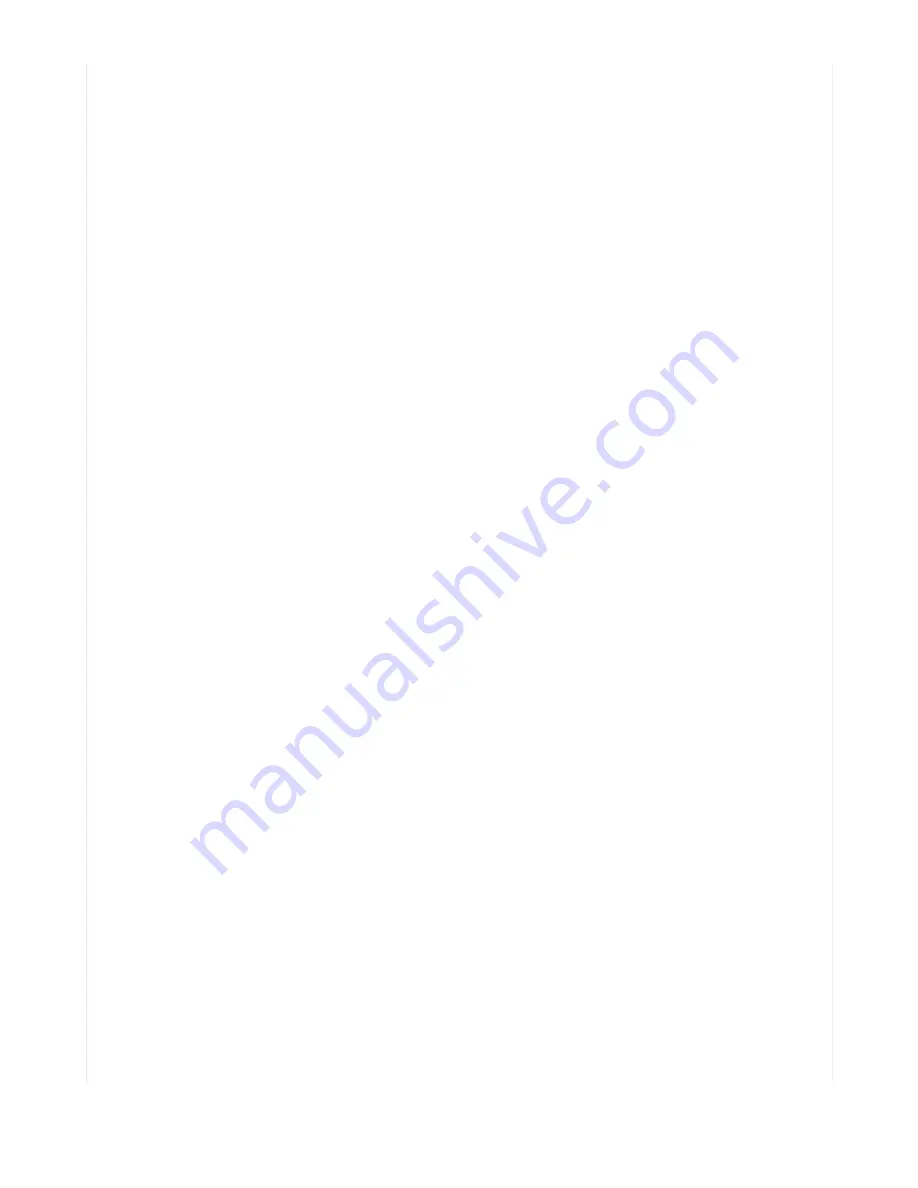
}
}
void generateSawtooth(int32_t amplitude, int32_t* buffer, uint16_t length) {
// Generate a sawtooth signal that goes from -amplitude/2 to amplitude/2
// and store it in the provided buffer of size length.
float delta = float(amplitude)/float(length);
for (int i=0; i<length; ++i) {
buffer[i] = -(amplitude/2)+delta*i;
}
}
void generateTriangle(int32_t amplitude, int32_t* buffer, uint16_t length) {
// Generate a triangle wave signal with the provided amplitude and store it in
// the provided buffer of size length.
float delta = float(amplitude)/float(length);
for (int i=0; i<length/2; ++i) {
buffer[i] = -(amplitude/2)+delta*i;
}
for (int i=length/2; i<length; ++i) {
buffer[i] = (amplitude/2)-delta*(i-length/2);
}
}
void generateSquare(int32_t amplitude, int32_t* buffer, uint16_t length) {
// Generate a square wave signal with the provided amplitude and store it in
// the provided buffer of size length.
for (int i=0; i<length/2; ++i) {
buffer[i] = -(amplitude/2);
}
for (int i=length/2; i<length; ++i) {
buffer[i] = (amplitude/2);
}
}
void playWave(int32_t* buffer, uint16_t length, float frequency, float seconds) {
// Play back the provided waveform buffer for the specified
// amount of seconds.
// First calculate how many samples need to play back to run
// for the desired amount of seconds.
uint32_t iterations = seconds*SAMPLERATE_HZ;
// Then calculate the 'speed' at which we move through the wave
// buffer based on the frequency of the tone being played.
float delta = (frequency*length)/float(SAMPLERATE_HZ);
// Now loop through all the samples and play them, calculating the
// position within the wave buffer for each moment in time.
for (uint32_t i=0; i<iterations; ++i) {
uint16_t pos = uint32_t(i*delta) % length;
int32_t sample = buffer[pos];
// Duplicate the sample so it's sent to both the left and right channel.
// It appears the order is right channel, left channel if you want to write
// stereo sound.
i2s.write(sample, sample);
}
}
void setup() {
// Configure serial port.
Serial.begin(115200);
Serial.println("Zero I2S Audio Tone Generator");
// Initialize the I2S transmitter.
© Adafruit Industries
https://learn.adafruit.com/adafruit-i2s-stereo-decoder-uda1334a
Page 35 of 45