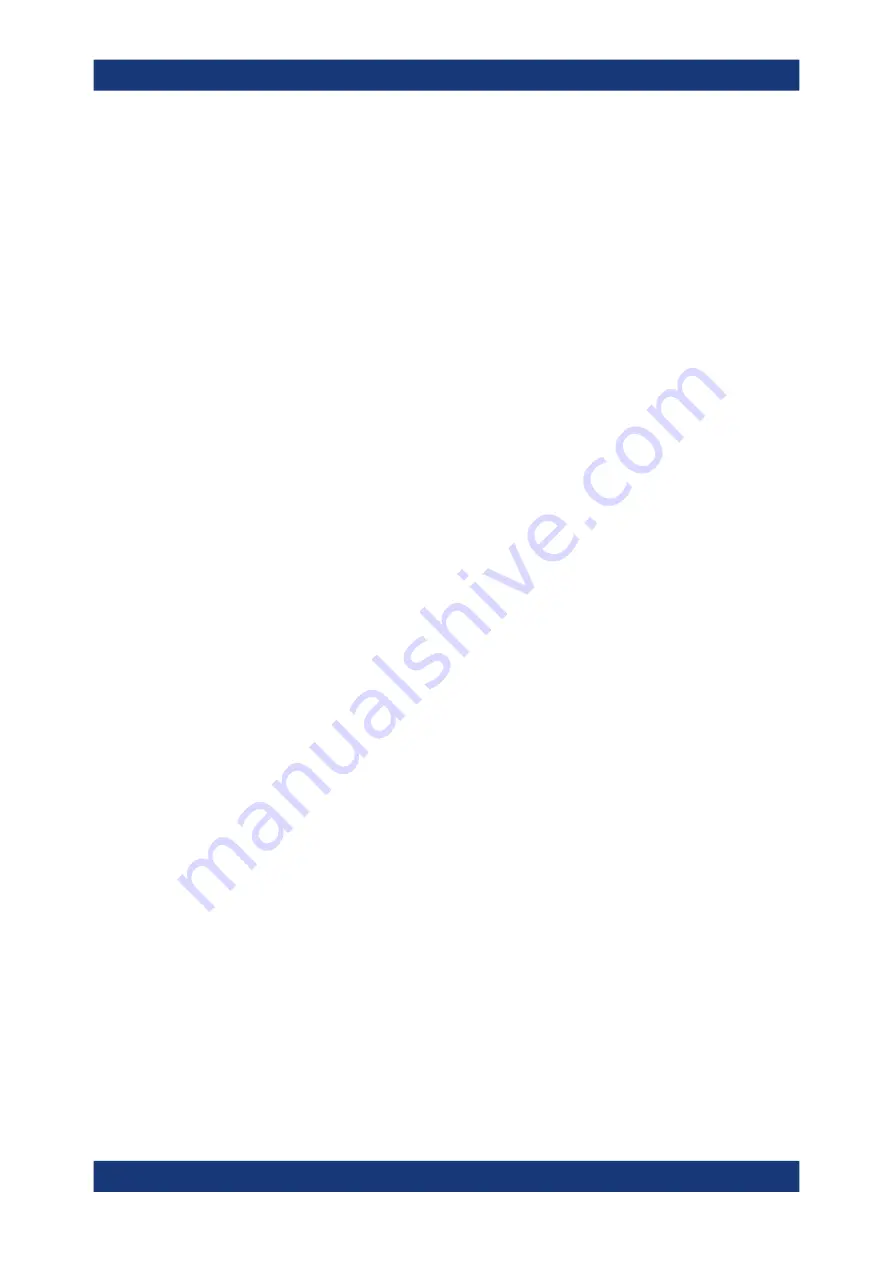
I/Q over Ethernet (QSFP+) protocol
R&S
®
SMM-K507
46
User Manual 1179.5718.02 ─ 02
Example: To transfer waveform data
# General, network and port specfications
import socket
import ctypes, sys
from ctypes import *
UDP_IP_CLIENT = "192.168.180.9"
UDP_IP_ADDRESS = "192.168.180.14"
UDP_PORT_NO = 49152
UDP_PORT_CLIENT = 32551
frm_size = 63624
strCmdWvConfig = "STOP_ARB_AND_SET_ARB_PARAMS:"
strCmdWvRestart = "CHECK_STATE_AND_RESTART_ARB"
class rxAck_t(Structure): # size = 20
_fields_ = [("frm_cnt", c_uint16), # 0x0200
("reserve", c_uint8), # 0
("frm_err", c_uint8), # 0: no errors, other: error bit mask
("info", c_uint32), # number of received samples
("reserved", c_uint8 * 12)]
class txFrm_t(Structure):
_fields_ = [("frm_cnt", c_uint16), # flow cntrl counter
("reserved", c_uint8),
("frm_type", c_uint8), # frame type (command/data)
("pld_sz", c_uint16), # frame payload without header in
# bytes
("prt_ver", c_uint16), # protocol version
("payload", c_uint8 * frm_size)]
class startWvTransmFrm_t(Structure):
_fields_ = [("frm_cnt", c_uint16), # flow cntrl counter
("reserve", c_uint8),
("frm_type", c_uint8), # frame type (command/data)
("pld_sz", c_uint16), # frame payload without header in
# bytes
("prt_ver", c_uint16), # protocol version
("segm_id", c_uint32), # 0
("segm_addr",c_uint32), # 0
("samples", c_uint64)] # number of 32bit (2x16bit), I/Q
# samples (number is multible of 128)
def readAck(clientSock):
clientSock.settimeout(3.0)
resp = clientSock.recvfrom(18) # read 18 bytes
clientSock.settimeout(None)
ack = bytearray(resp[0]) + bytearray(2)
return rxAck_t.from_buffer(ack)
Using a python script for waveform data transfer