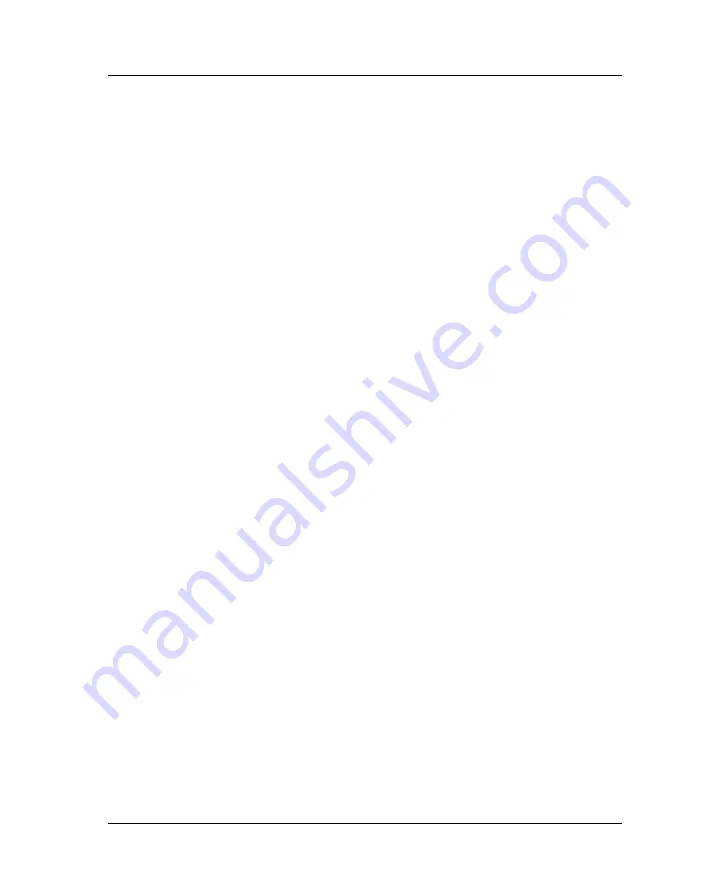
50
Argus Encoder Family Version 2.6 API Developer’s Guide
FMTestApp
Following is an example of the implementation of an event handler. Note that we
call
MessageBox()
for demonstration purposes only.
For deliverable applications,
you should not hold up a log event — or any other type of event — with a
function requiring user input.
/*******************************************************************************
* OnLog()
*
* Event sink called by Filter Manager fires a log event.
******************************************************************************/
STDMETHODIMP CFilterManagerEvents::OnLog(long code, BSTR error)
{
CString strMessage = _T(“”);
strMessage.Format ( _T(“%s”), ( LPCTSTR ) error);
pView->MessageBox( strMessage );
if( !pFile )
return S_OK;
// Send error message to log file.
if( code < 0 )
_ftprintf( pFile, _T(“Warning %ld: %s\n”), code, strMessage );
else if (code !=99)
_ftprintf( pFile, _T(“%s\n”), strMessage);
fflush( pFile );
return S_OK;
}
When you implement the event interfaces, it is important to consider what Argus
software threads or processes will be firing them. Most events generated by the
Argus API will be called in the context of their own thread, which is engaged in
near real-time processing with the encoder hardware. If event interface methods
cause excessive delays, exceptions to be raised, or the calling thread to wait indef-
initely on a synchronization object, undesirable behavior is sure to result. If these
types of operations need to be performed in response to an event, create your own
thread, return control to the calling thread, and proceed to do the processing in the
context of your own thread.
For example, if receiving a Finished event should trigger your application to start
the next encode, make certain that your implementation of Finished event just
toggles a flag or a semaphore, which prompts another thread (perhaps the main