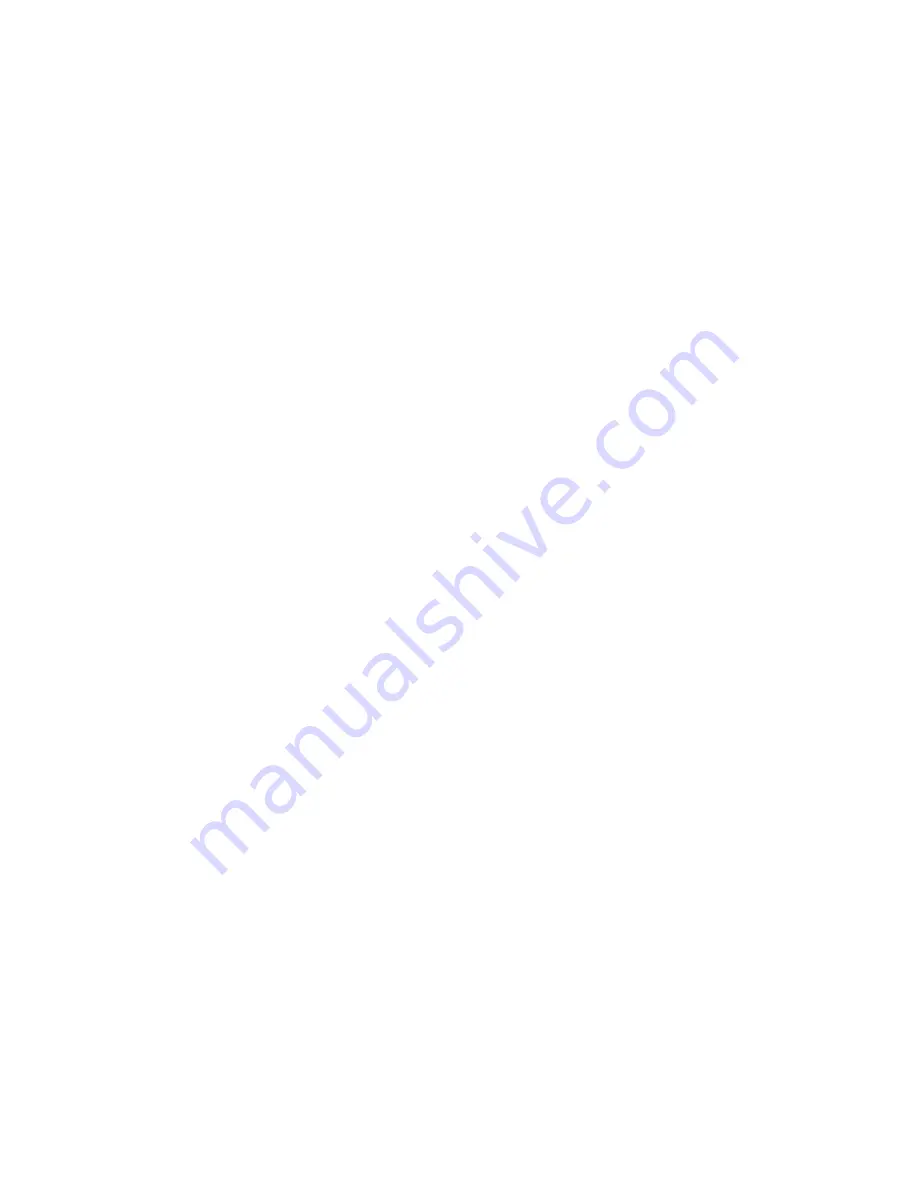
C
HAPTER
2: Tutorial
Callers and selectors
23
typedef struct {
AIFilterHandle filterVariation1;
AIToolHandle toolVariation1;
AIMenuItemHandle aboutPluginMenu;
} Globals;
Globals* g = nil;
static AIErr StartupPlugin ( SPInterfaceMessage* message )
{
AIErr error = kNoErr;
error = AcquireSuites( message->d.basic );
if (!error) {
// Allocate our globals - Illustrator will keep track of these.
error = message->d.basic->AllocateBlock( sizeof(Globals), (void **) &g );
if ( !error ) {
message->d.globals = g;
}
}
if (!error) {
error = AddFilter(message);
}
if (!error) {
error = AddTool(message);
}
if (!error) {
error = AddAction(message);
}
if (!error) {
error = AddMenu(message);
}
ReleaseSuites( message->d.basic );
return error;
}
When allocating memory during this phase, you should use memory management functions provided by
the Illustrator API (see
SPBasicSuite
or
SPBlocksSuite
for example) and put a reference to the memory
in the
globals
field of the
SPMessageData
structure. Illustrator keeps this value for you and passes it back to
you on subsequent calls, so it is a convenient place to store information you may need next time the
plug-in is loaded. Illustrator does not care what you put in the
globals
field. Usually, it is a pointer to a
block of memory. If you do not have any global data, you can leave the
globals
field empty.
During the start-up message, you need to inform Illustrator of the filters, tools and so on that your plug-in
adds. For example, the
StartupPlugin
function above adds a filter. The filter is added by calling the
AIFilterSuite::AddFilter
function provided by the Illustrator API. See the
AddFilter
function in
Tutorial.cpp
for the code that makes this call:
error = sAIFilter->AddFilter( message->d.self, "Tutorial", &filterData,
kToolWantsToTrackCursorOption, &g->filterVariation1);
When adding most plug-in types, the same or similar arguments are used. The first argument is a reference
to the plug-in itself. You can get this from the
SPMessageData
structure. The second argument is an
identifier for the plug-in type. This C-style string identifies the current added plug-in to a second plug-in,
one perhaps searching for the plug-in’s functionality. To be helpful, it should be as descriptive as possible.
Following the identifier are data and options specific to the plug-in type. The data is any information
specific to the plug-in type on a platform. For instance, the filter above has a filter
category
and filter
title
.
All filters with the same category are placed together in one submenu.