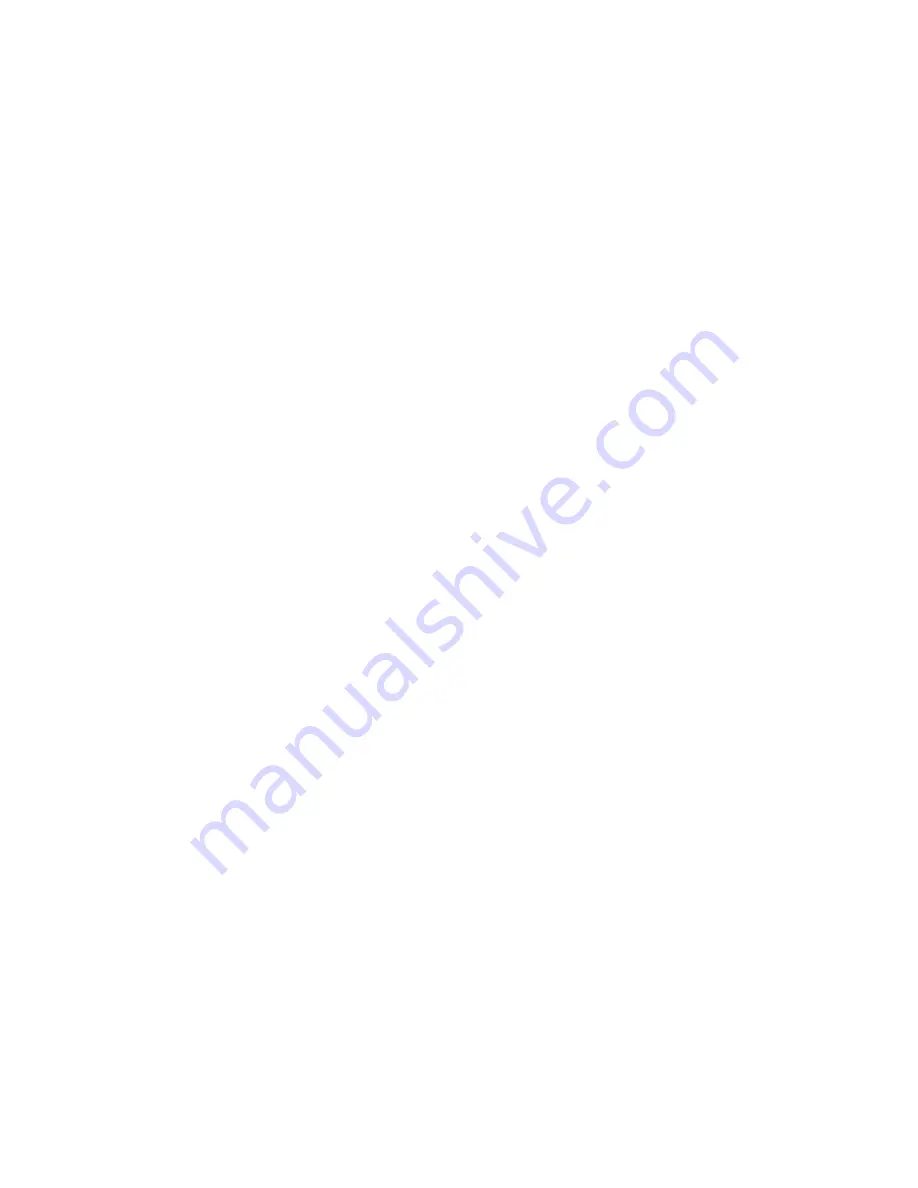
The final initialization step is to flush the receiver buffers. You do this with two reads from the
receiver buffer at Base A0. When done, the UART is ready to use.
Reception
Reception can be handled in two ways: polling and interrupt-driven. When polling, reception is
accomplished by constantly reading the Line Status Register at Base A5. Bit 0 of this
register is set high whenever data are ready to be read from the chip. A simple polling loop must
continuously check this bit and read in data as it becomes available. The following code fragment
implements a polling loop and uses a value of 13, (ASCII Carriage Return) as an end-of-
transmission marker:
do{
while (!(inportb(BA5) & 1)); /*Wait until data ready*/
data[i++]= inportb(BASEADDR);
}while (data[i]!=13);
/*Reads the line until null character
rec'd*/
Interrupt-driven communications should be used whenever possible and is required for high data
rates. Writing an interrupt-driven receiver is not much more complex than writing a polled receiver
but care should be taken when installing or removing your interrupt handler to avoid writing the
wrong interrupt, disabling the wrong interrupt, or turning interrupts off for too long a period.
The handler would first read the Interrupt Identification Register at Base A2. If the
interrupt is for Received Data Available, the handler then reads the data. If no interrupt is
pending, control exits the routine. A sample handler, written in C, is as follows:
readback = inportb(BA2);
if(readback & 4)
/*Readback will be set to 4 if data are available*/
data[i++] = inportb(BASEADDR);
outportb(0x20,0x20);
/*Write
EOI to 8259 Interrupt Controller*/
return;
Transmission
The Auto feature of the card automatically enables the transmitter when data are ready to send
so no software enabling is required. The following software example is for non-Auto operation.
First the RTS line should be set high by writing a 1 to Bit 1 of the Modem Control Register at
Base A4. The RTS line is used to toggle the transceiver from receive mode to transmit
mode and vice versa. It is not carried out on the line in RS485 and is not used for handshaking.
Similarly, the CTS line is not used in RS485 and should always be enabled by installing a jumper
as described earlier.
After the above is done, the card is ready to send data. To transmit a string of data, the
transmitter must first check Bit 5 of the Line Status Register at Base A5. That bit is the
transmitter-holding-register-empty flag. If it is high, the transmitter has sent the data. The process
of checking the bit until it goes high followed by a write is repeated until no data remains. After all
data has been transmitted, the RTS bit should be reset by writing a 0 to Bit 1 of the Modem
Control Register.
Manual PCI-ICM-2S
15