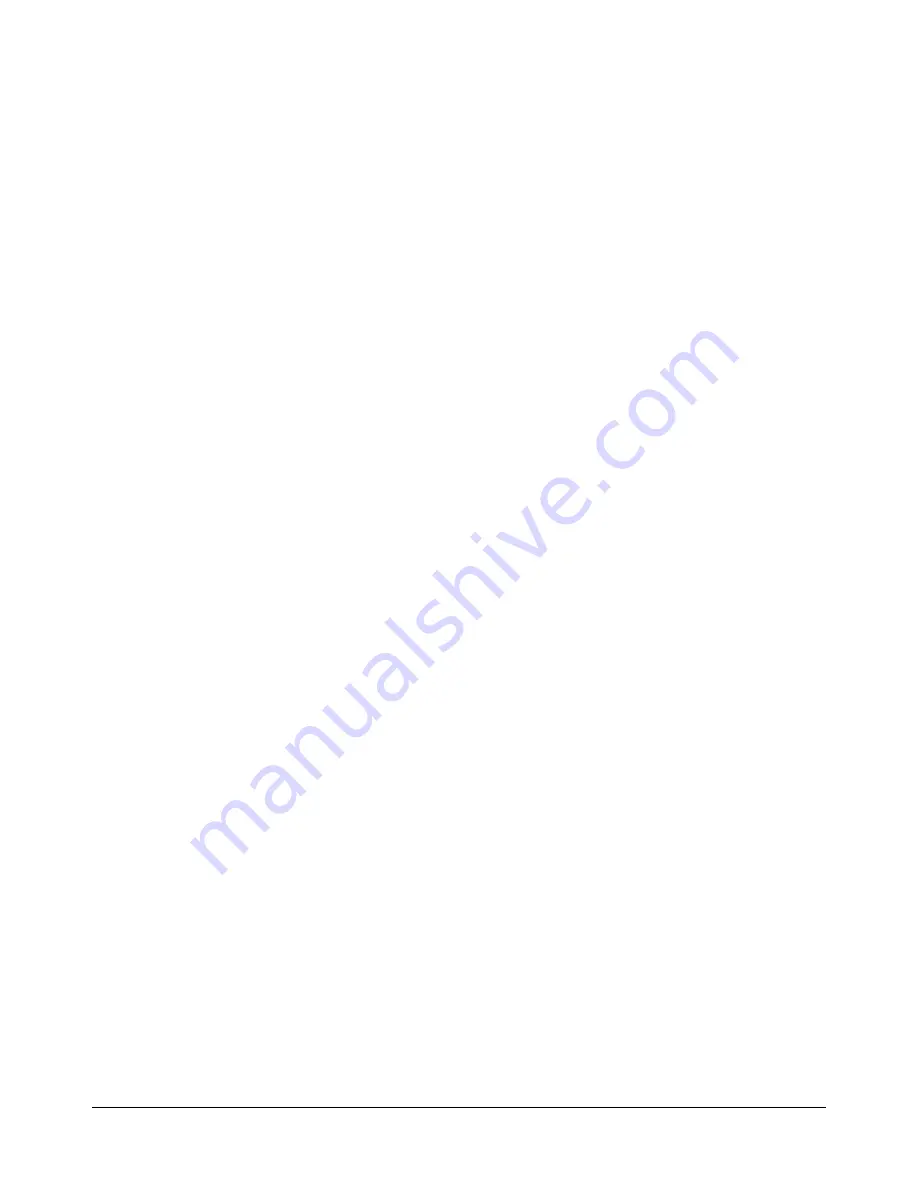
Creating and Running Custom Algorithms
115
No decimal point or exponent specified.
32-bit hexadecimal integer; 0Xhhh... or 0xhhh... where h is a hex digit.
32-bit floating point; ddd., ddd.ddd, ddde
±
dd, dddE
±
dd, ddd.dddedd,
or ddd.dddEdd where d is a decimal digit.
Flow Control:
conditional construct if(){ } else { }
Intrinsic Functions:
Return minimum; min(<expr1>,<expr2>)
Return maximum; max(<expr1>,<expr2>)
User defined function; <user_name>(<expr>)
Write value to CVT element; writecvt(<expr>,<expr>)
Write value to FIFO buffer; writefifo(<expr>)
Write value to both CVT and FIFO; writeboth(<expr>,<expr>)
Example Language
Usage
Here are examples of some Algorithm Language elements assembled to
show them used in context. Later sections will explain any unfamiliar
elements you see here:
Example 1;
/*** get input from channel 8, calculate output, check limits, output to ch 16 & 17 ***/
static float output_max = .020;
/* 20 mA max output */
static float output_min = .004;
/* 4 mA min output */
static float input_val, output_val;
/* intermediate I/O vars */
input val_ = I108;
/* get value from input buffer channel 8*/
output_val = 12.5 * input_val;
/* calculate desired output */
if ( output_val > output_max )
/* check output greater than limit */
output_val = output_max;
/* if so, output max limit */
else if( output_val < output_min)
/* check output less than limit */
output_val = output_min;
/* if so, output min limit */
O116 = output_val / 2;
/* split output_val between two SCP */
O117 = output_val / 2;
/* channels to get up to 20mA max */
Example 2;
/*** same function as example 1 above but shows a different approach ***/
static float max_output = .020;
/* 20 mA max output */
static float min_output = .004;
/* 4 mA min output */
/* following lines input, limit output between min and max_output, and outputs. */
/* output is split to two current output channels wired in parallell to provide 20mA */
O116 = max( min_output, min( max_output, (12.5 * I108) / 2 ) );
O117 = max( min_output, min( max_output, (12.5 * I108) / 2 ) );
The Algorithm Execution Environment
This section describes the execution environment that the HP E1415
provides for your algorithms. Here we describe the relationship of your
algorithm to the main() function that calls it.
