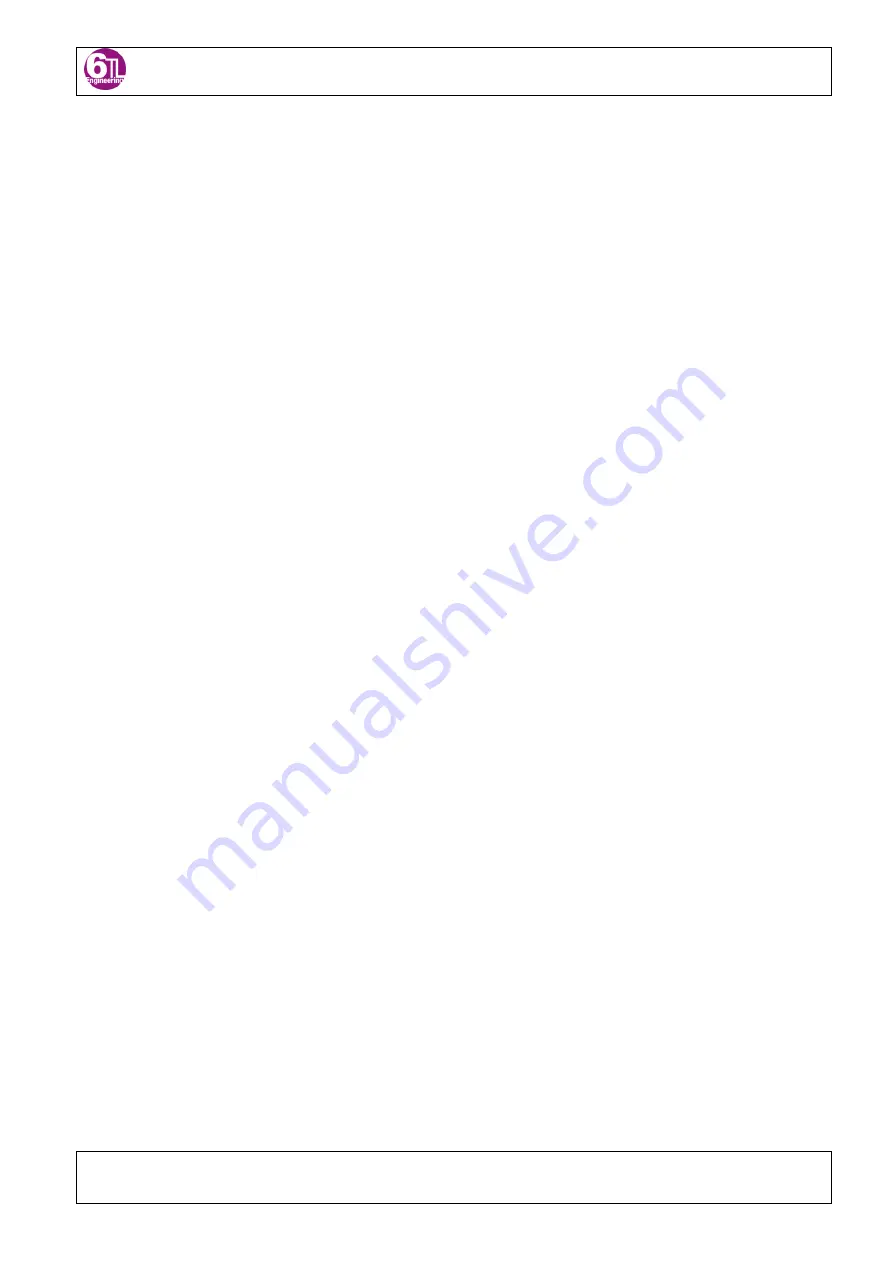
TECHNICAL MANUAL
620020E05
Due to the continuous products improvement, the indications of the present manual can be modified without previous warning and in any
case are a contract commitment. The present information publishing does not represent resignation of intellectual property or patent.
Copyright
2009-2010 S.A. Sistel -E 08211 BARCELONA
Pag. 12
/* Print a description of an NI-CAN error/warning. */
void PrintStat(NCTYPE_STATUS status, char *source)
{
char statusString[1024];
if (status != 0)
{
ncStatusToString(status, sizeof(statusString), statusString);
printf("\n%s\nSource = %s\n", statusString, source);
// close object handle, then exit.
ncCloseObject(TxHandle);
exit(1);
}
}
int main ()
{
NCTYPE_STATUS Status;
NCTYPE_CAN_FRAME Transmit;
NCTYPE_ATTRID AttrIdList[8];
NCTYPE_UINT32 AttrValueList[8];
NCTYPE_UINT32 Baudrate = 50000;
char Interface[7] = "CAN0";
int ch;
/* Configure the CAN Network Interface Object */
AttrIdList[0] = NC_ATTR_BAUD_RATE;
AttrValueList[0] = Baudrate;
AttrIdList[1] = NC_ATTR_START_ON_OPEN;
AttrValueList[1] = NC_TRUE;
AttrIdList[2] = NC_ATTR_READ_Q_LEN;
AttrValueList[2] = 0;
AttrIdList[3] = NC_ATTR_WRITE_Q_LEN;
AttrValueList[3] = 1;
AttrIdList[4] = NC_ATTR_CAN_COMP_STD;
AttrValueList[4] = 0;
AttrIdList[5] = NC_ATTR_CAN_MASK_STD;
AttrValueList[5] = NC_CAN_MASK_STD_DONTCARE;
AttrIdList[6] = NC_ATTR_CAN_COMP_XTD;
AttrValueList[6] = 0;
AttrIdList[7] = NC_ATTR_CAN_MASK_XTD;
AttrValueList[7] = NC_CAN_MASK_XTD_DONTCARE;
Status = ncConfig(Interface, 8, AttrIdList, AttrValueList);
if (Status < 0)
{
PrintStat(Status, "ncConfig");
}
/* open the CAN Network Interface Object */
Status = ncOpenObject(Interface, &TxHandle);
if (Status < 0)
{
PrintStat(Status, "ncOpenObject");
}
/* print the Help to the I/O window */
printf("\n\ninitialized successfuly on CAN0 ...\n\nPress 't' to transmit a frame \n\nPress 'q' to
quit \n\n");
/* Pulse YAV904X8 relay 1 second, each time the user is pressing a key */
do
{
ch = _getch();
if (ch == 't')
{
Transmit.Data[0] = 0x02; // YAV command prefix
Transmit.Data[1] = 0x01; // YAV command prefix
Transmit.Data[2] = 0x00; // relay number
Transmit.Data[3] = 0x01; // YAV set relay ON command
Transmit.DataLength = 4; // Set CAN frame lenght