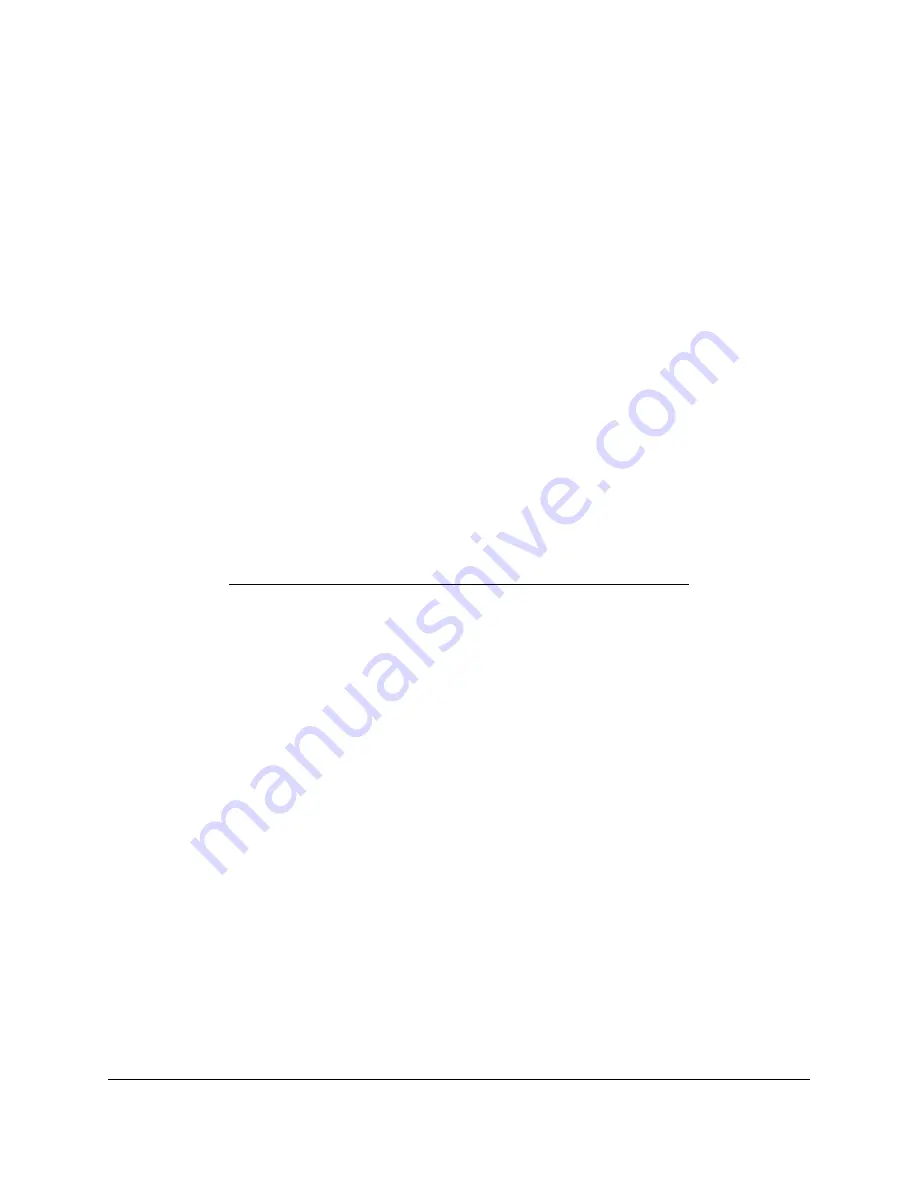
You can also write a computer program to use the serial port. The freely available Microsoft
.NET framework contains a
SerialPort
class that makes it easy to read and write bytes from
a serial port. Here is some example C# .NET code that uses a serial port:
// Choose the port name and the baud rate.
System.IO.Ports.SerialPort port = new System.IO.Ports.SerialPort("COM4", 115200);
// Connect to the port.
port.Open();
// Transmit two bytes on the TX line: 1, 2
port.Write(new byte[]{1, 2}, 0, 2);
// Wait for a byte to be received on the RX line.
int response = port.ReadByte();
// Show the user what byte was received.
MessageBox.Show("Received byte: " + response);
// Disconnect from the port so that other programs can use it.
port.Close();
5.a. Communicating via the Serial Control Lines
In addition to transmitting bytes on the
TX
line and receiving bytes on the
RX
line, the USB-
to-TTL-serial adapter can use programmer pins
A
and
B
as serial handshaking lines of your
choosing. Each pin can be configured as an input or an output by identifying it with a serial
handshaking line using the programmer’s configuration utility (see
). The table
below shows which handshaking lines are available (CTS is not available because there is no
provision for it in the USB CDC ACM subclass).
Direction Name .NET System.IO.Ports.SerialPort member
Output
DTR
DtrEnable
Output
RTS
RtsEnable
Input
CD
CDHolding
Input
DSR
DsrHolding
Input
RI
N/A
By default, pins
A
and
B
are high-impedance inputs that are not identified with any
handshaking line. The programmer stores the handshaking line configurations in persistent
memory, so they only need to be configured when you want to change the associations.
Pololu USB AVR Programmer User's Guide
© 2001–2010 Pololu Corporation
5. Communicating via the USB-to-TTL-Serial Adapter
Page 26 of 36