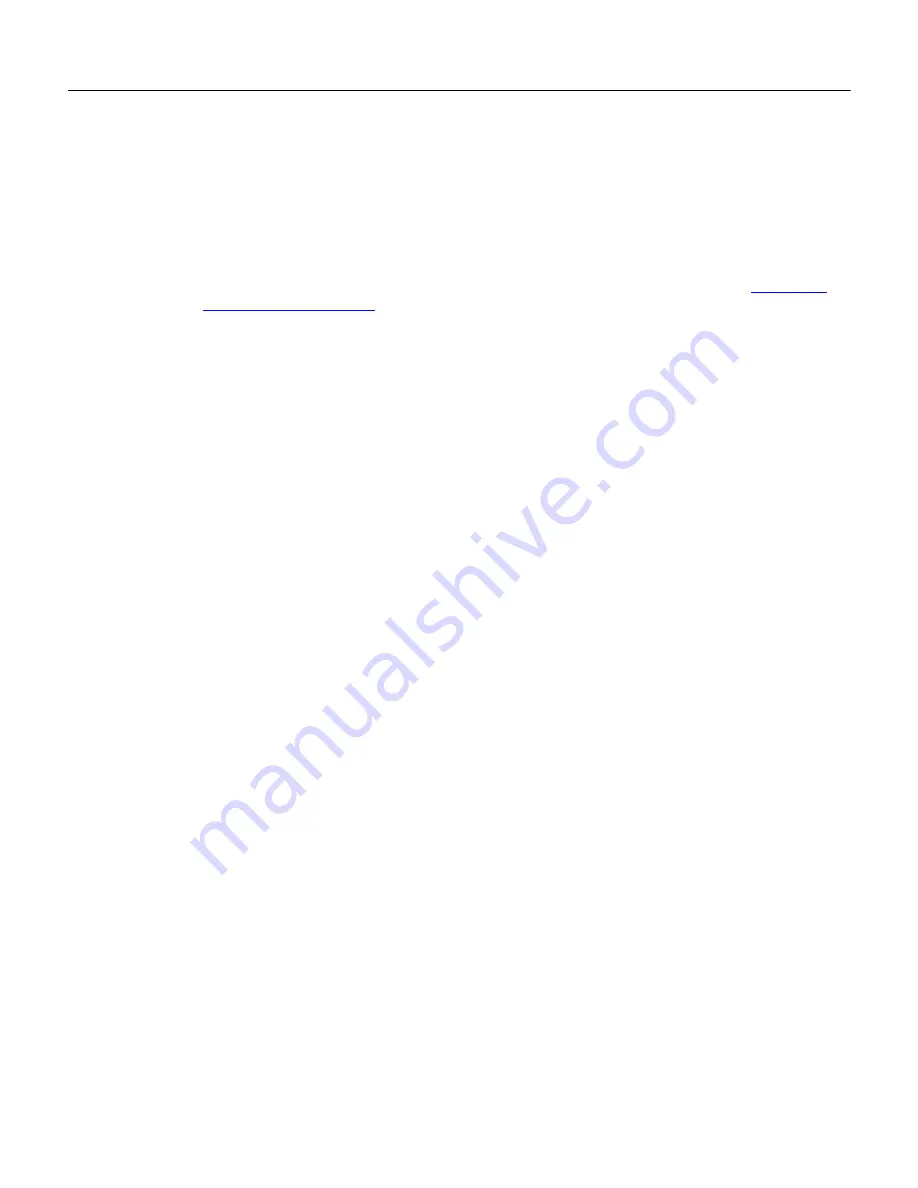
Adobe Acrobat SDK
Working with Bookmarks
Developing Plug-ins and Applications
Retrieving all bookmarks 119
//Retrieve a specific bookmark
myBookmark = PDBookmarkGetByTitle (rootBookmark, bookmarkTitle,
strlen(bookmarkTitle), -1);
if (PDBookmarkIsValid (myBookmark))
AVAlertNote("The bookmark was retrieved");
else
AVAlertNote("The bookmark was not retrieved");
Note:
In the previous code example, a
PDDoc
object named
myPDDoc
is passed to the
PDDocGetBookmarkRoot
method. For information about creating this object, see
“Creating a
PDDoc object” on page 83
.
Retrieving all bookmarks
You can use the Acrobat core API to retrieve all bookmarks located within a PDF document. For example,
you can retrieve the title of every bookmark that is located within a PDF document.
The following code example creates a recursive user-defined function named
VisitAllBookmarks
. First
it invokes the
PDBookmarkIsValid
method to ensure that the bookmark that is passed is valid (the root
bookmark is always valid.)
Second, this user-defined function retrieves the title of the bookmark by invoking the
PDBookmarkGetTitle
method. This method requires the following arguments:
●
A
PDBookmark
object that contains the title to retrieve.
●
A character pointer that is populated with the bookmarks title.
●
An
ASInt32
object that represents the size of the character pointer.
Because the size of the bookmark’s title is unknown, the
PDBookmarkGetTitle
is invoked twice. The
first time it is invoked,
NULL
is passed as the buffer address (second argument) and
0
is specified as the
buffer size (third argument). The text length is returned to an
ASInt32
object named
bufSize
. The
ASmalloc
method is invoked which allocates
bufSize
bytes to the character pointer.
The second time
PDBookmarkGetTitle
is invoked, the allocated character pointer is passed as well as
the
ASInt32
object named
bufSize
. The character pointer is populated with the bookmark’s title. The
AVAlertNote
method is invoked and the character pointer is passed as an argument that results in the
bookmark’s title being displayed within a message box.
The
PDBookmarkHasChildren
method is invoked to determine whether there are any child bookmarks
under the current bookmark. If there are child bookmarks, the
PDBookmarkGetFirstChild
method is
invoked to retrieve the first child bookmark. A recursive call is made to
VisitAllBookmarks
(that is, the
user-defined method is invoking itself ) until there are no more children bookmarks. Then the
PDBookmarkGetNext
method is invoked to get a sibling bookmark and the process continues until there
are no more bookmarks within the PDF document.
Example 9.6
Retrieving existing bookmarks
//Recursively go through bookmark tree to visit each bookmark
void VisitAllBookmarks (PDBookmark aBookmark)
{
PDBookmark treeBookmark;
DURING
//Ensure that the bookmark is valid