The Main Function
All ’C’ language programs consist of one or more functions. A ’C’ program
must have a function called main(). In the HP E1415, the main() function is
usually generated automatically by the driver when you execute the INIT
command. The main() function executes each time the module is triggered,
Содержание VXI 75000 C Series
Страница 2: ......
Страница 16: ...16 ...
Страница 18: ......
Страница 30: ...30 Getting Started Chapter 1 Notes ...
Страница 32: ...32 Field Wiring Chapter 2 Figure 2 1 Channel Numbers at SCP Positions ...
Страница 44: ...44 Field Wiring Chapter 2 Figure 2 11 HP E1415 Terminal Module ...
Страница 54: ...54 Field Wiring Chapter 2 Notes ...
Страница 61: ...Programming the HP E1415 for PID Control 61 Chapter 3 Programming Overview Diagram ...
Страница 124: ...124 Creating and Running Custom Algorithms Chapter 4 Figure 4 2 Algorithm Operating Sequence Diagram ...
Страница 136: ...136 Creating and Running Custom Algorithms Chapter 4 Notes ...
Страница 152: ...152 Algorithm Language Reference Chapter 5 Notes ...
Страница 304: ...304 HP E1415 Command Reference Chapter 6 Command Quick Reference Notes ...
Страница 308: ...308 Specifications Appendix A Thermocouple Type E 200 800C SCPs HP E1501 02 03 ...
Страница 309: ...Specifications 309 Appendix A Thermocouple Type E 200 800C SCPs HP E1508 09 ...
Страница 310: ...310 Specifications Appendix A Thermocouple Type E 0 800C SCPs HP E1501 02 03 ...
Страница 311: ...Specifications 311 Appendix A Thermocouple Type E 0 800C SCPs HP E1509 09 ...
Страница 312: ...312 Specifications Appendix A Thermocouple Type E Extended SCPs HP E1501 02 03 ...
Страница 313: ...Specifications 313 Appendix A Thermocouple Type E Extended SCPs HP E1508 09 ...
Страница 314: ...314 Specifications Appendix A Thermocouple Type J SCPs HP E1501 02 03 ...
Страница 315: ...Specifications 315 Appendix A Thermocouple Type J SCPs HP E1508 09 ...
Страница 316: ...316 Specifications Appendix A Thermocouple Type K SCPs HP E1501 02 03 ...
Страница 317: ...Specifications 317 Appendix A Thermocouple Type R SCPs HP E1501 02 03 ...
Страница 318: ...318 Specifications Appendix A Thermocouple Type R SCPs HP E1508 09 ...
Страница 319: ...Specifications 319 Appendix A Thermocouple Type S SCPs HP E1501 02 03 ...
Страница 320: ...320 Specifications Appendix A Thermocouple Type S SCPs HP E1508 09 ...
Страница 321: ...Specifications 321 Appendix A Thermocouple Type T SCPs HP E1501 02 03 ...
Страница 322: ...322 Specifications Appendix A Thermocouple Type T SCPs HP E1508 09 ...
Страница 323: ...Specifications 323 Appendix A 5K Thermistor Reference SCPs HP E1501 02 03 ...
Страница 324: ...324 Specifications Appendix A 5K Thermistor Reference SCPs HP E1508 09 ...
Страница 325: ...Specifications 325 Appendix A RTD Reference SCPs HP E1501 02 03 ...
Страница 326: ...326 Specifications Appendix A RTD SCPs HP E1501 02 03 ...
Страница 327: ...Specifications 327 Appendix A RTD SCPs HP E1508 09 ...
Страница 328: ...328 Specifications Appendix A 2250 Thermistor SCPs HP E1501 02 03 ...
Страница 329: ...Specifications 329 Appendix A 2250 Thermistor SCPs HP E1508 09 ...
Страница 330: ...330 Specifications Appendix A 5K Thermistor SCPs HP E1501 02 03 ...
Страница 331: ...Specifications 331 Appendix A 5K Thermistor SCPs HP E1508 09 ...
Страница 332: ...332 Specifications Appendix A 10K Thermistor SCPs HP E1501 02 03 ...
Страница 333: ...Specifications 333 Appendix A 10K Thermistor SCPs HP E1508 09 ...
Страница 334: ...334 Specifications Appendix A Notes ...
Страница 346: ...346 Glossary Appendix C Notes ...
Страница 388: ...388 Generating User Defined Functions Appendix F Notes ...
Страница 438: ...438 Index Writing the algorithm 129 values to CVT elements 120 values to the FIFO 121 Z ZERO CALibration ZERO 194 ...