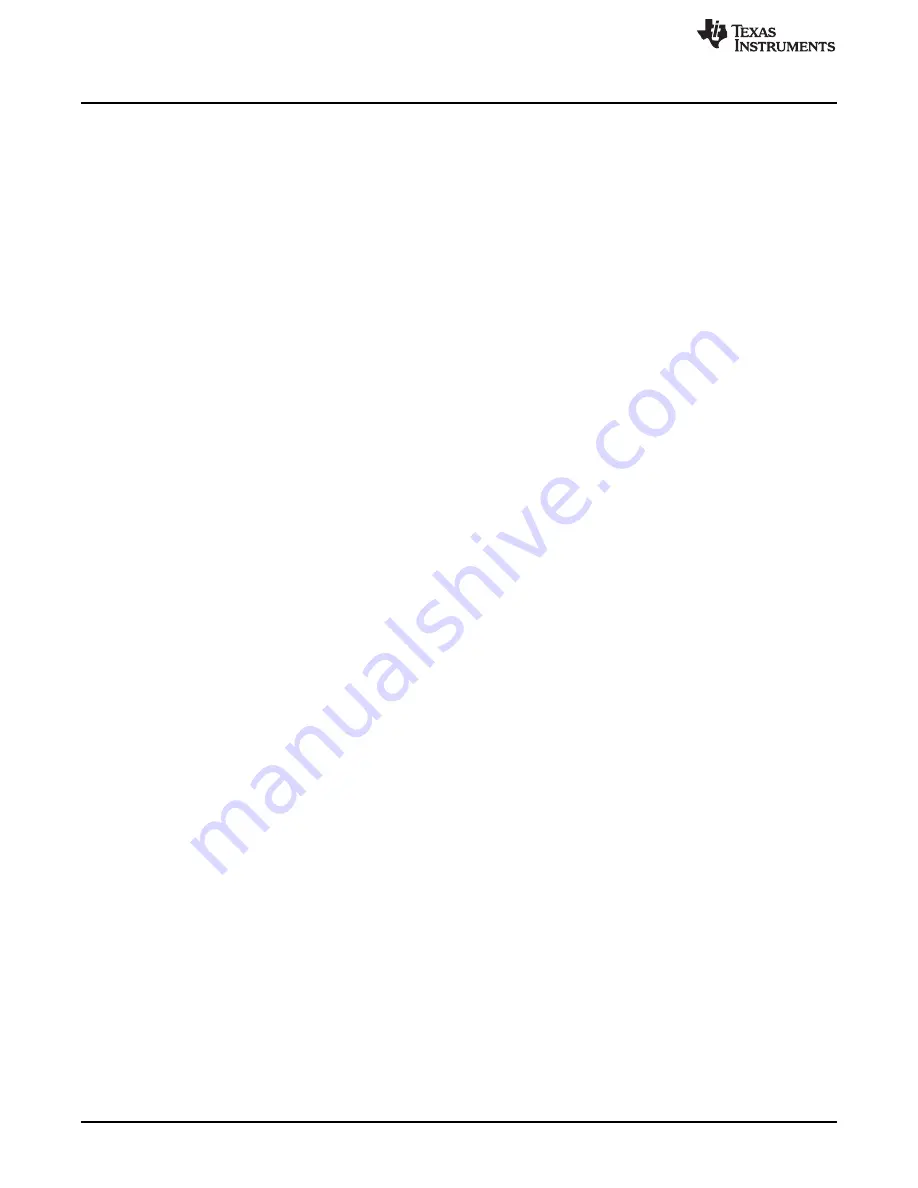
Operation Modes
92
SWRU455A – February 2017 – Revised March 2017
Copyright © 2017, Texas Instruments Incorporated
Socket
{
// error
}
An example of a non-blocking TCP connect:
_i16 Status;
SlSockAddrIn_t
Addr;
Addr.sin_family
= SL_AF_INET;
Addr.sin_port
= sl_Htons(5001);
Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(192,168,1,31));
Status = SL_ERROR_BSD_EALREADY;
while
( 0 > Status )
{
Status = sl_Connect(Sd, ( SlSockAddr_t *)&Addr,
sizeof
(SlSockAddr_t));
if
( 0 > Status )
{
if
( SL_ERROR_BSD_EALREADY != Status )
{
// error
break
;
}
}
}
An example of receiving data with no wait flag:
_i16 Status;
_i8
RecvBuf[1460];
Status = sl_Recv(Sd, RecvBuf, 1460, SL_MSG_DONTWAIT);
if
( (0 > Status) && (SL_ERROR_BSD_EAGAIN != Status) )
{
// error
}
An example of setting the receive data timeout:
_i16 Status;
struct
SlTimeval_t TimeVal;
TimeVal.tv_sec =
5;
// Seconds
TimeVal.tv_usec = 0;
// Microseconds. 10000 microseconds resolution
Status = sl_SetSockOpt(Sd,SL_SOL_SOCKET,SL_SO_RCVTIMEO, (_u8 *)&TimeVal, sizeof(TimeVal));
//
Enable receive timeout
if
( Status )
{
// error
}
6.7.2 Trigger Mode
The trigger mode enables host applications to be triggered by the SimpleLink device when network activity
is detected, without using the blocking mode or polling the socket. This mode is useful when the power
consumption is extremely sensitive and the host processor is able to enter a deep sleep, recover fast, and
retain memory. The trigger mode is implemented by calling sl_Select. The host enters a deep sleep and
wakes up due to an event, when one or more sockets become ready. After the host wakes up, sl_Select
must be called again to identify the network activity. All blocking socket operations can be monitored by
sl_Select, called with time-out values set to 0 (sec and µs), which allow application flexibility to implement
many communication use cases. Only one select operation is supported simultaneously.
To define the host application in trigger mode follow these steps:
•
Define host IRQ as the host wake up source.
•
Ensure slcb_SocketTriggerEventHandler is registered under user.h and handle the trigger