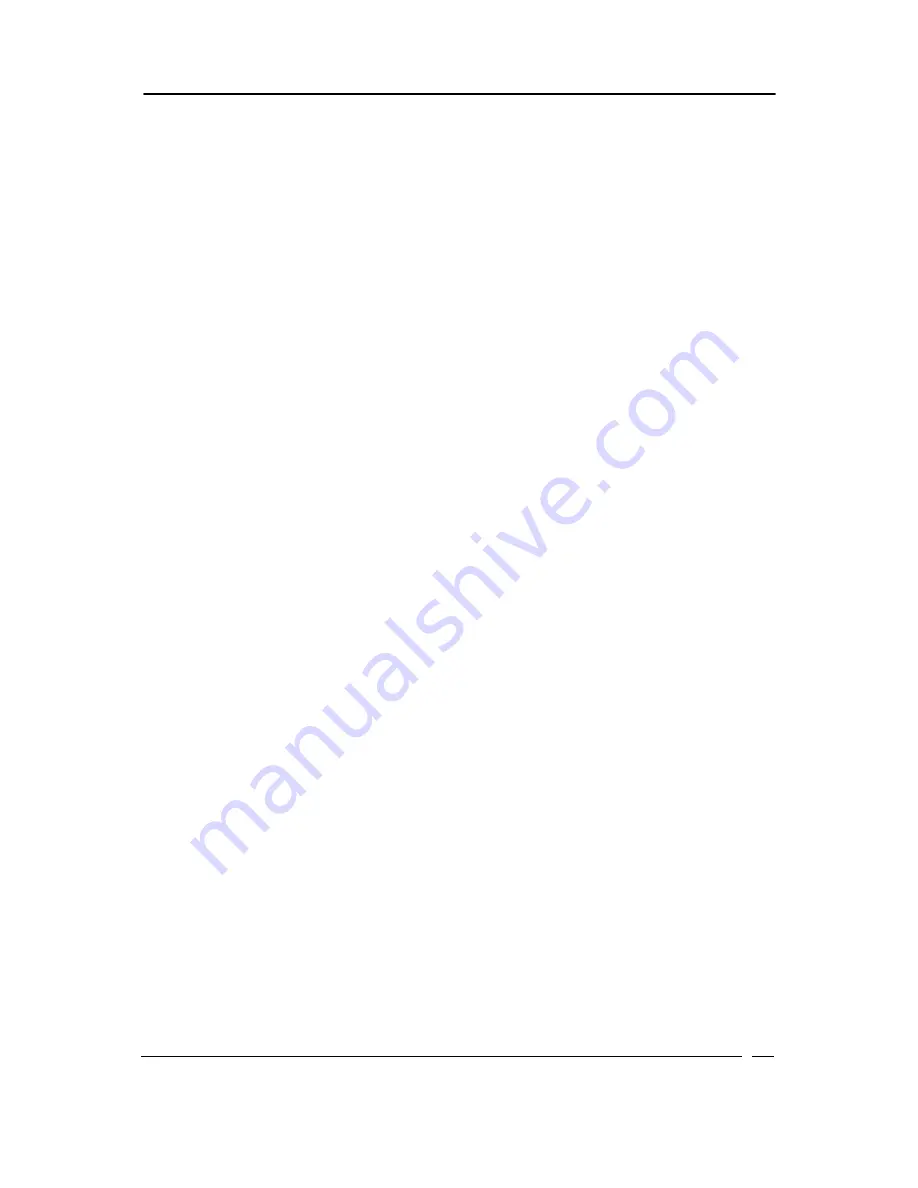
AR-B1893 User’s Guide
AR-B11893 Pentium M inside (With VGA)
with On Board DDR, 4 Port built in LAN, 1 Mini PCI, 1 CF
35
5.1 Watch Dog Test program source codes :
/* Watchdog test program for AR-M9942 */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <conio.h>
#include <dos.h>
typedef unsigned char byte;
const byte config_port=0x2E;
const byte config_data=0x2F;
/* Global values */
static int time_unit=0; // 0=second
// 1=minute
static int time_out=3;
/* function reference */
void get_wdog_time(char *timestr);
void prepare_superio();
void close_superio();
void print_help_message();
void main(int argc, char *argv[])
{
unsigned long waiting_sec;
int x, y;
if(argc > 1) {
if(strcmp(argv[1],"-h") == 0) {
print_help_message();
exit(0);
}
get_wdog_time(argv[1]);
}
prepare_superio(); //turn-on watchdog
if(time_unit == 0)
waiting_sec = time_out;
else
waiting_sec = time_out * 60;
x=wherex();
y=wherey();
while(waiting_sec > 0) {
gotoxy(x,y);
printf(" "); //clear the line :)
gotoxy(x,y);
printf(" %ld\tseconds",waiting_sec);
waiting_sec = waiting_sec - 1;
delay(1000);
}
printf("Error!! Watchdog not function!\n");
//won't be displayed
close_superio();
}
/* get time_unit and time_out from user
input */
void get_wdog_time(char *timestr)
{
int tmp;
tmp=strlen(timestr);
if( (timestr[tmp] == 'm') || (timestr[tmp] ==
'M') ) {
time_unit=1;
}
timestr[tmp]='\0';
tmp=atoi(timestr);
if(tmp != 0) {
if(tmp >255) {
tmp=255;
}
time_out=tmp;
}
}
/* prepare superio for watchdog function */
void prepare_superio()
{
byte tmp;
outportb(config_port,0x87); //enter
configuration mode
outportb(config_port,0x87);
outportb(config_port,0x2B); //CR2B -
GPIO multiplexed pin