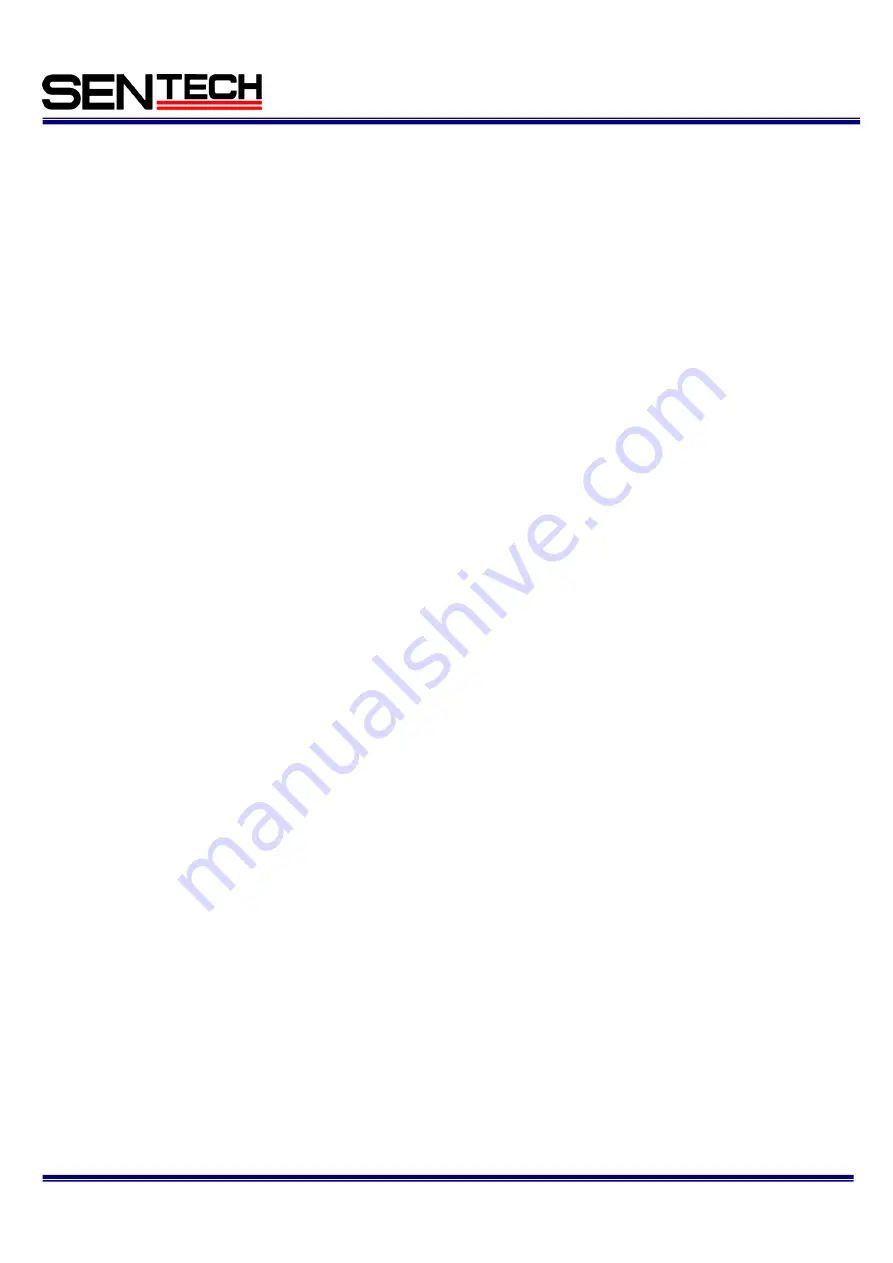
31/33
Sentech GigE Vision Camera StGigE SDK
Sample Guide Rev.1.00
2.5 Uses the serial communication
This sample shows how to use the serial communication between the camera and the PC.
Communicates to the main FPGA of the camera when selects "PvIPEngineSerial0" for the port number.
Sentech GigE cameras do NOT use "PvIPEngineSerial1".
The following sample program shows how to sends 6Byte data then receives 4Byte data.
//SerialPort Open
BOOL SerialPortOpen( PvDevice *pDevice, PvSerialPortIPEngine *pPort, INT iPortNo )
{
PvResult lResult;
if( iPortNo==0 ) lResult = pPort->Open( pDevice, PvIPEngineSerial0 );
else lResult = pPort->Open( pDevice, PvIPEngineSerial1 );
return lResult.IsOK();
}
//SerialPort Close
BOOL SerialPortClose( PvSerialPortIPEngine *pPort )
{
BOOL bReval=TRUE;
if( pPort->IsOpened() ){
PvResult lResult = pPort->Close();
bReval = lResult.IsOK();
}
return bReval;
}
//Data Send and Recieve
BOOL SerialDataSendRev( PvSerialPortIPEngine *pPort, PBYTE pbyteSend, WORD wSendsize, PBYTE pbyteRev,
WORD wRevsize, PWORD pReadsize )
{
PvUInt32 aBytesWritten;
PvResult lResult = pPort->Write( pbyteSend, wSendsize, aBytesWritten );
if( lResult.IsOK() ){
PvUInt32 readBytes;
DWORD dwTimes = 100;
do{
lResult = pPort->GetRxBytesReady(readBytes);
if( lResult.IsOK() && readBytes>=wRevsize )
break;
Sleep(10);
}while(--dwTimes);
PvUInt32 aBytesRead;
PvUInt32 aTimeout=1000;
lResult = pPort->Read( pbyteRev, wRevsize, aBytesRead, aTimeout );
if( lResult.IsOK() ) *pReadsize = aBytesRead;
}
return lResult.IsOK();
}