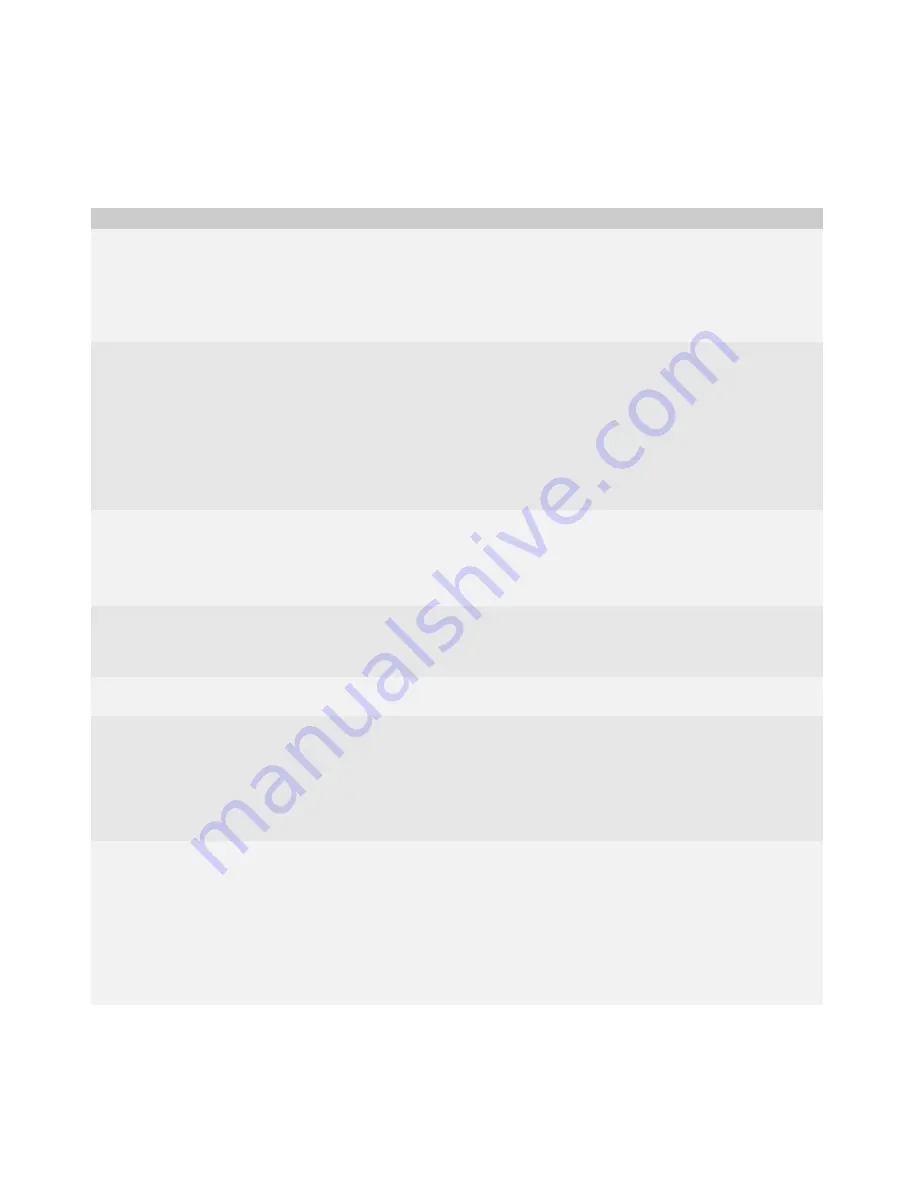
21
3: Work with a message
Send a message
Task
Steps
Create a message.
1.
Create a
Message
object.
2. Specify a folder in which to save a copy of the sent message.
Store store = Session.getDefaultInstance().getStore();
Folder[] folders = store.list(Folder.SENT);
Folder sentfolder = folders[0];
Message msg = new Message(sentfolder);
Specify the recipients.
1.
Create an array of
Address
objects.
2. Add each address to the array.
3. Create code to catch an
AddressException
, which is thrown if an address is invalid.
Address toList[] = new Address[1];
try {
toList[0]= new Address("[email protected]", "Clyde Warren");
} catch(AddressException e) {
System.out.println(e.toString());
}
Add the recipients.
1.
Invoke
Message.addRecipients()
and provide the type of recipient (TO, CC, or BCC) and
the array of addresses to add as parameters to the method.
2. If the message has multiple types of recipients, invoke
addRecipients()
once for each
recipient type.
msg.addRecipients(Message.RecipientType.TO, toList);
Specify the name and email address of
the sender.
>
Invoke
setFrom(Address)
.
Address from = new Address("[email protected]", "Clyde
Warren");
msg.setFrom(from);
Add a subject line.
>
Invoke
setSubject(String)
.
msg.setSubject("Test Message");
Specify the message contents.
>
Invoke
setContent(String)
. Typically, the BlackBerry® Java® Application retrieves content
from text that a BlackBerry device user types in a field.
try {
msg.setContent("This is a test message.");
} catch(MessagingException e) {
System.out.println(e.getMessage());
}
Send the message.
1.
Invoke
Session.getTransport()
and store the returned object in a variable of type
Transport
. The
Transport
object represents the messaging transport protocol.
Transport trans = Session.getTransport();
2. Invoke
trans.send(Message).
try {
trans.send(msg);
} catch(MessagingException e) {
System.out.println(e.getMessage());
}
Summary of Contents for JAVA DEVELOPMENT ENVIRONMENT - - DEVICE APPLICATIONS INTEGRATION - DEVELOPMENT GUIDE
Page 4: ......
Page 7: ......
Page 10: ...10 BlackBerry Device Applications Integration Guide...
Page 12: ...12 BlackBerry Device Applications Integration Guide...
Page 50: ...50 BlackBerry Device Applications Integration Guide...
Page 56: ...56 BlackBerry Device Applications Integration Guide...
Page 65: ......
Page 66: ...2008 Research In Motion Limited Published in Canada...